Assume that XO contains a positive integer value. Write a recursive procedure with the label "fib" that calculates the X0th entry of the Fibonacci sequence, F(XO). In essence, your procedure should implement the following C function: int fib(int n) { if (n==0) return 0 else if (n=1) return 1 else return fib(n-1) + fib(n-2) } The argument will be provided in XO. Leave the result, F(XO), in X7. Note: You must implement the function using recursion! Iterative solutions will not pass! For convenience of debugging, a procedure labeled "debug:" has been defined which will print the values of all non-zero registers (through x29). Remove any calls to debug: for final submission. Answer: (penalty regime: 0 %) Reset answer 1▾ fib: 2 cmp x0, #0 3 beq base_case_zero 4 cmp x0, #1 5 beq base_case_one 6 sub x0, x0, #1 7 bl fib 8 mov x2, x7 9 sub x0, x0, #1 10 bl fib 11 add x7, x7, x2 12 ret 13 base_case_zero: 14 mov x7, #0 15 16 17 ret base_case_one: mov x7, #1 18 ret 19 Check > × Test Expected Got x0=0 ×7: x0=1 x7 1 x7: 0 x7: 1 x0=5 X7: 5 ***Time limit exceeded*** × Testing was aborted due to error. Your code must pass all tests to earn any marks. Try again.
Assume that XO contains a positive integer value. Write a recursive procedure with the label "fib" that calculates the X0th entry of the Fibonacci sequence, F(XO). In essence, your procedure should implement the following C function: int fib(int n) { if (n==0) return 0 else if (n=1) return 1 else return fib(n-1) + fib(n-2) } The argument will be provided in XO. Leave the result, F(XO), in X7. Note: You must implement the function using recursion! Iterative solutions will not pass! For convenience of debugging, a procedure labeled "debug:" has been defined which will print the values of all non-zero registers (through x29). Remove any calls to debug: for final submission. Answer: (penalty regime: 0 %) Reset answer 1▾ fib: 2 cmp x0, #0 3 beq base_case_zero 4 cmp x0, #1 5 beq base_case_one 6 sub x0, x0, #1 7 bl fib 8 mov x2, x7 9 sub x0, x0, #1 10 bl fib 11 add x7, x7, x2 12 ret 13 base_case_zero: 14 mov x7, #0 15 16 17 ret base_case_one: mov x7, #1 18 ret 19 Check > × Test Expected Got x0=0 ×7: x0=1 x7 1 x7: 0 x7: 1 x0=5 X7: 5 ***Time limit exceeded*** × Testing was aborted due to error. Your code must pass all tests to earn any marks. Try again.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter15: Recursion
Section: Chapter Questions
Problem 12PE
Question
My code does not work. Assume that X0 contains a positive integer value. Write a recursive procedure with the label "fib" that calculates the X0th entry of the Fibonacci sequence, F(X 0). In essence, your procedure should implement the following C function: int fib(int n) (if (n==0) return 0 else if (n == 1) return 1 else return fib(n-1)+fib(n-2)) The argument will be provided in XO. Leave the result, F(X0), in X7. Note: You must implement the function using recursion! Iterative solutions will not pass! For convenience of debugging, a procedure labeled "debug:" has been defined which will print the values of all non - zero registers (through x29). Remove any calls to debug: for final submission.

Transcribed Image Text:Assume that XO contains a positive integer value. Write a recursive procedure with the label "fib" that calculates the X0th entry of the Fibonacci sequence, F(XO). In essence, your procedure should implement the following C function:
int fib(int n) {
if (n==0)
return 0
else if (n=1)
return 1
else
return fib(n-1) + fib(n-2)
}
The argument will be provided in XO. Leave the result, F(XO), in X7.
Note: You must implement the function using recursion! Iterative solutions will not pass!
For convenience of debugging, a procedure labeled "debug:" has been defined which will print the values of all non-zero registers (through x29). Remove any calls to debug: for final submission.
Answer: (penalty regime: 0 %)
Reset answer
1▾ fib:
2
cmp x0, #0
3
beq base_case_zero
4
cmp x0, #1
5
beq base_case_one
6
sub x0, x0, #1
7
bl fib
8
mov x2, x7
9
sub x0, x0, #1
10
bl fib
11
add x7, x7, x2
12
ret
13 base_case_zero:
14
mov x7, #0
15
16
17
ret
base_case_one:
mov x7, #1
18
ret
19
Check
>
×
Test Expected Got
x0=0
×7:
x0=1 x7 1
x7: 0
x7: 1
x0=5 X7: 5
***Time limit exceeded*** ×
Testing was aborted due to error.
Your code must pass all tests to earn any marks. Try again.
AI-Generated Solution
Unlock instant AI solutions
Tap the button
to generate a solution
Recommended textbooks for you
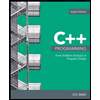
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
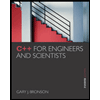
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
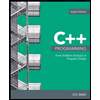
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
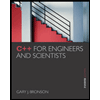
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr