1. Create a java program from the supplied starter code: TestRecursion.java. 2. The array given can be used or you may input a different one and search for a value in it. YOU MUST have a minimum of 11 elements in your array if you do not use mine. 3. Create a method bubleSortRecur() that performs a bubble sort using recursion. search. The method accepts two parameters: an array of integers (any order) and the length. Print the original array, in the main() method after the sort is called. 4. Call another method, BinarySearchRecur() that performs binary search recursively. It should take 4 parameters: a sorted array of integers, start position (index) and end position(index) of the array, and the integer value to find. It should return the index of the element if found, if not then it should return -1. Print the one integer being searched, and its index position from within the main() method
1. Create a java program from the supplied starter code: TestRecursion.java.
2. The array given can be used or you may input a different one and search for a value in it. YOU MUST
have a minimum of 11 elements in your array if you do not use mine.
3. Create a method bubleSortRecur() that performs a bubble sort using recursion. search. The method
accepts two parameters: an array of integers (any order) and the length. Print the original array, in the
main() method after the sort is called.
4. Call another method, BinarySearchRecur() that performs binary search recursively. It should take 4
parameters: a sorted array of integers, start position (index) and end position(index) of the array, and the
integer value to find. It should return the index of the element if found, if not then it should return -1.
Print the one integer being searched, and its index position from within the main() method
PLEASE ADD TO THE CODE GIVEN BELOW AND FOLLOW THE SLASHED LINES FOR ASSISTANCE
import java.util.Arrays;
public class TestRecursion {
public static void main(String[] args) {
int Xarr[] = new int[] {1,22,3,41,5,66,74,8,16,9,99,23,45,174,249,201,341,64,72,104};
System.out.println("original array: "+ Arrays.toString(Xarr));
bubbleSortRecur(Xarr,Xarr.length);
System.out.println("sorted array: "+Arrays.toString(Xarr));
int k= 0;
int zKey=66;
k = binarySearchRecur(Xarr,0,Xarr.length-1,zKey);
System.out.println("Search value: "+zKey+" found in position: "+k);
}
public static void bubbleSortRecur(int Xarr[], int n) {
//Recall from chapter9, when the first pass in the
//bubblesort is done, the highest value in the array
//is at the end. SO, you call itself with the same 'array'
//and one les for the length...
//base case is when the length, 'n' is equal 1.
}
public static int binarySearchRecur(int[] array, int start, int end, int target) {
//if the 'end' is less than the 'start' value
//you have reached the end of the array and
//not found the 'target' value.
//IF the target is the 'array[middle]' value
// then return the 'middle' as the value found to match
// the target (or zKey)
//if the target is less than the array element in middle
// the call yourself with 'start' and middle-1
// else call yourslef with 'middle+1' and 'end'
//remember you can call yourself with a 'return' statement since this
//method always returns an integer.
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

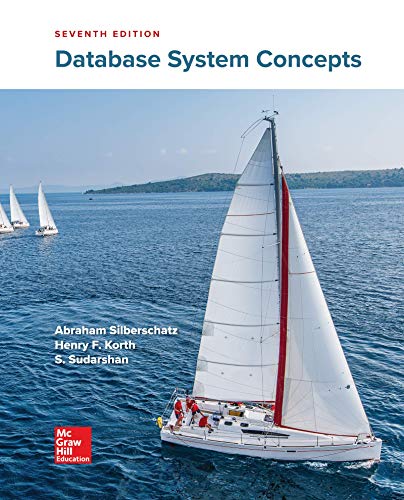
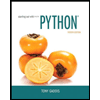
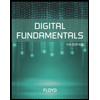
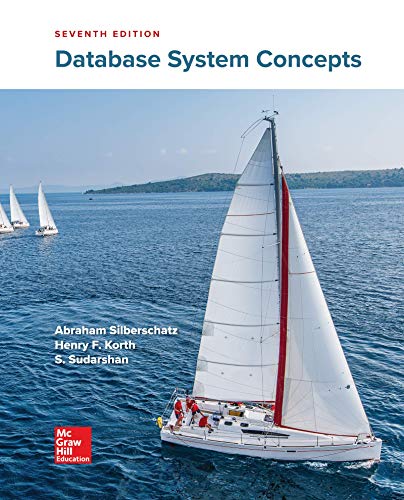
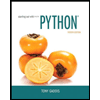
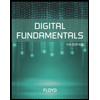
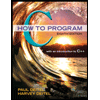
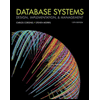
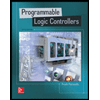