Here are The files that mentioned on the picture : //EarthObject.h - class declaration for the movement of an object on the earth /* This header file defines the structure of the `EarthObject` class. It declares the member variables needed to simulate an object's motion under Earth's gravity, such as gravitational acceleration constant, initial height, horizontal speed, and positional coordinates (x and y). Additionally, it declares constructors (default and parameterized) and member functions (setters and getters) necessary for the class's functionality. */ //TODO: Create a class called EarthObject And //EarthObject.cpp - function definitions for the movement of an object on the earth /* The implementation file for the `EarthObject` class. It defines the functionality of the constructors, setters, and getters declared in the header file. Setters include validation checks for input values, and getters calculate the x and y positions of the object over time based on the physics of motion under Earth's gravitational influence. */ #include "EarthObject.h" //TODO: Add your implementation here //MoonObject.h - class declaration for the movement of an object on the moon /* this header file defines the `MoonObject` class, tailored to simulate motion on the Moon. It includes additional properties, such as the initial vertical speed, to account for differences in lunar gravity. The file declares constructors, member variables, and member functions specific to lunar conditions. */ //TODO: Create a class called MoonObject And //MoonObject.cpp - function definitions for the movement of an object on the Moon. /* This is the implementation file for the `MoonObject` class. It provides the definitions for the class's constructors, setters (with validation), and getters. The functionalities are adjusted to reflect the Moon's lower gravitational acceleration and the possibility of an initial vertical speed. */ #include "MoonObject.h" //TODO: Add your implementation here And //ObjectMain.cpp - main program for moving objects on the moon and on earth #include #include "MoonObject.h" #include "EarthObject.h" using namespace std; int main(void) { double initialHeightEarth = 100; double horizontalSpeedEarth = 100; double initialHeightMoon = 100; double initialVerticalSpeedMoon = 10; double horizontalSpeedMoon = 100; MoonObject moon(initialHeightMoon, initialVerticalSpeedMoon, horizontalSpeedMoon); EarthObject earth(initialHeightEarth, horizontalSpeedEarth); double time; for (time = 0.0; moon.getYPos(time) > 0 && earth.getYPos(time) > 0; time += 0.001) {} cout << "time:" << time << endl; cout << "The moon object's horizontal position is " << moon.getXPos(time) << "m and vertical position is " << moon.getYPos(time) << "m" << endl; cout << "The earth object's horizontal position is " << earth.getXPos(time) << "m and vertical position is " << earth.getYPos(time) << "m" << endl << endl; bool valid; //TODO: Prompt the user to enter new parameters for the moon object and the earth object. // For the moon, the parameters are: initial height, initial vertical speed, // and the horizontal speed. // For the earth, the parameters are: initial height and the horizontal speed. //Be sure to perform validation on the entered values. //The simulation runs again for (time = 0.0; moon.getYPos(time) > 0 && earth.getYPos(time) > 0; time += 0.001) {} cout << endl; cout << "time:" << time << endl; cout << "The moon object's horizontal position is " << moon.getXPos(time) << "m and vertical position is " << moon.getYPos(time) << "m" << endl; cout << "The earth object's horizontal position is " << earth.getXPos(time) << "m and vertical position is " << earth.getYPos(time) << "m" << endl; return 0; }
Here are The files that mentioned on the picture : //EarthObject.h - class declaration for the movement of an object on the earth /* This header file defines the structure of the `EarthObject` class. It declares the member variables needed to simulate an object's motion under Earth's gravity, such as gravitational acceleration constant, initial height, horizontal speed, and positional coordinates (x and y). Additionally, it declares constructors (default and parameterized) and member functions (setters and getters) necessary for the class's functionality. */ //TODO: Create a class called EarthObject And //EarthObject.cpp - function definitions for the movement of an object on the earth /* The implementation file for the `EarthObject` class. It defines the functionality of the constructors, setters, and getters declared in