Genco Pura Olive Oil has a file that contains its customers, a file that contains the customers’ orders, and has begun creating a program to read the files and print the contents in a human-readable format. Files to submit: Customer.py runCustomer.py Input files: There are two input files. The first one is called customers.txt. Each line of this input file contains a customer number and name separated by a comma. The second input file is called orders.txt. This file contains lines each corresponding to an order. Each line contains a customer number, the order date, a product code, a product name, quantity, and order total all separated by commas. Customer.py In a file called Customer.py, create a class called Customer. My file would be called conwayCustomer.py and my class would be called ConwayCustomer. Attributes The class will have three attributes (also called instance variables): an int called number, a string called name, and a list called orders. The list will contain a list of tuples, each corresponding to an order for that customer. __init__() Define a constructor (init) method. In addition to self, the constructor will define two more parameters, one for customer number, and one for customer name. Set the list (called orders) to an empty list. addOrder() Define a method addOrder(). In addition to self, this method will define one more parameter, a tuple called order. Add this tuple to the list attribute called orders. __str__() Define the __str__() method. An object’s str() method builds and returns a string representation of an object’s state. This allows an object to be sent to the print() function (as is occurring in the main method). Create a string that includes the customer’s name and number. Then append to this string all the orders in the orders list. Loop over the list and add the items in the tuple to the string. The items in a tuple can be accessed the same way the items in a list can be accessed – using the name of the tuple and the index as a subscript. runCustomer.py A file called runCustomer.py is provided to you and is partially completed. Change this filename to runCustomer.py. My file would be called runConwayCustomer.py. This file contains an import statement, a main method, a function called readCustomers(), and the function header of a function you will create called readOrders(). You will have to modify the import statement to from Customer import Customer. My import statement would be from conwayCustomer import ConwayCustomer The main method calls a function called readCustomers() that opens an input file, reads the contents, and returns a dictionary. It then calls a function called readOrders() that takes that dictionary as a parameter; this function will read from an input file called orders.txt and add the orders to the appropriate customer. The main method then loops through the customer dictionary and prints each customer. Function readCustomers() The function readCustomers() is already written. This function opens the input file, customers.txt, for reading. An empty dictionary is created. The dictionary will map a customer’s number to a customer object. As each line of the input file is read in as a string, the string is split using the comma delimiter. This returns a list. The first item in the resulting list is the customer number and the second item is the customer name. The customer object is created by calling the Customer class’ constructor (init) method using these values. An entry is made into the dictionary using the customer number as the key and the Customer object as the value (you’ll have to modify this to reflect your class name). Finally, the dictionary is returned. Function readOrders() You are creating the readOrders() function. This function takes as its parameter the dictionary filled with customer number/customer object key/value pairs. This function should open the input file, orders.txt, for reading. It should then loop through the file in a similar way as the readCustomers() function, as the values are also delimited by commas. Get the customer object from the dictionary. This can be done using the dictionary get() method and sending the customer number. The get method can take a second argument that is returned if the customer number is not a key in the dictionary. If the customer exists in the dictionary, create a tuple consisting of the date (a string), the product code (a string), a product name (a string), the quantity (an int), and the order total (a float). Add this tuple to the customer’s list of orders by sending it to the customer’s addOrder() method.
