Boats For problems A, B, and C you will be writing two different classes to simulate a Boat race. Problem A is to write the first class Boat. A Boat object must hold the following information boat_name: string top_speed: int current_progress: int Write a constructor that allows the programmer to create an object of type Boat with the arguments boat_name and top_speed. The boat_name should be set to the value of the corresponding argument - this argument is required. The top_speed should default to the value 3 if no value is passed in for the argument. The value for current_progress should always be set to 0. Implement Boat class with setter and getter methods: Provide setters for the following instance variables: set_top_speed takes in an int and updates the top_speed set_boat_name takes in a string and updates the boat_name set_current_progress takes in a int and updates the current_progress Provide getters for the following instance variables with no input parameters passed in: get_boat_name returns the boat_name get_top_speed returns the top_speed get_current_progress returns the current_progress Overload the __str__ method so that it returns a string containing the boat_name and current_progress. The string should look like the following example. Please note there is only 1 space after the colon. Whirlwind: 0 A method named move which takes no arguments (other than self) and returns an int. The move method should select a random integer between 0 and top_speed (inclusive on both sides), then increment current_progress by that random value, and finally return that random value.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Boats
For problems A, B, and C you will be writing two different classes to simulate a Boat race. Problem A is to write the first class Boat. A Boat object must hold the following information
- boat_name: string
- top_speed: int
- current_progress: int
- Write a constructor that allows the programmer to create an object of type Boat with the arguments boat_name and top_speed.
- The boat_name should be set to the value of the corresponding argument - this argument is required.
- The top_speed should default to the value 3 if no value is passed in for the argument.
- The value for current_progress should always be set to 0.
- Implement Boat class with setter and getter methods:
- Provide setters for the following instance variables:
- set_top_speed takes in an int and updates the top_speed
- set_boat_name takes in a string and updates the boat_name
- set_current_progress takes in a int and updates the current_progress
- Provide getters for the following instance variables with no input parameters passed in:
- get_boat_name returns the boat_name
- get_top_speed returns the top_speed
- get_current_progress returns the current_progress
- Overload the __str__ method so that it returns a string containing the boat_name and current_progress. The string should look like the following example. Please note there is only 1 space after the colon.
Whirlwind: 0
- A method named move which takes no arguments (other than self) and returns an int. The move method should select a random integer between 0 and top_speed (inclusive on both sides), then increment current_progress by that random value, and finally return that random value.
Examples:
Copy the following if __name__ == "__main__" block into your hw11.py file, and comment out tests for parts of the class you haven’t implemented yet. The lines that have output include the expected value next to them as a comment (note that some lines are random and your output will not match exactly).
if __name__ == '__main__':
my_boat = Boat("The Fire Ball", 12)
other_boat = Boat("The Leaf", 100)
print(type(my_boat)) #<class '__main__.Boat'>
print(str(my_boat)) #The Fire Ball: 0
print(my_boat.get_boat_name()) #The Fire Ball
print(my_boat.get_top_speed()) #12
print(my_boat.get_current_progress()) #0
print(my_boat.move()) #Some number between 0 and 12
print(my_boat.get_current_progress()) #Same num as prev line
print(my_boat.move()) #Another number between 0 and 12
print(my_boat.get_current_progress()) #Sum of prev 2 lines
my_boat.set_top_speed(100)
my_boat.set_boat_name("The Sam")
my_boat.set_current_progress(1000)
print(my_boat) #The Sam: 1000
print(my_boat.get_current_progress()) #1000
for i in range(9980):
my_boat.move()
print(my_boat.get_current_progress()) #Should be about 500000

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

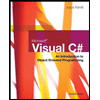
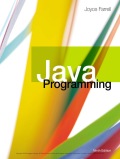
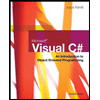
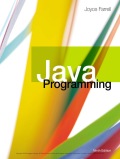