Here is the code I am using. It is almost correct. Can you please help me figure this out please? Below is my code as well as the incorrect output: import random # Functions for generating lottery numbers, counting matches, and simulating plays def generate_lottery_numbers(): """ Generates a list of 5 random integers between 1 and 42, inclusive, with no duplicates. Returns: list: A list of lottery numbers """ return random.sample(range(1, 43), 5) def count_matches(my_list, lottery_list): """ Takes two lists of equal length representing the player’s chosen number list and the generated lottery list and returns the number of matches between my_list and lottery_list. For example, count_matches([10, 6, 20, 5, 7], [30, 6, 7, 40, 5]) will return 3, since both lists contain 5, 6, 7. Parameters: my_list (list): Your lottery numbers. lottery_list (list): A list of the winning numbers. Returns: int: The number of matching integers """ return len(set(my_list) & set(lottery_list)) def play_lottery_once(): """ Uses generate_lottery_numbers() and count_matches() to return the reward gained in playing the lottery one time. The lottery costs $1 to enter. The game award is determined according to the Table derived from data published by the state of Georgia. (If a player wins "one free play", we simply calculate this as winning $1). Returns: int: The total dollar amount gained by playing the game """ player_numbers = generate_lottery_numbers() lottery_numbers = generate_lottery_numbers() matches = count_matches(player_numbers, lottery_numbers) # Determine the reward based on the number of matches if matches == 0: return -1 # Loss, -$1 elif matches == 1: return 0 # Break-even, $0 elif matches == 2: return 0 # No win for 2 matches elif matches == 3: return 5 # Win $5 elif matches == 4: return 20 # Win $20 elif matches == 5: return 100 # Win $100 def sim_many_plays(n): """ Simulates a single person playing the lottery n times, to determine their overall winnings at the end of each simulated lottery. Parameters: n (int): The number of times the person plays the lottery. Returns: list: The total winnings after each of the n lotteries """ winnings = [] for _ in range(n): result = play_lottery_once() if not winnings: winnings.append(result) else: winnings.append(winnings[-1] + result) return winnings if __name__ == "__main__": seed = int(input('Enter a seed for the simulation: ')) random.seed(seed) # Simulate 1000 plays by one person and plot the winnings. winnings = sim_many_plays(1000) print("Total winnings after each lottery:", winnings) Here is the feedback for the parts of my code that are in correct: 9:play_lottery_once(), num matches is 1 Tests play_lottery_once() returns val when number of matches is 1 Test feedback play_lottery_once() returned 0 when 1 number matches in the lottery 11:play_lottery_once(), num matches is 3 Tests play_lottery_once() returns val when number of matches is 3 Test feedback play_lottery_once() returned 5 when 3 numbers match in the lottery 12:play_lottery_once(), num matches is 4 Tests play_lottery_once() returns val when number of matches is 4 Test feedback play_lottery_once() returned 20 when 4 numbers match in the lottery 13:play_lottery_once(), num matches is 5 Tests play_lottery_once() returns val when number of matches is 5 Test feedback play_lottery_once() returned 100 when 5 numbers match in the lottery All of the correct answers say "Your test produced no output." I have tried multiple times to have this question answered; however, each answer produces the same outputs. Really hope you can help me.
Here is the code I am using. It is almost correct. Can you please help me figure this out please?
Below is my code as well as the incorrect output:
import random
# Functions for generating lottery numbers, counting matches, and simulating plays
def generate_lottery_numbers():
""" Generates a list of 5 random integers between 1 and 42, inclusive,
with no duplicates.
Returns:
list: A list of lottery numbers
"""
return random.sample(range(1, 43), 5)
def count_matches(my_list, lottery_list):
""" Takes two lists of equal length representing the player’s chosen number
list and the generated lottery list and returns the number of matches between
my_list and lottery_list.
For example, count_matches([10, 6, 20, 5, 7], [30, 6, 7, 40, 5]) will
return 3, since both lists contain 5, 6, 7.
Parameters:
my_list (list): Your lottery numbers.
lottery_list (list): A list of the winning numbers.
Returns:
int: The number of matching integers
"""
return len(set(my_list) & set(lottery_list))
def play_lottery_once():
""" Uses generate_lottery_numbers() and count_matches() to return the
reward gained in playing the lottery one time. The lottery costs $1 to enter.
The game award is determined according to the Table derived from data published
by the state of Georgia. (If a player wins "one free play", we simply
calculate this as winning $1).
Returns:
int: The total dollar amount gained by playing the game
"""
player_numbers = generate_lottery_numbers()
lottery_numbers = generate_lottery_numbers()
matches = count_matches(player_numbers, lottery_numbers)
# Determine the reward based on the number of matches
if matches == 0:
return -1 # Loss, -$1
elif matches == 1:
return 0 # Break-even, $0
elif matches == 2:
return 0 # No win for 2 matches
elif matches == 3:
return 5 # Win $5
elif matches == 4:
return 20 # Win $20
elif matches == 5:
return 100 # Win $100
def sim_many_plays(n):
""" Simulates a single person playing the lottery n times, to determine
their overall winnings at the end of each simulated lottery.
Parameters:
n (int): The number of times the person plays the lottery.
Returns:
list: The total winnings after each of the n lotteries
"""
winnings = []
for _ in range(n):
result = play_lottery_once()
if not winnings:
winnings.append(result)
else:
winnings.append(winnings[-1] + result)
return winnings
if __name__ == "__main__":
seed = int(input('Enter a seed for the simulation: '))
random.seed(seed)
# Simulate 1000 plays by one person and plot the winnings.
winnings = sim_many_plays(1000)
print("Total winnings after each lottery:", winnings)
Here is the feedback for the parts of my code that are in correct:
9:play_lottery_once(), num matches is 1
Tests play_lottery_once() returns val when number of matches is 1
Test feedback
play_lottery_once() returned 0 when 1 number matches in the lottery
11:play_lottery_once(), num matches is 3
Tests play_lottery_once() returns val when number of matches is 3
Test feedback
play_lottery_once() returned 5 when 3 numbers match in the lottery
12:play_lottery_once(), num matches is 4
Tests play_lottery_once() returns val when number of matches is 4
Test feedback
play_lottery_once() returned 20 when 4 numbers match in the lottery
13:play_lottery_once(), num matches is 5
Tests play_lottery_once() returns val when number of matches is 5
Test feedback
play_lottery_once() returned 100 when 5 numbers match in the lottery
All of the correct answers say "Your test produced no output."
I have tried multiple times to have this question answered; however, each answer produces the same outputs. Really hope you can help me.

Step by step
Solved in 3 steps with 1 images

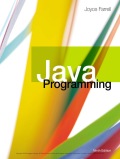
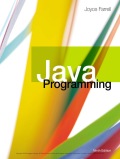