the header file. Setters include validation checks for input values, and getters calculate the x and y positions of the object over time based on the physics of motion under Earth's gravitational influence. */ #include "EarthObject.h" //TODO: Add your implementation here //MoonObject.h - class declaration for the movement of an object on the moon /* this header file defines the `MoonObject` class, tailored to simulate motion on the Moon. It includes additional properties, such as the initial vertical speed, to account for differences in lunar gravity. The file declares constructors, member variables, and member functions specific to lunar conditions. */ //TODO: Create a class called MoonObject And //MoonObject.cpp - function definitions for the movement of an object on the Moon. /* This is the implementation file for the `MoonObject` class. It provides the definitions for the class's constructors, setters (with validation), and getters. The functionalities are adjusted to reflect the Moon's lower gravitational acceleration and the possibility of an initial vertical speed. */ #include "MoonObject.h" //TODO: Add your implementation here And //ObjectMain.cpp - main program for moving objects on the moon and on earth #include #include "MoonObject.h" #include "EarthObject.h" using namespace std; int main(void) { double initialHeightEarth = 100; double horizontalSpeedEarth = 100; double initialHeightMoon = 100; double initialVerticalSpeedMoon = 10; double horizontalSpeedMoon = 100; MoonObject moon(initialHeightMoon, initialVerticalSpeedMoon, horizontalSpeedMoon); EarthObject earth(initialHeightEarth, horizontalSpeedEarth); double time; for (time = 0.0; moon.getYPos(time) > 0 && earth.getYPos(time) > 0; time += 0.001) {} cout << "time:" << time << endl; cout << "The moon object's horizontal position is " << moon.getXPos(time) << "m and vertical position is " << moon.getYPos(time) << "m" << endl; cout << "The earth object's horizontal position is " << earth.getXPos(time) << "m and vertical position is " << earth.getYPos(time) << "m" << endl << endl; bool valid; //TODO: Prompt the user to enter new parameters for the moon object and the earth object. // For the moon, the parameters are: initial height, initial vertical speed, // and the horizontal speed. // For the earth, the parameters are: initial height and the horizontal speed. //Be sure to perform validation on the entered values. //The simulation runs again for (time = 0.0; moon.getYPos(time) > 0 && earth.getYPos(time) > 0; time += 0.001) {} cout << endl; cout << "time:" << time << endl; cout << "The moon object's horizontal position is " << moon.getXPos(time) << "m and vertical position is " << moon.getYPos(time) << "m" << endl; cout << "The earth object's horizontal position is " << earth.getXPos(time) << "m and vertical position is " << earth.getYPos(time) << "m" << endl; return 0; }
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter11: More Object-oriented Programming Concepts
Section: Chapter Questions
Problem 1GZ
Related questions
Question
100%
Here are The files that mentioned on the picture :
//EarthObject.h - class declaration for the movement of an object on the earth
/*
This header file defines the structure of the `EarthObject` class.
It declares the member variables needed to simulate an object's motion
under Earth's gravity, such as gravitational acceleration constant,
initial height, horizontal speed, and positional coordinates (x and y).
Additionally, it declares constructors (default and parameterized)
and member functions (setters and getters) necessary for the class's functionality.
*/
//TODO: Create a class called EarthObject
And
//EarthObject.cpp - function definitions for the movement of an object on the earth
/*
The implementation file for the `EarthObject` class. It defines the
functionality of the constructors, setters, and getters declared in the header file.
Setters include validation checks for input values, and
getters calculate the x and y positions of the object over time
based on the physics of motion under Earth's gravitational influence.
*/
#include "EarthObject.h"
//TODO: Add your implementation here
//MoonObject.h - class declaration for the movement of an object on the moon
/*
this header file defines the `MoonObject` class,
tailored to simulate motion on the Moon.
It includes additional properties, such as the initial vertical speed,
to account for differences in lunar gravity. The file declares constructors,
member variables, and member functions specific to lunar conditions.
