#include "Skybox.h" /* #define BMP NEG_X_FILE_PATH "../../Models/Lab8/BMP/NegX.bmp" #define BMP NEG_Y_FILE_PATH "../../Models/Lab8/BMP/NegY.bmp" #define BMP NEG_Z_FILE_PATH "../../Models/Lab8/BMP/NegZ.bmp" #define BMP_POS_X_FILE_PATH "../../Models/Lab8/BMP/PosX.bmp" #define BMP_POS_Y_FILE_PATH"../../Models/Lab8/BMP/PosY.bmp" #define BMP_POS_Z_FILE_PATH "../../Models/Lab8/BMP/PosZ.bmp" #define BMP_POS_Y_FILE_PATH"../../Models/solar_system/spacebox/spacebox_bottom.bmp" #define BMP_POS_X_FILE_PATH"../../Models/solar_system/spacebox/spacebox_east.bmp" #define BMP_POS_Z_FILE_PATH "../../Models/solar_system/spacebox/spacebox_north.bmp" #define BMP_NEG_Z_FILE_PATH "../../Models/solar_system/spacebox/spacebox_south.bmp" #define BMP_NEG_Y_FILE_PATH "../../MODELS/solar_system/spacebox/spacebox_top.bmp" #define BMP_NEG_X_FILE_PATH "../../Models/solar_system/spacebox/spacebox_west.bmp" #define BMP_NEG_X_FILE_PATH"../../Models/Lab8/skybox/xneg.bmp" #define BMP_POS_X_FILE_PATH"../../Models/Lab8/skybox/xpos.bmp" #define BMP NEG_Y_FILE_PATH"../../Models/Lab8/skybox/yneg.bmp" #define BMP_POS_Y_FILE_PATH "../../Models/Lab8/skybox/ypos.bmp" #define BMP NEG_Z_FILE_PATH "../../Models/Lab8/skybox/zneg.bmp" #define BMP_POS_Z_FILE_PATH"../../Models/Lab8/skybox/zpos.bmp" */ #define BMP_NEG_X_FILE_PATH"../../Models/Lab8/skybox_compass/xneg.bmp" #define BMP_POS_X_FILE_PATH"../../Models/Lab8/skybox_compass/xpos.bmp" #define BMP NEG_Y_FILE_PATH "../../Models/Lab8/skybox_compass/yneg.bmp" #define BMP_POS_Y_FILE_PATH "../../Models/Lab8/skybox_compass/ypos.bmp" #define BMP NEG_Z_FILE_PATH"../../Models/Lab8/skybox_compass/zneg.bmp" #define BMP_POS_Z_FILE_PATH "../../Models/Lab8/skybox_compass/zpos.bmp" Skybox::Skybox (Shader* shader) Model(shader) { } Just call the parent constructor Skybox::~Skybox() { } void Skybox::upload_CubeMap_images() { unsigned char* temp_image_data; int width, height, size; 1) Enable cube mapping glEnable(GL_TEXTURE_CUBE_MAP); // 4) Load all six images into the correct "place" in the cube map. Look in the Resources folders for the names. Make sure you delete the memory you used between each call. // load_bmp_file(BMP_NEG_X_FILE_PATH, &width, &height, &size, &temp_image_data); glTexImage2D(GL_TEXTURE_CUBE_MAP_NEGATIVE_X, 0, GL_RGB, width, height, 0, GL_BGR, GL_UNSIGNED_BYTE, temp_image_data); delete (temp_image_data); load_bmp_file(BMP_NEG_Y_FILE_PATH, &width, &height, &size, &temp_image_data); g1TexImage2D(GL_TEXTURE_CUBE_MAP_NEGATIVE_Y, 0, GL_RGB, width, height, 0, GL_BGR, GL_UNSIGNED_BYTE, temp_image_data); delete (temp_image_data); load_bmp_file(BMP_NEG_Z_FILE_PATH, &width, &height, &size, &temp_image_data); glTexImage2D(GL_TEXTURE_CUBE_MAP_NEGATIVE_Z, 0, GL_RGB, width, height, 0, GL_BGR, GL_UNSIGNED_BYTE, temp_image_data); delete (temp_image_data); load_bmp_file(BMP_POS_X_FILE_PATH, &width, &height, &size, &temp_image_data); glTexImage2D(GL_TEXTURE_CUBE_MAP_POSITIVE_X, 0, GL_RGB, width, height, 0, GL_BGR, GL_UNSIGNED_BYTE, temp_image_data); delete (temp_image_data); load_bmp_file(BMP_POS_Y_FILE_PATH, &width, &height, &size, &temp_image_data); glTexImage2D(GL_TEXTURE_CUBE_MAP_POSITIVE_Y, 0, GL_RGB, width, height, 