3 9 10 11 12 det verity(number): # do not change this line! # write your code here so that it verifies the card number # be sure to indent your code! return True # modify this line as needed input = "5000-0000-0000" # change this as you test your function output = verify(input) # invoke the method using a test input print(output) # prints the output of the function # do not remove this line! he rules must be checked in this order, and if any of the rules are violated, the
3 9 10 11 12 det verity(number): # do not change this line! # write your code here so that it verifies the card number # be sure to indent your code! return True # modify this line as needed input = "5000-0000-0000" # change this as you test your function output = verify(input) # invoke the method using a test input print(output) # prints the output of the function # do not remove this line! he rules must be checked in this order, and if any of the rules are violated, the
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter15: Recursion
Section: Chapter Questions
Problem 20PE
Related questions
Question

Transcribed Image Text:12345678
9
10
11
12
def verify(number)
: # do not change this line!
#write your code here so that it verifies the card number
# be sure to indent your code!
return True # modify this line as needed
input = "5000-0000-0000" # change this as you test your function
output = verify(input) # invoke the method using a test input
print(output) # prints the output of the function
# do not remove this line!
The rules must be checked in this order, and if any of the rules are violated, the function
should return the violated rule number, e.g. if the input is "4238-0679-9123", then the
function should return 2, indicating that rule #2 was violated because although rule #1
was satisfied (the first digit is a 4), rule #2 was not, since the fourth digit (which is 8) is
not one greater than the fifth (which is 0). If all rules are satisfied, then the function
should return True.
Note that the card number is not actually a number, but is a string of characters. In
Python, you can generally use a string the same way you would use a list, e.g.
accessing individual characters using their 0-based index. Hint: You will need to do this
for checking all the rules.
However, when you access a character using its 0-based index, Python will treat it as a
character/letter and not a number, even if it's a digit, and you need to be careful about
how you use it in mathematical operations. For instance, if you had the characters '1'
and '2' and try to add them, Python would concatenate them and use them to form a
longer string; in this case, you would get "12". However, if you try to subtract, multiply,
divide, etc. then Python will give you an error.
To convert a character/letter to a number, use the "int" function, e.g. "x = int('1')" will
convert the character/letter '1' to the number 1 so that you can use it in mathematical
operations. Hint: you will need this for rules 2-4.

Transcribed Image Text:Description
Credit card companies and banks use built-in security measures when creating the
account numbers on credit cards to make sure the card numbers follow certain rules
(you didn't think they were random, did you?). This means that there are only certain
valid credit card numbers, and validity can quickly be detected by using an algorithm
that may involve adding up parts of the numbers or performing other checks.
In this activity, you will implement a function that determines whether or not a card
number is valid, according to some simple algorithms. Note that these algorithms are
purely made-up; don't try to use them to create fake credit card numbers! :-)
We will assume that the credit card number is a string consisting of 14 characters and is
in the format #### #### ####, including the dashes, where '#' represents a digit
between 0-9, so that there are 12 digits overall. We will revisit this assumption in the an
optional later activity.
In the space below, implement a function called "verify" that takes a single parameter
called "number" and then checks the following rules:
1. The first digit must be a 4.
2. The fourth digit must be one greater than the fifth digit; keep in mind that these
are separated by a dash since the format is ####-####-
3. The sum of all digits must be evenly divisible by 4.
4. If you treat the first two digits as a two-digit number, and the seventh and eighth
digits as a two-digit number, their sum must be 100.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
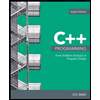
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
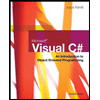
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
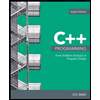
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
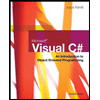
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,