In the previous assignment, you wrote a program to print the calendar for one month in a particular year. You will find a sample solution on Moodle in two versions: a2_v1.cpp uses the % operator for positioning the cursor on the appropriate column, and a2_v2.cpp resets the cursor each time it reaches 7. Please use one of these versions to do the following: In this assignment, you have to print the complete 12-month calendar for a particular year. The user will be asked to enter the year, as well as the day of the week for the 1st of January. The program will then have a loop that executes 12 times, each time calling a function that prints one month. At the end, the program prints the day of the week when the year ends. Note that the day of the week for the first of a particular month will be one following the last day of the week for the previous month. So the complete main() will be like: int main() { cout << "which year? "; int year; } // needed to decide whether it's a leap year (for February). cin >> year; cout << "enter the day of the week for the 1st of January (1=Sun, 2-Mon etc) "; int first; cin >> first; for (int month= 1; month <= 12; month++) draw_month (first, month, year); In addition, the tasks of: Finding the maximum days in a particular month of a particular year Finding the name of a month given the month number Finding the name of the day of the week given the day number are each performed by a separate function, which will be called from within the draw_month function. To help you, here are the signatures for all the functions you need: int find_max_days (int month, int year); string month_name (int month); string day_of_week (int pos); void draw_month (int &first, int month, int year); 1
Can you do the question using the program below which is a2_v1.cpp? Thank you very much
#include <iostream>
#include <iomanip>
#include <conio.h>
using namespace std;
int main(){
cout << "Which month would you like to print (1=Jan, 2=Feb etc..)? ";
int month; // needed to calculate the maximum days in that month
cin >> month;
cout << "which year? ";
int year; // needed to decide whether it's a leap year (for February)
cin >> year;
cout << "enter the day of the week for the 1st of the month (1=Sun, 2=Mon etc) ";
int first;
cin >> first;
// find the number of days in that particular month
int max_days;
switch (month){
case 1: case 3: case 5: case 7: case 8: case 10: case 12: max_days = 31; break;
case 4: case 6: case 9: case 11: max_days = 30; break;
case 2: if (year % 4 != 0 || year%100==0 && year%400!=0)
max_days = 28;
else max_days = 29; break;
default: break;
}
// find the name of the month
string mth;
switch (month){
case 1: mth = "January"; break;
case 2: mth = "February"; break;
case 3: mth = "March"; break;
case 4: mth = "April"; break;
case 5: mth = "May"; break;
case 6: mth = "June"; break;
case 7: mth = "July"; break;
case 8: mth = "August"; break;
case 9: mth = "September"; break;
case 10: mth = "October"; break;
case 11: mth = "November"; break;
case 12: mth = "December"; break;
}
// print a heading
cout << "\n Calendar for month " << mth << " of "<< year <<endl<<endl;
cout << " Sun Mon Tue Wed Thu Fri Sat\n";
// Print the whole month
int day = 1;
cout << setw (first*4) << day; // position the "1" under the right day of the week
int pos = first; // used to place the number in the correct column
for (day = 2; day <= max_days; day++){ // loop to print all the days
if (pos % 7 == 0) // go to a new line at the end of the week
cout << endl;
cout << setw(4) << day;
pos++;
}
// print the last weekday of the month
cout <<"\n\nThe month ends on a ";
switch (pos%7){
case 1: cout << "Sunday"; break;
case 2: cout << "Monday"; break;
case 3: cout << "Tuesday"; break;
case 4: cout << "Wednesday"; break;
case 5: cout << "Thursday"; break;
case 6: cout << "Friday"; break;
case 0: cout << "Saturday"; break;
}
getch();
}


Step by step
Solved in 3 steps with 8 images

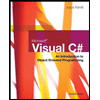
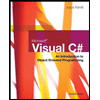