C++ program
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter13: Structures
Section: Chapter Questions
Problem 2PP
Related questions
Question
C++ program
#include <iostream>
#include <string>
#include<cstring>
using namespace std;
const int NAME_SIZE = 20;
const int STREET_SIZE = 30;
const int CITY_SIZE = 20;
const int STATE_CODE_SIZE = 3;
class Customer {
long customerNumber;
char name[NAME_SIZE];
char streetAddress_1[STREET_SIZE];
char streetAddress_2[STREET_SIZE];
char city[CITY_SIZE];
char state[STATE_CODE_SIZE];
int zipCode;
public:
void setCustomerNumber(long customerNo)
{
customerNumber = customerNo;
}
bool setName(char name[])
{
if (strlen(name) <= NAME_SIZE)
{
(this->name, name);
returntrue;
}
returnfalse;
}
bool setStreetAddress_1(char streetAddress_1[])
{
if (strlen(streetAddress_1) <= STREET_SIZE)
{
(this->streetAddress_1, streetAddress_1);
returntrue;
}
returnfalse;
}
bool setStreetAddress_2(char streetAddress_2[])
{
if (strlen(streetAddress_2) <= STREET_SIZE)
{
(this->streetAddress_2, streetAddress_2);
returntrue;
}
returnfalse;
}
bool setCity(char city[])
{
if (strlen(city) <= CITY_SIZE)
{
for (int i = 0; city[i] != '\0'; i++)
this->city[i] = toupper(city[i]);
this->city[strlen(city) - 1] = '\0';
returntrue;
}
returnfalse;
}
bool setState(char state[])
{
if (strlen(state) <= STATE_CODE_SIZE)
{
for (int i = 0; state[i] != '\0'; i++)
this->state[i] = toupper(state[i]);
this->state[strlen(state) - 1] = '\0';
returntrue;
}
returnfalse;
}
bool setZipCode(int zipCode)
{
if (zipCode > 0 && zipCode < 99999)
{
this->zipCode = zipCode;
returntrue;
}
returnfalse;
}
void print()
{
cout << " Customer Number : " << customerNumber << endl;
cout << " Name : " << name << endl;
cout << " Street Address 1 :" << streetAddress_1 << endl;
cout << " Street Address 2 : " << streetAddress_2 << endl;
cout << " City : " << city << endl;
cout << " State : " << state << endl;
cout << " Zipcode : " << zipCode << endl;
}
};
int main() {
Customer customer;
char name[100], strAdd1[100], strAdd2[100], city[100], state[100];
int zip;
srand(time(NULL));
long custNo;
cout << " Name : ";
cin.getline(name, sizeof(name), '\n');
while (!customer.setName(name))
{
cout << " Invalid name.\n Name : ";
cin.getline(name, sizeof(name), '\n');
}
cout << " Street Address 1 : ";
cin.getline(strAdd1, sizeof(strAdd1), '\n');
while (!customer.setStreetAddress_1(strAdd1))
{
cout << " Invalid Street Address1.\n Street Address 1 : ";
cin.getline(strAdd1, sizeof(strAdd1), '\n');
}
cout << " Street Address 2 : ";
cin.getline(strAdd2, sizeof(strAdd2), '\n');
while (!customer.setStreetAddress_2(strAdd2))
{
cout << " Invalid Street Address 2.\n Street Address 2 : ";
cin.getline(strAdd2, sizeof(strAdd2), '\n');
}
cout << " City : ";
cin.getline(city, sizeof(city), '\n');
while (!customer.setCity(city))
{
cout << " Invalid City. \n City: ";
cin.getline(city, sizeof(city), '\n');
}
cout << " State : ";
cin.getline(state, sizeof(state), '\n');
while (!customer.setState(state))
{
cout << " Invalid State.\n State: ";
cin.getline(state, sizeof(state), '\n');
}
cout << " Zip code :";
cin >> zip;
while (!customer.setZipCode(zip))
{
cout << " Invalid Zip code.\n Zip code :";
cin >> zip;
}
custNo = (long)((rand() % 9000) + 1000);// randomly generate customer number between 1000-9999
customer.setCustomerNumber(custNo);
cout << "\n Customer : " << endl;
customer.print();
return0;
}
Refactor Assignment 1 into 3 project related files.
Customer.h - Class SpecificationCustomer.cpp - Class Implementation (Methods)TestCustomer.cpp - Your code that performs the logic from Assignment 1.
The 3 files need to be named as listed above and should compile without errors.
Deliverables are the 3 files listed above. This can be in a zip file. These files need to match the layouts as described in the chapter. Be aware the const items can be in the header file.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
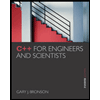
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
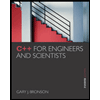
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr