I need to combine these programs into one with a menu system that looks like this: Menu ==== 1. Play Rock-Paper-Scissors 2. Play Yahtzee 3. Exit Program #1 #include #include #include using namespace std; int main() { unsigned seed = time(0); srand(seed); int maxWins = 0, player1Wins = 0, player2Wins = 0; while (maxWins % 2 == 0) { cout << "Enter an odd number: "; cin >> maxWins; } int count = 1; while (player1Wins < maxWins && player2Wins < maxWins) { int player1 = rand() % 3; int player2 = rand() % 3; string player1Choice, player2Choice; if (player1 == 0) { player1Choice = "rock"; } else if (player1 == 1) { player1Choice = "scissors"; } else { player1Choice = "paper"; } if (player2 == 0) { player2Choice = "rock"; } else if (player2 == 1) { player2Choice = "scissors"; } else { player2Choice = "paper"; } cout << "game #" << count << endl; cout << "player1 : " << player1Choice << endl; cout << "player2 : " << player2Choice << endl; if (player1Choice == "rock") { if (player2Choice == "scissors") { player1Wins++; } else if (player2Choice == "paper") { player2Wins++; } } else if (player1Choice == "scissors") { if (player2Choice == "rock") { player2Wins++; } else if (player2Choice == "paper") { player1Wins++; } } else if (player1Choice == "paper") { if (player2Choice == "rock") { player1Wins++; } else if (player2Choice == "scissors") { player2Wins++; } } cout << "player1 = " << player1Wins << ", player2 = " << player2Wins << endl; count++; } if (player1Wins > player2Wins) { cout << "PLAYER1 WINS THE MATCH" << endl; } else { cout << "PLAYER2 WINS THE MATCH" << endl; } return 0; } Program #2 #include #include #include using namespace std; int main() { unsigned seed = time(0); srand(seed); int i = 1; while (true) { int dice1 = rand() % 6 + 1; int dice2 = rand() % 6 + 1; int dice3 = rand() % 6 + 1; int dice4 = rand() % 6 + 1; int dice5 = rand() % 6 + 1; cout << "Roll " << i << " : " << dice1 << " " << dice2 << " " << dice3 << " " << dice4 << " " << dice5; if (dice2 != dice1 && dice3 != dice1 && dice3 != dice2 && dice4 != dice3 && dice4 != dice2 && dice4 != dice1 && dice5 != dice4 && dice5 != dice3 && dice5 != dice2 && dice5 != dice1) { cout << " No matches this time!"; } else if (dice1 == dice2 && dice2 == dice3 && dice3 == dice4 && dice4 == dice5) { cout << "\nYAHTZEE" << endl; return 0; } cout << endl; i++; } return 0; }
I need to combine these programs into one with a menu system that looks like this:
Menu
====
1. Play Rock-Paper-Scissors
2. Play Yahtzee
3. Exit
Program #1
#include<iostream>
#include<cstdlib>
#include<ctime>
using namespace std;
int main()
{
unsigned seed = time(0);
srand(seed);
int maxWins = 0, player1Wins = 0, player2Wins = 0;
while (maxWins % 2 == 0) {
cout << "Enter an odd number: ";
cin >> maxWins;
}
int count = 1;
while (player1Wins < maxWins && player2Wins < maxWins) {
int player1 = rand() % 3;
int player2 = rand() % 3;
string player1Choice, player2Choice;
if (player1 == 0) {
player1Choice = "rock";
}
else if (player1 == 1) {
player1Choice = "scissors";
}
else {
player1Choice = "paper";
}
if (player2 == 0) {
player2Choice = "rock";
}
else if (player2 == 1) {
player2Choice = "scissors";
}
else {
player2Choice = "paper";
}
cout << "game #" << count << endl;
cout << "player1 : " << player1Choice << endl;
cout << "player2 : " << player2Choice << endl;
if (player1Choice == "rock") {
if (player2Choice == "scissors") {
player1Wins++;
}
else if (player2Choice == "paper") {
player2Wins++;
}
}
else if (player1Choice == "scissors") {
if (player2Choice == "rock") {
player2Wins++;
}
else if (player2Choice == "paper") {
player1Wins++;
}
}
else if (player1Choice == "paper") {
if (player2Choice == "rock") {
player1Wins++;
}
else if (player2Choice == "scissors") {
player2Wins++;
}
}
cout << "player1 = " << player1Wins << ", player2 = " << player2Wins << endl;
count++;
}
if (player1Wins > player2Wins) {
cout << "PLAYER1 WINS THE MATCH" << endl;
}
else {
cout << "PLAYER2 WINS THE MATCH" << endl;
}
return 0;
}
Program #2
#include<iostream>
#include<cstdlib>
#include<ctime>
using namespace std;
int main()
{
unsigned seed = time(0);
srand(seed);
int i = 1;
while (true) {
int dice1 = rand() % 6 + 1;
int dice2 = rand() % 6 + 1;
int dice3 = rand() % 6 + 1;
int dice4 = rand() % 6 + 1;
int dice5 = rand() % 6 + 1;
cout << "Roll " << i << " : " << dice1 << " " << dice2 << " " << dice3 << " " << dice4 << " " << dice5;
if (dice2 != dice1 && dice3 != dice1 && dice3 != dice2 && dice4 != dice3 && dice4 != dice2 && dice4 != dice1 && dice5 != dice4 && dice5 != dice3 && dice5 != dice2 && dice5 != dice1) {
cout << " No matches this time!";
}
else if (dice1 == dice2 && dice2 == dice3 && dice3 == dice4 && dice4 == dice5) {
cout << "\nYAHTZEE" << endl;
return 0;
}
cout << endl;
i++;
}
return 0;
}

Step by step
Solved in 3 steps with 9 images

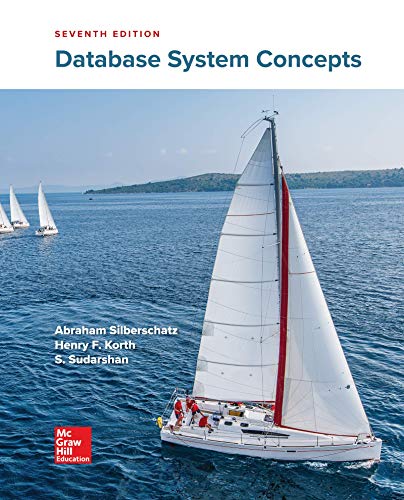
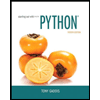
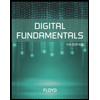
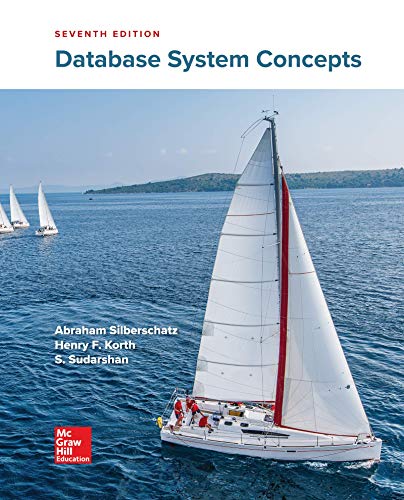
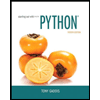
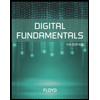
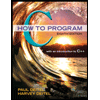
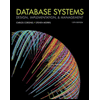
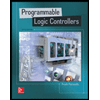