0, GL_BGR, GL_UNSIGNED_BYTE, temp_image_data); delete (temp_image_data); load_bmp_file(BMP_POS_Z_FILE_PATH, &width, &height, &size, &temp_image_data); g1TexImage2D(GL_TEXTURE_CUBE_MAP_POSITIVE_Z, 0, GL_RGB, width, height, 0, GL_BGR, GL_UNSIGNED_BYTE, temp_image_data); delete (temp_image_data); // 5) Set the S, T and R parameters for the texture, but make sure you use GL_TEXTURE_CUBE_MAP glTexParameterf (GL_TEXTURE_CUBE_MAP, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameterf (GL_TEXTURE_CUBE_MAP, GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexParameterf (GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE); glTexParameterf (GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE); glTexParameterf(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_R, GL_CLAMP_TO_EDGE); // 6) Get the current ID/location of the "cubeMap" variable in the shader and put it into "cube_map_ID" cube_map_ID = glGetUniformLocation (shader->program_ID, "cubeMap"); // 2) Generate a texture ID to hold the cube map (use tex_buffer_ID, which is inherited from Model; } glGenTextures (1, &tex_buffer_ID); // 3) Bind tex_buffer_ID as the current buffer, but make sure you do it as a cube map! glBindTexture(GL_TEXTURE_CUBE_MAP, tex_buffer_ID); // Bind the current buffer to texture unit 0. This makes the cubeMap variable refer to this texture. glUniform1i(cube_map_ID, 0);
Skybox class (M Visual Studios): Modify the skybox class appropriately to handle cube mapping. This involves loading six images for the cube map, setting appropriate texture parameters, and ensuring the normals of the cube model that encoded and runs sucessfully
Task: The upload_CubeMap_images() function in the Skybox.cpp file needs to be implemented sucessfully according to the instructions.
Instructions:
Enable cube mapping: Use glEnable(GL_TEXTURE_CUBE_MAP) to enable cube mapping.
Generate a texture ID: Use glGenTextures() to generate a texture ID to hold the cube map. This ID is stored in the tex_buffer_ID member variable of the Skybox class.
Bind the texture ID: Use glBindTexture() to bind the texture ID as the current buffer. Make sure to bind it as a cube map using GL_TEXTURE_CUBE_MAP.
Load six images: Load all six images into the correct "place" in the cube map. You can use the provided file paths (BMP_NEG_X_FILE_PATH, BMP_NEG_Y_FILE_PATH, etc.) to load the images.
(Make sure to delete the memory used for each image after uploading it to OpenGL.)
Set texture parameters: Use glTexParameterf() to set the S, T, and R parameters for the texture. Make sure to use GL_TEXTURE_CUBE_MAP as the target.
Get uniform location: Use glGetUniformLocation() to get the current ID/location of the "cubeMap" variable in the shader. Store this location in the cube_map_ID member variable of the Skybox class.
Bind the texture to a texture unit: Use glUniform1i() to bind the current buffer to texture unit 0. This makes the cubeMap variable in the shader refer to this texture.


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

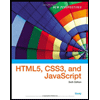
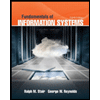
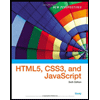
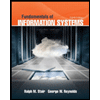