*/
//TODO: Create a class called MoonObject
And
//MoonObject.cpp - function definitions for the movement of an object on the Moon.
/*
This is the implementation file for the `MoonObject` class.
It provides the definitions for the class's constructors,
setters (with validation), and getters. The functionalities are adjusted
to reflect the Moon's lower gravitational acceleration and
the possibility of an initial vertical speed.
*/
#include "MoonObject.h"
//TODO: Add your implementation here
And
//ObjectMain.cpp - main program for moving objects on the moon and on earth
#include
#include "MoonObject.h"
#include "EarthObject.h"
using namespace std;
int main(void) {
double initialHeightEarth = 100;
double horizontalSpeedEarth = 100;
double initialHeightMoon = 100;
double initialVerticalSpeedMoon = 10;
double horizontalSpeedMoon = 100;
MoonObject moon(initialHeightMoon, initialVerticalSpeedMoon, horizontalSpeedMoon);
EarthObject earth(initialHeightEarth, horizontalSpeedEarth);
double time;
for (time = 0.0; moon.getYPos(time) > 0 && earth.getYPos(time) > 0; time += 0.001) {}
cout << "time:" << time << endl;
cout << "The moon object's horizontal position is " << moon.getXPos(time) << "m and vertical position is " << moon.getYPos(time) << "m" << endl;
cout << "The earth object's horizontal position is " << earth.getXPos(time) << "m and vertical position is " << earth.getYPos(time) << "m" << endl << endl;
bool valid;
//TODO: Prompt the user to enter new parameters for the moon object and the earth object.
// For the moon, the parameters are: initial height, initial vertical speed,
// and the horizontal speed.
// For the earth, the parameters are: initial height and the horizontal speed.
//Be sure to perform validation on the entered values.
//The simulation runs again
for (time = 0.0; moon.getYPos(time) > 0 && earth.getYPos(time) > 0; time += 0.001) {}
cout << endl;
cout << "time:" << time << endl;
cout << "The moon object's horizontal position is " << moon.getXPos(time) << "m and vertical position is " << moon.getYPos(time) << "m" << endl;
cout << "The earth object's horizontal position is " << earth.getXPos(time) << "m and vertical position is " << earth.getYPos(time) << "m" << endl;
return 0;
}

Transcribed Image Text:Blame 246 lines (163 loc)
review Code
• Validation of Entered Values:
18.9 KB
o Ensure that all entered values are valid (greater than zero) using the SetInitialHeight,
SetInitialVerticalSpeed, and SetHorizontalSpeed methods of the respective classes.
o Use conditional statements to check the return value of these setter methods, If any setter returns
false, prompt the user to re-enter the value.
Additional
• Updating Object Parameters:
informations:
o Once valid values are entered, update the parameters of the moon and earth objects with these new
values.
• Rerun the Simulation:
o After updating the parameters, rerun the simulation loop to calculate the new positions of the Moon
and Earth objects over time.
o Display the results (final time, horizontal and vertical positions) to the user.
Additional Tips for Students
• Make sure to understand the flow of the existing code before adding new code.
• Pay close attention to how values are validated and used in the simulation.
• Test the program with different inputs to ensure that the validation works correctly and the simulation
produces expected results.
Documentation:
• Make sure to document your code, explaining the purpose and functionality of each segment. Proper
commenting aids in maintenance and future modifications. Once your solution passes all tests and you
have documented it, you are ready to finalize and submit!
• Document your code at the beginning with the file name, your name, and student number.
• Add comments throughout the code where necessary to explain your logic.
→Resources:
• Sample code that might assist you with this lab can be found at: Resistor.h, Inductor.h, Resistor.cpp,
Inductor.cpp and RLCircuit.cpp.
o This sample code simulates a resistor/inductor circuit where the current going through the circuit is
given and the voltage drop across the entire circuit has to be calculated.