Genco Pura Olive Oil has a file that contains its customers, a file that contains the customers’ orders, and has begun creating a program to read the files and print the contents in a human-readable format. Files to submit: Customer.py runCustomer.py Input files: There are two input files. The first one is called customers.txt. Each line of this input file contains a customer number and name separated by a comma. The second input file is called orders.txt. This file contains lines each corresponding to an order. Each line contains a customer number, the order date, a product code, a product name, quantity, and order total all separated by commas. Customer.py In a file called Customer.py, create a class called Customer. My file would be called conwayCustomer.py and my class would be called ConwayCustomer. Attributes The class will have three attributes (also called instance variables): an int called number, a string called name, and a list called orders. The list will contain a list of tuples, each corresponding to an order for that customer. __init__() Define a constructor (init) method. In addition to self, the constructor will define two more parameters, one for customer number, and one for customer name. Set the list (called orders) to an empty list. addOrder() Define a method addOrder(). In addition to self, this method will define one more parameter, a tuple called order. Add this tuple to the list attribute called orders. __str__() Define the __str__() method. An object’s str() method builds and returns a string representation of an object’s state. This allows an object to be sent to the print() function (as is occurring in the main method). Create a string that includes the customer’s name and number. Then append to this string all the orders in the orders list. Loop over the list and add the items in the tuple to the string. The items in a tuple can be accessed the same way the items in a list can be accessed – using the name of the tuple and the index as a subscript. runCustomer.py A file called runCustomer.py is provided to you and is partially completed. Change this filename to runCustomer.py. My file would be called runConwayCustomer.py. This file contains an import statement, a main method, a function called readCustomers(), and the function header of a function you will create called readOrders(). You will have to modify the import statement to from Customer import Customer. My import statement would be from conwayCustomer import ConwayCustomer The main method calls a function called readCustomers() that opens an input file, reads the contents, and returns a dictionary. It then calls a function called readOrders() that takes that dictionary as a parameter; this function will read from an input file called orders.txt and add the orders to the appropriate customer. The main method then loops through the customer dictionary and prints each customer. Function readCustomers() The function readCustomers() is already written. This function opens the input file, customers.txt, for reading. An empty dictionary is created. The dictionary will map a customer’s number to a customer object. As each line of the input file is read in as a string, the string is split using the comma delimiter. This returns a list. The first item in the resulting list is the customer number and the second item is the customer name. The customer object is created by calling the Customer class’ constructor (init) method using these values. An entry is made into the dictionary using the customer number as the key and the Customer object as the value (you’ll have to modify this to reflect your class name). Finally, the dictionary is returned. Function readOrders() You are creating the readOrders() function. This function takes as its parameter the dictionary filled with customer number/customer object key/value pairs. This function should open the input file, orders.txt, for reading. It should then loop through the file in a similar way as the readCustomers() function, as the values are also delimited by commas. Get the customer object from the dictionary. This can be done using the dictionary get() method and sending the customer number. The get method can take a second argument that is returned if the customer number is not a key in the dictionary. If the customer exists in the dictionary, create a tuple consisting of the date (a string), the product code (a string), a product name (a string), the quantity (an int), and the order total (a float). Add this tuple to the customer’s list of orders by sending it to the customer’s addOrder() method.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 5 images

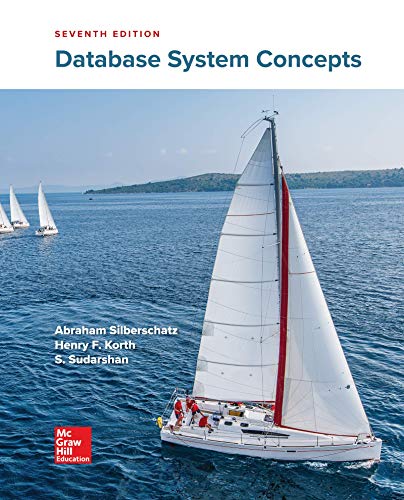
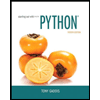
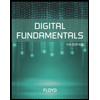
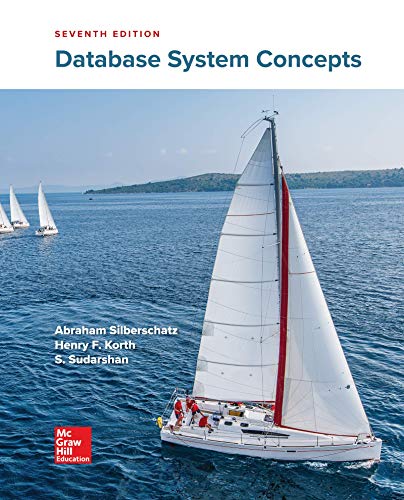
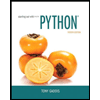
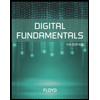
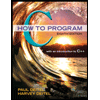
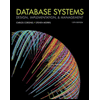
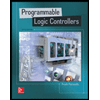