c class Assignment7 { /** * You do NOT need to make any changes to the main method. You need to write three methods that perform the action * described below. * * The main me
in java:
import java.util.Arrays;
public class Assignment7 {
/**
* You do NOT need to make any changes to the main method. You need to write three methods that perform the action
* described below.
*
* The main method will help you test if your method works or not.
* Notice the method names, the arguments/parameters it needs to have, and the return type of the method. Use
* that to create your methods below.
*/
public static void main(String[] args) {
System.out.println("Once you implement no23 method, it should print out: false");
int[] array0 = {3, 5, 6};
System.out.println("Your implementation of no23 method printed out: " + no23(array0));
System.out.println("\nOnce you implement lucky13 method, it should print out: false");
int[] array1 = {1, 3, 5, 6};
System.out.println("Your implementation of lucky13 method printed out: " +lucky13(array1));
System.out.println("\nOnce you implement plusTwo method, it should print out: [1, 9, 2, 4, 6]");
int[] array2 = {1, 9};
int[] array3 = {2, 4, 6};
// We use a method called toString to print the array. Do not worry about it.
System.out.println("Your implementation of lucky13 method printed out: " + Arrays.toString(plusTwo(array2, array3)));
}
/** Method 1
Given an int array of any given length, return true if it does not contain a 2 or 3.
no23([4, 5, 6, 7, 8]) → true
no23([4, 2, 1, 1, 1]) → false
no23([3, 5, 3, 6, 1]) → false
Key ideas:boolean return type; array basic loop; condition check
**/
/** Method 2
Given an array of ints, return true if the array does not contain 1 and 3 both (anywhere in the array).
lucky13([0, 2, 4]) → true
lucky13([1, 2, 3]) → false
lucky13([1, 2, 4]) → true
lucky13([2, 3, 4]) → true
lucky13([3, 1, 4]) → false
lucky13([1, 3]) → false
lucky13([3,3,1]) → false
Key ideas: basic for-each loop (or for loop); iterating through loop; conditional check
**/
/** Method 3
Given 2 int arrays, return a new array length containing all the elements.
plusTwo([1, 2], [3, 4, 5]) → [1, 2, 3, 4, 5]
plusTwo([4], [2, 2, 2]) → [4, 2, 2, 2]
plusTwo([9, 2, 3, 5, 7], [3, 4, 1, 4, 5]) → [9, 2, 3, 5, 7, 3, 4, 1, 4, 5]
Key ideas: creating arrays; for loops; using .length property; returning an array
**/

Step by step
Solved in 4 steps with 2 images

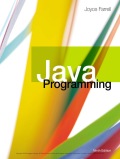
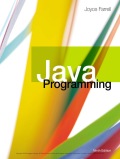