In C programming. How do I find the max and min values of the corressponding columns.
In C programming. How do I find the max and min values of the corressponding columns.
#include <stdio.h>
#include <math.h>
#include <stdlib.h>
#define NROWS 5
#define NCOLS 5
int main(void)
{
int i,j, max, min, m, n;
float M[NROWS][NCOLS], Mt[NROWS][NCOLS];
char key_hit;
//Input the sizes
printf("ROW size(1-5) : ");scanf("%d",&m);
printf("Column size(1-5): ");scanf("%d",&n);
//Size Check
while(m>NROWS || n>NCOLS)
{
printf("Please re-enter the sizes. \n");
printf("Row size(1-5) : ");scanf("%d", &m);
printf("Column size(1-5) : ");scanf("%d", &n);
}
//Input the Matrix Data
for(i=0; i<m; i++)
for(j=0; j<n; j++)
{
printf("M[%d][%d] = ", i,j);
scanf("%f", &M[i][j]);
}
//Print the Matrix Data
for(i=0; i<m; i++)
{
for(j=0; j<n; j++)
{
printf("%1.2f\t", M[i][j]);
}
printf("\n");
}
//Choices of matrix operations
do{
printf("\n\nIs there anything choice you would like to do?");
printf("\nThe numbers related to the choices are listed below:");
printf("\n1. Display The matrix M.");
printf("\n2. Display The Matrix and its transpose.");
printf("\n3. Max Values of each column.");
printf("\n4. End the program.");
printf("\nPlease enter your choice (the number): ");
scanf(" %c", &key_hit);
key_hit == '0';
//Option 1. Display Matrix M
if (key_hit == '1')
{
printf("M = ");
for(i=0; i<m; i++)
{
for(j=0; j<n; j++)
{
printf("%.2f\t", M[i][j]);
}
printf("\n\t");
}
}
// Transpose of Matrix M
if (key_hit == '2')
{
printf("M^t = ");
for(i=0; i<m; i++)
{
for(j=0; j<n; j++)
{
Mt[i][j] = M[j][i];
printf("%.2f\t", Mt[i][j]);
}
printf("\n\t");
}
}
//Min and Max values of Columns
if(key_hit == '3')
{
min = M[1][1];
max = M[1][1];
for(i=1; i<m; i++)
for(j=1; j<n; j++)
{
if(M[i][j] < min)
{
min = M[j];
}
if(M[i][j] > max)
{
max = M[j];
}
}
printf("\nThe max column value entry is: %i", max);
printf("\nThe min column value entry is: %i", min);
}
}while(key_hit!='4');
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

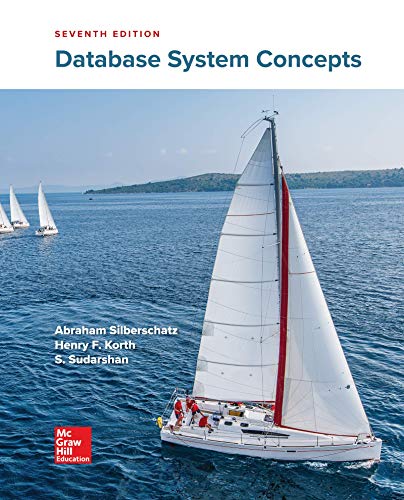
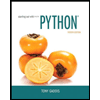
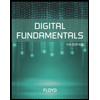
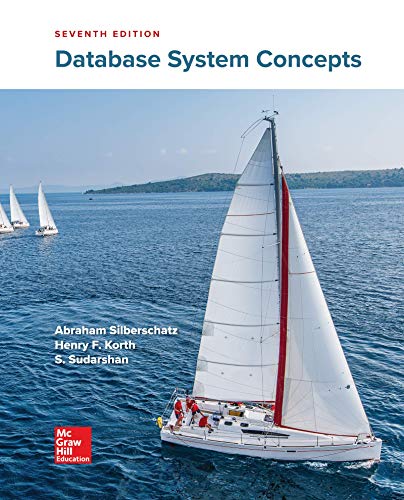
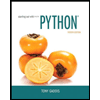
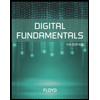
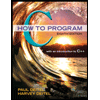
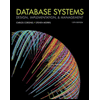
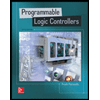