In Java. Just fill in the code where it says "/* Complete the method and update the return statement */"please. Program Specifications Write a program to calculate U.S. income tax owed given wages, taxable interest, unemployment compensation, status (dependent, single, or married), and taxes withheld. Dollar amounts are displayed as integers with comma separators. Ex: System.out.printf("Cost: $%,d\n", cost); Note: this program is designed for incremental development. Complete each step and submit for grading before starting the next step. Only a portion of tests pass after each step but confirm progress. Step 1. Within main() input wages, taxable interest, unemployment compensation, status (0=dependent, 1=single, and 2=married), and taxes withheld as integers. Step 2 . Complete the calcAGI() method. Calculate the adjusted gross income (AGI) that is the sum of wages, interest, and unemployment. Convert any negative values to positive before summing to correct potential input errors. Return the AGI. Note the provided code in main() calls calcAGI() and outputs the returned value. Submit for grading to confirm two tests pass. Ex: If the input is: 20000 23 500 1 400 The output is: AGI: $20,523 Step 3 . Complete the getDeduction() method. Return the deduction amount based on status: (0) dependent = 6000, (1) single = 12000, or (2) married=24000. Return 6000 if the status is anything but 0, 1, or 2. Within main() call getDeduction() and output the returned value. Submit for grading to confirm four tests pass. Ex: If the input is: 20000 23 500 1 400 The additional output is: AGI: $20,523 Deduction: $12,000 Step 4 . Complete the calcTaxable() method. Calculate taxable amount (AGI - deduction). Set taxable to zero if calculation results in negative value. Return taxable value. Within main() call calcTaxable() and output the returned value. Submit for grading to confirm six tests pass. Ex: If the input is: 20000 23 500 1 400 The additional output is: AGI: $20,523 Deduction: $12,000 Taxable income: $8,523 Step 5. Complete the calcTax() method. Calculate tax amount based on status and taxable income (see tables below). Tax amount should be stored initially as a double, rounded to the nearest whole number using Math.round(), and converted to an integer before returning. Within main() call calcTax() and output the returned value. Submit for grading to confirm eight tests pass. Ex: If the input is: 50000 0 0 2 5000 The additional output is: AGI: $50,000 Deduction: $24,000 Taxable income: $26,000 Federal tax: $2,720 Income Tax for Dependent or Single Filers $0 - $10,000 10% of the income $10,001 - $40,000 $1,000 + 12% of the amount over $10,000 $40,001 - $85,000 $4,600 + 22% of the amount over $40,000 over $85,000 $14,500 + 24% of the amount over $85,000 Income Tax for Married Filers $0 - $20,000 10% of the income $20,001 - $80,000 $2,000 + 12% of the amount over $20,000 over $80,000 $9,200 + 22% of the amount over $80,000 Step 6. Complete the calcTaxDue() method. Set withheld parameter to zero if negative to correct potential input error. Calculate and return amount of tax due (tax - withheld). Within main() call calcTaxDue() and output returned value. Submit for grading to confirm all tests pass. Ex: If the input is: 80000 0 500 2 12000 The additional output is: AGI: $80,500 Deduction: $24,000 Taxable income: $56,500 Federal tax: $6,380 Tax due: $-5,620 import java.util.Scanner; public class LabProgram { // Calculate AGI and repair any negative values public static int calcAGI(int wages, int interest, int unemployment) { /* Complete the method and update the return statement */ return -1; } // Calculate deduction depending on single, dependent or married public static int getDeduction(int status) { /* Complete the method and update the return statement */ return -1; } // Calculate taxable but not allow negative results public static int calcTaxable(int agi, int deduction) { /* Complete the method and update the return statement */ return -1; } // Calculate tax for single or dependent public static int calcTax(int status, int taxable) { /* Complete the method and update the return statement */ return -1; } // Calculate tax due and check for negative withheld public static int calcTaxDue(int tax, int withheld) { /* Complete the method and update the return statement */ return -1; } public static void main(String [] args) { Scanner scan = new Scanner(System.in); int wages = 0; int interest = 0; int unemployment = 0; int status = -1; int withheld = 0; int agi; // Step #1: Input information // Step #2: Calculate AGI agi = calcAGI(wages, interest, unemployment); System.out.printf("AGI: $%,d\n", agi); }
In Java. Just fill in the code where it says "/* Complete the method and update the return statement */"please.
Program Specifications Write a program to calculate U.S. income tax owed given wages, taxable interest, unemployment compensation, status (dependent, single, or married), and taxes withheld. Dollar amounts are displayed as integers with comma separators. Ex: System.out.printf("Cost: $%,d\n", cost);
Note: this program is designed for incremental development. Complete each step and submit for grading before starting the next step. Only a portion of tests pass after each step but confirm progress.
Step 1. Within main() input wages, taxable interest, unemployment compensation, status (0=dependent, 1=single, and 2=married), and taxes withheld as integers.
Step 2 . Complete the calcAGI() method. Calculate the adjusted gross income (AGI) that is the sum of wages, interest, and unemployment. Convert any negative values to positive before summing to correct potential input errors. Return the AGI. Note the provided code in main() calls calcAGI() and outputs the returned value. Submit for grading to confirm two tests pass.
Ex: If the input is:
20000 23 500 1 400
The output is:
AGI: $20,523
Step 3 . Complete the getDeduction() method. Return the deduction amount based on status: (0) dependent = 6000, (1) single = 12000, or (2) married=24000. Return 6000 if the status is anything but 0, 1, or 2. Within main() call getDeduction() and output the returned value. Submit for grading to confirm four tests pass.
Ex: If the input is:
20000 23 500 1 400
The additional output is:
AGI: $20,523 Deduction: $12,000
Step 4 . Complete the calcTaxable() method. Calculate taxable amount (AGI - deduction). Set taxable to zero if calculation results in negative value. Return taxable value. Within main() call calcTaxable() and output the returned value. Submit for grading to confirm six tests pass.
Ex: If the input is:
20000 23 500 1 400
The additional output is:
AGI: $20,523 Deduction: $12,000 Taxable income: $8,523
Step 5. Complete the calcTax() method. Calculate tax amount based on status and taxable income (see tables below). Tax amount should be stored initially as a double, rounded to the nearest whole number using Math.round(), and converted to an integer before returning. Within main() call calcTax() and output the returned value. Submit for grading to confirm eight tests pass.
Ex: If the input is:
50000 0 0 2 5000
The additional output is:
AGI: $50,000 Deduction: $24,000 Taxable income: $26,000 Federal tax: $2,720
Income | Tax for Dependent or Single Filers |
---|---|
$0 - $10,000 | 10% of the income |
$10,001 - $40,000 | $1,000 + 12% of the amount over $10,000 |
$40,001 - $85,000 | $4,600 + 22% of the amount over $40,000 |
over $85,000 | $14,500 + 24% of the amount over $85,000 |
Income | Tax for Married Filers |
---|---|
$0 - $20,000 | 10% of the income |
$20,001 - $80,000 | $2,000 + 12% of the amount over $20,000 |
over $80,000 | $9,200 + 22% of the amount over $80,000 |
Step 6. Complete the calcTaxDue() method. Set withheld parameter to zero if negative to correct potential input error. Calculate and return amount of tax due (tax - withheld). Within main() call calcTaxDue() and output returned value. Submit for grading to confirm all tests pass.
Ex: If the input is:
80000 0 500 2 12000
The additional output is:
AGI: $80,500 Deduction: $24,000 Taxable income: $56,500 Federal tax: $6,380 Tax due: $-5,620
import java.util.Scanner;
public class LabProgram {
// Calculate AGI and repair any negative values
public static int calcAGI(int wages, int interest, int unemployment) {
/* Complete the method and update the return statement */
return -1;
}
// Calculate deduction depending on single, dependent or married
public static int getDeduction(int status) {
/* Complete the method and update the return statement */
return -1;
}
// Calculate taxable but not allow negative results
public static int calcTaxable(int agi, int deduction) {
/* Complete the method and update the return statement */
return -1;
}
// Calculate tax for single or dependent
public static int calcTax(int status, int taxable) {
/* Complete the method and update the return statement */
return -1;
}
// Calculate tax due and check for negative withheld
public static int calcTaxDue(int tax, int withheld) {
/* Complete the method and update the return statement */
return -1;
}
public static void main(String [] args) {
Scanner scan = new Scanner(System.in);
int wages = 0;
int interest = 0;
int unemployment = 0;
int status = -1;
int withheld = 0;
int agi;
// Step #1: Input information
// Step #2: Calculate AGI
agi = calcAGI(wages, interest, unemployment);
System.out.printf("AGI: $%,d\n", agi);
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

How do i fix this error in the code you provided?
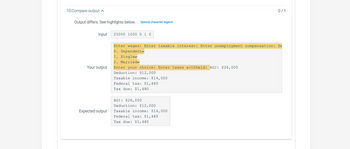
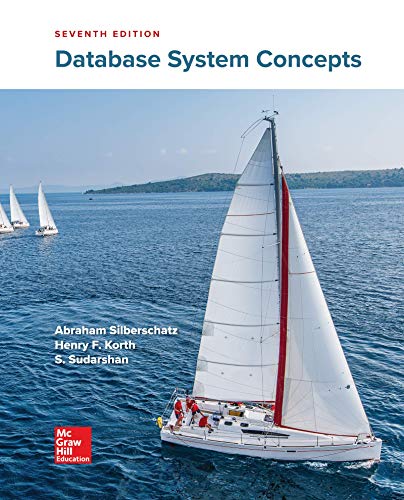
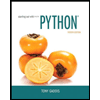
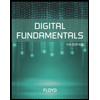
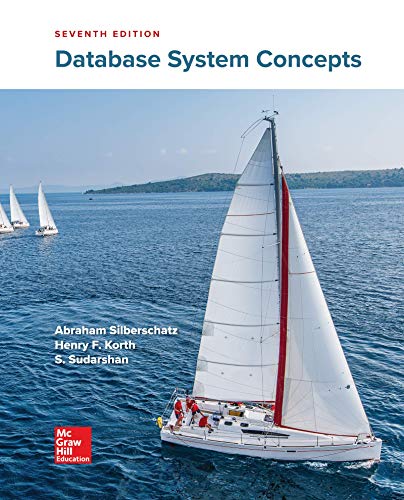
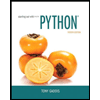
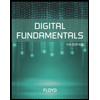
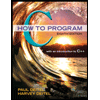
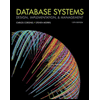
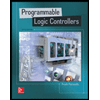