Boolean fields that represent whether the applicant is skilled in each of the following areas: word processing as hasWordSkill, spreadsheets as hasSpreadsheetSkill, databases as hasDatabaseSkill, and graphics as hasGraphicsSkill
Part A
Create a class named JobApplicant that holds data about a job applicant. Include a name as name, a phone number as phone, and four Boolean fields that represent whether the applicant is skilled in each of the following areas: word processing as hasWordSkill, spreadsheets as hasSpreadsheetSkill, databases as hasDatabaseSkill, and graphics as hasGraphicsSkill.
Include a blank constructor that takes no arguments and an overloaded constructor that accepts values for each of the fields.
Also include a get method for each field.
Part B
Create an application called TestJobApplicants that instantiates three JobApplicant objects in the main() method. Pass each object, in turn, to a method called getData() that gets user data for each field: the user enters Strings for the name and phone number, and enters four integers that indicate whether the applicant possesses each of the four skills. Return a complete JobApplicant object to the main() method.
After the objects are returned to the main() method, pass each to a boolean method called isQualified() that determines whether an applicant is qualified for an interview and returns true or false. A qualified applicant has at least three of the four skills. Then, in the main() method, display a message that includes the applicant’s name, phone number, and whether the applicant is qualified.
An example of the program is shown below:
Enter applicant's name >> John Smith
Enter applicant's phone number >> 456-7890
Is applicant skilled in word processing?
Enter 1 for yes or 2 for no >> 1
Is applicant skilled in spreadsheet?
Enter 1 for yes or 2 for no >> 1
Is applicant skilled in
Enter 1 for yes or 2 for no >> 2
Is applicant skilled in graphics?
Enter 1 for yes or 2 for no >> 2
... John Smith is not qualified for an interview at this time Phone: 456-7890 Jane Doe is not qualified for an interview at this time Phone: 555-0155 Iris is qualified for an interview Phone: 765-4321
Task 02: Add the data fields to the JobApplicant class.
Task 03: Define a blank constructor with no arguments and an overloaded constructor for the JobApplicant class.
Task 04: Define get methods for the name and phone data fields.
Task 05: Define get methods for hasWordSkill, hasSpreadsheetSkill, hasDatabaseSkill, and hasGraphicsSkill data fields.
Task 06: Create the TestJobApplicants class.
Task 07: Define the getData() method of the TestJobApplicants class.
Task 08: Define the isQualified() method of the TestJobApplicants class.
![|||| 0 a 2 400
G
Unit 5: Making Decisions
CENGAGE COMPANION: COMMANDS
Open Companion Tab
Refresh Companion Tab
Bundle Current Workspace
https://literate-sniffle-x5wx9wg5x7w4fr5r.github.dev/?folder=/workspaces/9780357673423_java-programming-10e-5...
J JobApplicant.java X J TestJobApplicants.java
J JobApplicantjava > JobApplicant
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48 }
MindTap - Cengage Learning X
// getter for the applicant's spreadsheet skills
public boolean getHasSpreadsheetskill() {
return this.hasSpreadsheetskill;
}
// getter for the applicant's database skills
public boolean getHasDatabaseskill() {
return hasDatabaseskill;
// getter for the applicant's graphics skills
public boolean getHasGraphicsskill() {
return hasGraphicsskill;
PROBLEMS OUTPUT DEBUG CONSOLE TERMINAL PORTS 1
o @jbowen3225
Companion - student [Codespace X
€33
> Codespaces: literate sniffle Ⓡ0A01 Java: Lightweight Mode
student [Codespaces: literate sniffle]
POLAR
HO
D
HO
Success Confirmation of Question X +
а
Q All
{}
→.../9788357673423_java-programming-18e-5d83f9ac-c9c6-4288-9e22-37326c529145/chapter5/ex87/student (terplate) $
()
·[+]
Welcome
= Companion X
metnoa called isųuall+lea() that determines wnetner an appucant is qualit
for an interview and returns true or false. A qualified applicant has at least th
of the four skills. Then, in the main() method, display a message that includes
applicant's name, phone number, and whether the applicant is qualified.
An example of the program is shown below:
Enter applicant's name >> John Smith
Enter applicant's phone number >> 456-7890
Is applicant skilled in word processing?
Enter 1 for yes or 2 for no >> 1
Is applicant skilled in spreadsheet?
Enter 1 for yes or 2 for no >> 1
Is applicant skilled in database?
Enter 1 for yes or 2 for no >> 2
Is applicant skilled in graphics?
Enter 1 for yes or 2 for no >> 2
John Smith is not qualified for an interview at this time Phone: 456-7:
Jane Doe is not qualified for an interview at this time Phone: 555-015!
Iris is qualified for an interview Phone: 765-4321
4
P
JO
08
...
bash +v0. ^ x
Layout: US O](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6cfcbabe-0283-4f69-afa3-58c47651b2d0%2Fe46851f0-89e5-425a-a716-e75ca480fba7%2Fjzlc0m_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

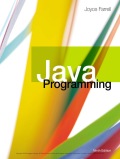
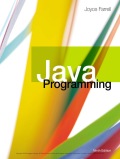