Change the last bolded part of code to using a function #include #include #include // function sum() -> Returns the sum of the elements of the array int sum(int array[]) { int i, sum = 0; for(i = 0; i < 5; i++) { sum += array[i]; // Summing up the elements } return sum; // Returning the sum } // function average() -> Returns the average of an array float average(int array[]) { int tot; float avg; tot = sum(array); // Calling the function sum() -> To get the sum of the elements of the array avg = tot / 5.0; // Computing the average return avg; // Returning the average } int main() { int a[5][5]; // 2-D array int i, j; int row[5]; // To store each row of the 2-D array float avg[5]; // To store the average of each of the 5 rows of the 2-D array int d1[5], d2[5]; // To store the diagonals of the 2-D array int sumD1, sumD2; // To store the sum of the 2 diagonals of the 2-D array srand(time(0)); for(i = 0; i < 5; i++) { for(j = 0; j < 5; j++) { a[i][j] = (rand() % 10) + 1; // Storing random integers in the 2-D array } } for(i = 0; i < 5; i++) { for(j = 0; j < 5; j++) { row[j] = a[i][j]; // Filling array- row with each row of the 2-D array } avg[i] = average(row); // Computing the average of each row by calling the function average() -> To get the average of the row } for(i = 0; i < 5; i++) { for(j = 0; j < 5; j++) { if(i == j) d1[i] = a[i][j]; // Filling the diagonal array if((i + j) == 4) d2[i] = a[i][j]; // Filling the diagonal array } } sumD1 = sum(d1); // Calling the function sum() -> To get the sum of the elements of the diagonal sumD2 = sum(d2); // Calling the function sum() -> To get the sum of the elements of the diagonal printf(" \t \t \t \t \tAverage\n"); for(i = 0; i < 5; i++) { for(j = 0; j < 5; j++) { printf("%d\t", a[i][j]); // Displaying elements of the row } printf("%.1f\n", avg[i]); // Displaying the average of the row } // Displaying the greater diagonal if(sumD1 < sumD2) { printf("\nThe top right to bottom left diagonal's total is greater and the value is %d.", sumD2); } else if(sumD1 > sumD2){ printf("\nThe top top left to bottom right diagonal's total is greater and the value is %d.", sumD1); } else { printf("\nNo diagonal is greater. The values are %d and %d.", sumD1, sumD2); } return 0; }
Change the last bolded part of code to using a function
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// function sum() -> Returns the sum of the elements of the array
int sum(int array[]) {
int i, sum = 0;
for(i = 0; i < 5; i++) {
sum += array[i]; // Summing up the elements
}
return sum; // Returning the sum
}
// function average() -> Returns the average of an array
float average(int array[]) {
int tot;
float avg;
tot = sum(array); // Calling the function sum() -> To get the sum of the elements of the array
avg = tot / 5.0; // Computing the average
return avg; // Returning the average
}
int main() {
int a[5][5]; // 2-D array
int i, j;
int row[5]; // To store each row of the 2-D array
float avg[5]; // To store the average of each of the 5 rows of the 2-D array
int d1[5], d2[5]; // To store the diagonals of the 2-D array
int sumD1, sumD2; // To store the sum of the 2 diagonals of the 2-D array
srand(time(0));
for(i = 0; i < 5; i++) {
for(j = 0; j < 5; j++) {
a[i][j] = (rand() % 10) + 1; // Storing random integers in the 2-D array
}
}
for(i = 0; i < 5; i++) {
for(j = 0; j < 5; j++) {
row[j] = a[i][j]; // Filling array- row with each row of the 2-D array
}
avg[i] = average(row); // Computing the average of each row by calling the function average() -> To get the average of the row
}
for(i = 0; i < 5; i++) {
for(j = 0; j < 5; j++) {
if(i == j)
d1[i] = a[i][j]; // Filling the diagonal array
if((i + j) == 4)
d2[i] = a[i][j]; // Filling the diagonal array
}
}
sumD1 = sum(d1); // Calling the function sum() -> To get the sum of the elements of the diagonal
sumD2 = sum(d2); // Calling the function sum() -> To get the sum of the elements of the diagonal
printf(" \t \t \t \t \tAverage\n");
for(i = 0; i < 5; i++) {
for(j = 0; j < 5; j++) {
printf("%d\t", a[i][j]); // Displaying elements of the row
}
printf("%.1f\n", avg[i]); // Displaying the average of the row
}
// Displaying the greater diagonal
if(sumD1 < sumD2) {
printf("\nThe top right to bottom left diagonal's total is greater and the value is %d.", sumD2);
} else if(sumD1 > sumD2){
printf("\nThe top top left to bottom right diagonal's total is greater and the value is %d.", sumD1);
} else {
printf("\nNo diagonal is greater. The values are %d and %d.", sumD1, sumD2);
}
return 0;
}

Step by step
Solved in 2 steps with 1 images

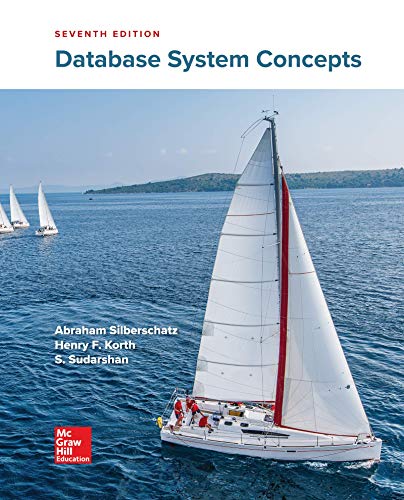
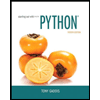
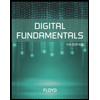
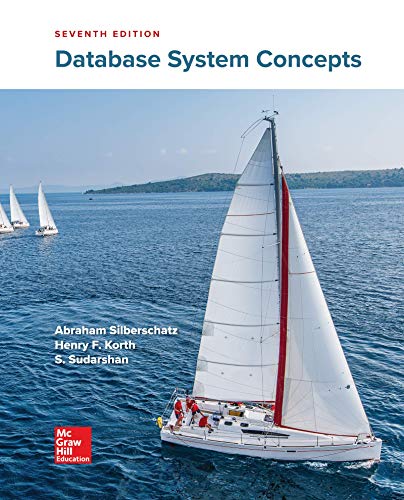
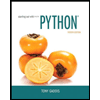
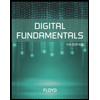
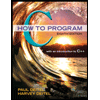
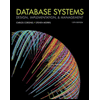
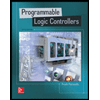