Add 3 parameters to each function in the code below. ********************************************* Code starts here ************************************************** Module.py #Defination to sort the list def sort(listNum): sortedList = [] #While loop will run until the listNum don't get null while(len(listNum) != 0 ): #Set the min as first element in list min = listNum[0] #iterate over the list to compare every element with num for ele in listNum: #If element is less than min if ele < min: #Then set min as element min = ele #append the sorted element in list sortedList.append(min) #Remove the sorted element from the list listNum.remove(min) return sortedList #Function to find the sum of all elements in list def SumOfList(listNum): #Set the sum as zero sum =0 #Iterate over the list to get every element for ele in listNum: #Keep adding the element to the sum variable sum = sum + ele #returnt the sum return sum #Function to find the max of the list def listMax(listNum): #To store the max max =0 #Iterate over the list for ele in listNum: #If element is greater than max then max = element if(ele>max): max = ele #Return the max return max *************************************************************************************** Main .py #Import the module file having the 3 functions import module import random def main(): #use the random module to create the list of random integers #To store the random numbers in range 0 to 10 randomList = [] #Create the loop to create the list of 5 random integers for i in range(0,5): #Use randint to create the random integer num = random.randint(0,10) #Add the random integer into the list randomList.append(num) #Now create the menu to let user choose from what to perform on the random list print("Random list created : ",randomList,"\nSpecify what operations you wanna perform on the list") print("1:Sorting\n2:Sum of all elements \n3:Maximum\nChooce 1-3 : ",end = "") #Now take input from the user choice = int(input()) #Now use decision structures to decide which function to call from module.py if(choice == 1 ): #Print the sorted list print("Sorted list as follows : " ,module.sort(randomList)) elif(choice == 2): #Print the sum of all elements in list print("The sum of all elements in list : ",module.SumOfList(randomList)) elif(choice == 3): #print the maximum of list print("The maximum of list is : ",module.listMax(randomList)) #When the __name__ will be ___main__ the main function will be called. if __name__ == "__main__": main()
Add 3 parameters to each function in the code below. ********************************************* Code starts here ************************************************** Module.py #Defination to sort the list def sort(listNum): sortedList = [] #While loop will run until the listNum don't get null while(len(listNum) != 0 ): #Set the min as first element in list min = listNum[0] #iterate over the list to compare every element with num for ele in listNum: #If element is less than min if ele < min: #Then set min as element min = ele #append the sorted element in list sortedList.append(min) #Remove the sorted element from the list listNum.remove(min) return sortedList #Function to find the sum of all elements in list def SumOfList(listNum): #Set the sum as zero sum =0 #Iterate over the list to get every element for ele in listNum: #Keep adding the element to the sum variable sum = sum + ele #returnt the sum return sum #Function to find the max of the list def listMax(listNum): #To store the max max =0 #Iterate over the list for ele in listNum: #If element is greater than max then max = element if(ele>max): max = ele #Return the max return max *************************************************************************************** Main .py #Import the module file having the 3 functions import module import random def main(): #use the random module to create the list of random integers #To store the random numbers in range 0 to 10 randomList = [] #Create the loop to create the list of 5 random integers for i in range(0,5): #Use randint to create the random integer num = random.randint(0,10) #Add the random integer into the list randomList.append(num) #Now create the menu to let user choose from what to perform on the random list print("Random list created : ",randomList,"\nSpecify what operations you wanna perform on the list") print("1:Sorting\n2:Sum of all elements \n3:Maximum\nChooce 1-3 : ",end = "") #Now take input from the user choice = int(input()) #Now use decision structures to decide which function to call from module.py if(choice == 1 ): #Print the sorted list print("Sorted list as follows : " ,module.sort(randomList)) elif(choice == 2): #Print the sum of all elements in list print("The sum of all elements in list : ",module.SumOfList(randomList)) elif(choice == 3): #print the maximum of list print("The maximum of list is : ",module.listMax(randomList)) #When the __name__ will be ___main__ the main function will be called. if __name__ == "__main__": main()
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Add 3 parameters to each function in the code below.
********************************************* Code starts here **************************************************
Module.py
#Defination to sort the list
def sort(listNum):
sortedList = []
#While loop will run until the listNum don't get null
while(len(listNum) != 0 ):
#Set the min as first element in list
min = listNum[0]
#iterate over the list to compare every element with num
for ele in listNum:
#If element is less than min
if ele < min:
#Then set min as element
min = ele
#append the sorted element in list
sortedList.append(min)
#Remove the sorted element from the list
listNum.remove(min)
return sortedList
#Function to find the sum of all elements in list
def SumOfList(listNum):
#Set the sum as zero
sum =0
#Iterate over the list to get every element
for ele in listNum:
#Keep adding the element to the sum variable
sum = sum + ele
#returnt the sum
return sum
#Function to find the max of the list
def listMax(listNum):
#To store the max
max =0
#Iterate over the list
for ele in listNum:
#If element is greater than max then max = element
if(ele>max):
max = ele
#Return the max
return max
***************************************************************************************
Main .py
#Import the module file having the 3 functions
import module
import random
def main():
#use the random module to create the list of random integers
#To store the random numbers in range 0 to 10
randomList = []
#Create the loop to create the list of 5 random integers
for i in range(0,5):
#Use randint to create the random integer
num = random.randint(0,10)
#Add the random integer into the list
randomList.append(num)
#Now create the menu to let user choose from what to perform on the random list
print("Random list created : ",randomList,"\nSpecify what operations you wanna perform on the list")
print("1:Sorting\n2:Sum of all elements \n3:Maximum\nChooce 1-3 : ",end = "")
#Now take input from the user
choice = int(input())
#Now use decision structures to decide which function to call from module.py
if(choice == 1 ):
#Print the sorted list
print("Sorted list as follows : " ,module.sort(randomList))
elif(choice == 2):
#Print the sum of all elements in list
print("The sum of all elements in list : ",module.SumOfList(randomList))
elif(choice == 3):
#print the maximum of list
print("The maximum of list is : ",module.listMax(randomList))
#When the __name__ will be ___main__ the main function will be called.
if __name__ == "__main__":
main()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
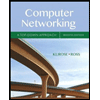
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
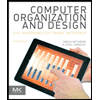
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
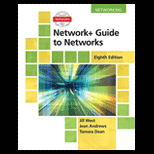
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
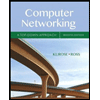
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
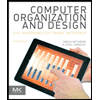
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
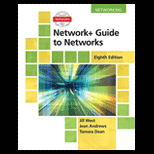
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
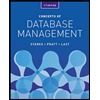
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
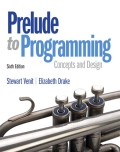
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
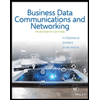
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY