Exception in thread "main" java.lang.Error: Unresolved compilation problem: Tree cannot be resolved to a type at Exercise28_05$UnweightedGraphWithGetPath.getPath(Exercise28_05.java:80) at Exercise28_05.(Exercise28_05.java:35) at Exercise28_05.main(Exercise28_05.java:8) Here is the part of my code that is experiencing issues: import java.util.*; import java.io.*; public class Exercise28_05 { public static void main(String[] args) throws Exception { new Exercise28_05(); } public Exercise28_05() throws Exception { String[] vertices = { "Seattle", "San Francisco", "Los Angeles", "Denver", "Kansas City", "Chicago", "Boston", "New York", "Atlanta", "Miami", "Dallas", "Houston" }; int[][] edges = { { 0, 1 }, { 0, 3 }, { 0, 5 }, { 1, 0 }, { 1, 2 }, { 1, 3 }, { 2, 1 }, { 2, 3 }, { 2, 4 }, { 2, 10 }, { 3, 0 }, { 3, 1 }, { 3, 2 }, { 3, 4 }, { 3, 5 }, { 4, 2 }, { 4, 3 }, { 4, 5 }, { 4, 7 }, { 4, 8 }, { 4, 10 }, { 5, 0 }, { 5, 3 }, { 5, 4 }, { 5, 6 }, { 5, 7 }, { 6, 5 }, { 6, 7 }, { 7, 4 }, { 7, 5 }, { 7, 6 }, { 7, 8 }, { 8, 4 }, { 8, 7 }, { 8, 9 }, { 8, 10 }, { 8, 11 }, { 9, 8 }, { 9, 11 }, { 10, 2 }, { 10, 4 }, { 10, 8 }, { 10, 11 }, { 11, 8 }, { 11, 9 }, { 11, 10 } }; UnweightedGraphWithGetPath graph = new UnweightedGraphWithGetPath<>( vertices, edges); Scanner input = new Scanner(System.in); System.out.print("Enter a starting city: "); String startingCity = input.nextLine(); System.out.print("Enter an ending city: "); String endingCity = input.nextLine(); List list = graph.getPath(graph.getIndex(startingCity), graph.getIndex(endingCity)); System.out.print("The path is "); for (Integer i : list) { System.out.print(graph.getVertex(i) + " "); } } // BEGIN REVEL SUBMISSION class UnweightedGraphWithGetPath extends UnweightedGraph { /** Construct an empty graph */ public UnweightedGraphWithGetPath() { } /** Construct a graph from vertices and edges stored in arrays */ public UnweightedGraphWithGetPath(V[] vertices, int[][] edges) { super(vertices, edges); } /** Construct a graph from vertices and edges stored in List */ public UnweightedGraphWithGetPath(List vertices, List edges) { super(vertices, edges); } /** Construct a graph for integer vertices 0, 1, 2 and edge list */ public UnweightedGraphWithGetPath(List edges, int numberOfVertices) { super(edges, numberOfVertices); } /** Construct a graph from integer vertices 0, 1, and edge array */ public UnweightedGraphWithGetPath(int[][] edges, int numberOfVertices) { super(edges, numberOfVertices); } public List getPath(int u, int v) { Tree tree = bfs(u); ArrayList path = new ArrayList<>(); do { path.add(v); v = tree.parent[v]; } while (v != -1); Collections.reverse(path); return path; } }
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
Tree cannot be resolved to a type
at Exercise28_05$UnweightedGraphWithGetPath.getPath(Exercise28_05.java:80)
at Exercise28_05.<init>(Exercise28_05.java:35)
at Exercise28_05.main(Exercise28_05.java:8)
Here is the part of my code that is experiencing issues:
import java.util.*;
import java.io.*;
public class Exercise28_05
{
public static void main(String[] args) throws Exception
{
new Exercise28_05();
}
public Exercise28_05() throws Exception
{
String[] vertices = { "Seattle", "San Francisco", "Los Angeles", "Denver",
"Kansas City", "Chicago", "Boston", "New York", "Atlanta", "Miami",
"Dallas", "Houston" };
int[][] edges = { { 0, 1 }, { 0, 3 }, { 0, 5 }, { 1, 0 }, { 1, 2 },
{ 1, 3 }, { 2, 1 }, { 2, 3 }, { 2, 4 }, { 2, 10 }, { 3, 0 }, { 3, 1 },
{ 3, 2 }, { 3, 4 }, { 3, 5 }, { 4, 2 }, { 4, 3 }, { 4, 5 }, { 4, 7 },
{ 4, 8 }, { 4, 10 }, { 5, 0 }, { 5, 3 }, { 5, 4 }, { 5, 6 }, { 5, 7 },
{ 6, 5 }, { 6, 7 }, { 7, 4 }, { 7, 5 }, { 7, 6 }, { 7, 8 }, { 8, 4 },
{ 8, 7 }, { 8, 9 }, { 8, 10 }, { 8, 11 }, { 9, 8 }, { 9, 11 },
{ 10, 2 }, { 10, 4 }, { 10, 8 }, { 10, 11 }, { 11, 8 }, { 11, 9 },
{ 11, 10 } };
UnweightedGraphWithGetPath<String> graph = new UnweightedGraphWithGetPath<>(
vertices, edges);
Scanner input = new Scanner(System.in);
System.out.print("Enter a starting city: ");
String startingCity = input.nextLine();
System.out.print("Enter an ending city: ");
String endingCity = input.nextLine();
List<Integer> list = graph.getPath(graph.getIndex(startingCity),
graph.getIndex(endingCity));
System.out.print("The path is ");
for (Integer i : list)
{
System.out.print(graph.getVertex(i) + " ");
}
}
// BEGIN REVEL SUBMISSION
class UnweightedGraphWithGetPath<V> extends UnweightedGraph<V>
{
/** Construct an empty graph */
public UnweightedGraphWithGetPath()
{
}
/** Construct a graph from vertices and edges stored in arrays */
public UnweightedGraphWithGetPath(V[] vertices, int[][] edges)
{
super(vertices, edges);
}
/** Construct a graph from vertices and edges stored in List */
public UnweightedGraphWithGetPath(List<V> vertices, List<Edge> edges)
{
super(vertices, edges);
}
/** Construct a graph for integer vertices 0, 1, 2 and edge list */
public UnweightedGraphWithGetPath(List<Edge> edges, int numberOfVertices)
{
super(edges, numberOfVertices);
}
/** Construct a graph from integer vertices 0, 1, and edge array */
public UnweightedGraphWithGetPath(int[][] edges, int numberOfVertices)
{
super(edges, numberOfVertices);
}
public List<Integer> getPath(int u, int v)
{
Tree tree = bfs(u);
ArrayList<Integer> path = new ArrayList<>();
do
{
path.add(v);
v = tree.parent[v];
} while (v != -1);
Collections.reverse(path);
return path;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

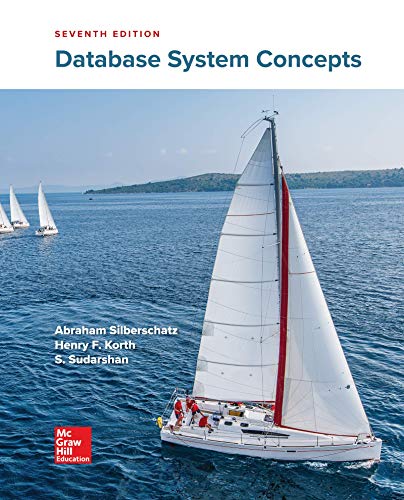
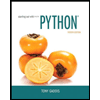
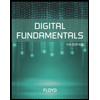
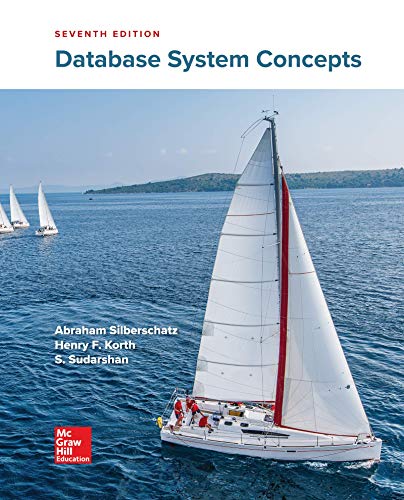
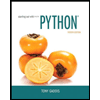
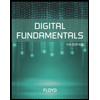
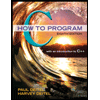
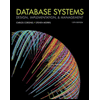
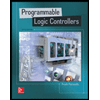