Sample Output
A sample run for the first 5 books is as follows:
time:4.516
The moon object's horizontal position is 451.6m and vertical position is 128.59m
The earth object's horizontal position is 451.6m and vertical position is -0.0338257m
Enter the initial height for the moon: 50
Enter the initial vertical speed for the moon: 5
Enter the initial horizontal speed for the moon: 200
Enter the initial height for the earth: 300
Enter the initial horizontal speed for the earth: 150
This how the
output is going to
be:
Reflection
time:7.821
The moon object's horizontal position is 1564.2m and vertical position is 39.406m
The earth object's horizontal position is 1173.15m and vertical position is -0.0292411m

Transcribed Image Text:Complete the Code:
Five files have been provided for you: EarthObject.h, Earth Object.cpp, MoonObject.h, MoonObject.cpp and
ObjectMain.cpp.
• Earth Object.h
o This header file defines the structure of the EarthObject class. It declares the member variables
needed to simulate an object's motion under Earth's gravity, such as gravitational acceleration
constant, initial height, horizontal speed, and positional coordinates (x and y). Additionally, it declares
constructors (default and parameterized) and member functions (setters and getters) necessary for
the class's functionality. Please complete the TODO sections.
• EarthObject.cpp
o The corresponding implementation file for the Earthobject class. It defines the functionality of the
constructors, setters, and getters declared in the header file. Setters include validation checks for input
values, and getters calculate the x and y positions of the object over time based on the physics of
motion under Earth's gravitational influence. Please complete the TODO sections.
● MoonObject.h
o Similar to Earthobject.h, this header file defines the MoonObject class, tailored to simulate motion
on the Moon. It includes additional properties, such as the initial vertical speed, to account for
differences in lunar gravity. The file declares constructors, member variables, and member functions
specific to lunar conditions. Please complete the TODO sections.
• MoonObject.cpp
o This is the implementation file for the MoonObject class. It provides the definitions for the class's
constructors, setters (with validation), and getters. The functionalities are adjusted to reflect the
Moon's lower gravitational acceleration and the possibility of an initial vertical speed. Please complete
the TODO sections.
ObjectMain.cpp
o The main driver file for the entire simulation. It contains the main() function, which integrates the
Earthobject and MoonObject classes. In this file, user inputs are taken to initialize objects
representing the Earth and Moon scenarios, and the objects' displacements are calculated and
displayed over time. The file includes a partially completed main() function with sections marked
TODO, where you are expected to add code to instantiate objects and manage the simulation flow
based on user input. Please complete the TODO sections.
Class Declaration: EarthObject
• Member Variables:
O const double g 9.81; Represents Earth's gravitational acceleration constant (9.81 m/s²). It's a
constant, indicating that this value doesn't change.
O double initialHeight; Stores the initial height from which the object is dropped.
o double horizontalSpeed; Stores the horizontal speed of the object.
o double xPos; Represents the x-coordinate (horizontal position) of the object.
O double ypos;: Represents the y-coordinate (vertical position) of the object.
• Constructors:
o Earthobject(double initialHeight, double horSpeed); A parameterized constructor that initializes
the object with a specific initial height and horizontal speed.
o EarthObject();: A default constructor that initializes the object with default values (zeroes for
numeric properties).
• Member Functions:
O double getXPos (double time) const;: Returns the x-position of the object after a given time.
double getYPos (double time) const;: Returns the y-position of the object after a given time.
o bool SetInitialHeight (double initialHeight); Sets the initial height of the object and returns true
if the height is greater than zero; otherwise returns false.
O
ENG
4D 12:08 PM
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 7 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
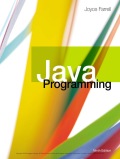
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
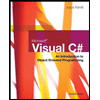
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
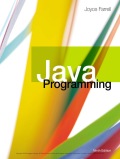
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
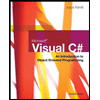
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,