JAVA I am trying to figure out how to connect the PaymentBatchProcessor Class (generic) to the Payment Class(interface). Can you explain the relationship and how I can populate payment.getamount() for my test. Does the paymentBatvhPossser also have to implement the Payment Class ? The other two classes are Check and IOU that implements the payment class. package cop2251.fall23.week4.sullivan; import java.util.ArrayList; import java.util.List; ///PaymentBatchProcessor to work with any of the three types shown above. The PaymentBatchProcessor //class should maintain a list of payments (using an ArrayList), //based upon the type it was instantiated to work with. //a method named add that adds the appropriate payment type to the ArrayList. //a method named getMax() that returns the payment with the largest amount //a method named getTotal() that returns the sum of all the payments //a method named getSize(), that returns how many payments are in the ArrayList. //Aggeration class - batch processor is dependent of payments //1. Define a generic Class T = DataType public class PaymentBatchProcessor { //variable to hold payments private Payment payment; // ArrayList to hold a collections of payments static ArrayList paymentslist = new ArrayList(); //2. Create an ArrayList ListOfPayments type //to maintain a list of payments //Create a perameter to pass the payment to, of Payment type public PaymentBatchProcessor(Payment payment) { this.payment = payment; PaymentBatchProcessor.paymentslist = new ArrayList(); } //A.//add()adds the appropriate payment type to the ArrayList. public static int add(int payment) { return payment; } //B.getMax() getMax() that returns the payment with the largest amount. public static double getMax(double amount) { double getAmount = 0.0; for (Payment payment : paymentslist) { // System.out.println(payment.getAmount()); if (payment.getAmount(getAmount)) > getAmount) { getAmount = payment.getAmount(); } } return getAmount; } //C.getTotal()that returns the sum of all the payments public static int getTotal (int sum) { double totalamount = 0; for (Payment payment : paymentslist) { totalamount += payment.getAmount(totalamount); } return (int) totalamount; } //D.getSize()that returns how many payments are in the ArrayList. public static int getSize(int howManyPayments) { int howManyPayments1 = paymentslist.size(); return howManyPayments1; } public static void main(String[] args) { Payment payment = new Check(25000.63); Payment payment1 = new IOU(3600.56); Payment payment2 = new Check(250000.64); Payment payment3 = new IOU(36000.56); Payment payment4 = new Check(2500.63); Payment payment5 = new IOU(36007.56); PaymentBatchProcessor <> processor = new PaymentBatchProcessor(payment); // add to the list processor.paymentslist.add(payment); processor.paymentslist.add(payment1); processor.paymentslist.add(payment2); processor.paymentslist.add(payment3); processor.paymentslist.add(payment4); processor.paymentslist.add(payment5); System.out.println(processor.paymentslist); double amount = processor.getMax(0); System.out.println("the max payment is" + amount); double total = processor.getTotal(); System.out.println("the total payment is" + total); int size = processor.getSize(); System.out.println("the no of payments are=" + size); } //are these needed for the payment class ? @Override public void setAmount(double amount) { // TODO Auto-generated method stub } //are these needed for the payment class ? @Override public double getAmount(double amount) { // TODO Auto-generated method stub return 0; } }
JAVA I am trying to figure out how to connect the PaymentBatchProcessor Class (generic) to the Payment Class(interface). Can you explain the relationship and how I can populate payment.getamount() for my test. Does the paymentBatvhPossser also have to implement the Payment Class ? The other two classes are Check and IOU that implements the payment class. package cop2251.fall23.week4.sullivan; import java.util.ArrayList; import java.util.List; ///PaymentBatchProcessor to work with any of the three types shown above. The PaymentBatchProcessor //class should maintain a list of payments (using an ArrayList), //based upon the type it was instantiated to work with. //a method named add that adds the appropriate payment type to the ArrayList. //a method named getMax() that returns the payment with the largest amount //a method named getTotal() that returns the sum of all the payments //a method named getSize(), that returns how many payments are in the ArrayList. //Aggeration class - batch processor is dependent of payments //1. Define a generic Class T = DataType public class PaymentBatchProcessor { //variable to hold payments private Payment payment; // ArrayList to hold a collections of payments static ArrayList paymentslist = new ArrayList(); //2. Create an ArrayList ListOfPayments type //to maintain a list of payments //Create a perameter to pass the payment to, of Payment type public PaymentBatchProcessor(Payment payment) { this.payment = payment; PaymentBatchProcessor.paymentslist = new ArrayList(); } //A.//add()adds the appropriate payment type to the ArrayList. public static int add(int payment) { return payment; } //B.getMax() getMax() that returns the payment with the largest amount. public static double getMax(double amount) { double getAmount = 0.0; for (Payment payment : paymentslist) { // System.out.println(payment.getAmount()); if (payment.getAmount(getAmount)) > getAmount) { getAmount = payment.getAmount(); } } return getAmount; } //C.getTotal()that returns the sum of all the payments public static int getTotal (int sum) { double totalamount = 0; for (Payment payment : paymentslist) { totalamount += payment.getAmount(totalamount); } return (int) totalamount; } //D.getSize()that returns how many payments are in the ArrayList. public static int getSize(int howManyPayments) { int howManyPayments1 = paymentslist.size(); return howManyPayments1; } public static void main(String[] args) { Payment payment = new Check(25000.63); Payment payment1 = new IOU(3600.56); Payment payment2 = new Check(250000.64); Payment payment3 = new IOU(36000.56); Payment payment4 = new Check(2500.63); Payment payment5 = new IOU(36007.56); PaymentBatchProcessor <> processor = new PaymentBatchProcessor(payment); // add to the list processor.paymentslist.add(payment); processor.paymentslist.add(payment1); processor.paymentslist.add(payment2); processor.paymentslist.add(payment3); processor.paymentslist.add(payment4); processor.paymentslist.add(payment5); System.out.println(processor.paymentslist); double amount = processor.getMax(0); System.out.println("the max payment is" + amount); double total = processor.getTotal(); System.out.println("the total payment is" + total); int size = processor.getSize(); System.out.println("the no of payments are=" + size); } //are these needed for the payment class ? @Override public void setAmount(double amount) { // TODO Auto-generated method stub } //are these needed for the payment class ? @Override public double getAmount(double amount) { // TODO Auto-generated method stub return 0; } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
JAVA I am trying to figure out how to connect the PaymentBatchProcessor Class (generic) to the Payment Class(interface). Can you explain the relationship and how I can populate payment.getamount() for my test. Does the paymentBatvhPossser also have to implement the Payment Class ? The other two classes are Check and IOU that implements the payment class.
package cop2251.fall23.week4.sullivan;
import java.util.ArrayList;
import java.util.List;
///PaymentBatchProcessor to work with any of the three types shown above. The PaymentBatchProcessor
//class should maintain a list of payments (using an ArrayList),
//based upon the type it was instantiated to work with.
//a method named add that adds the appropriate payment type to the ArrayList.
//a method named getMax() that returns the payment with the largest amount
//a method named getTotal() that returns the sum of all the payments
//a method named getSize(), that returns how many payments are in the ArrayList.
//Aggeration class - batch processor is dependent of payments
//1. Define a generic Class T = DataType
public class PaymentBatchProcessor {
//variable to hold payments
private Payment payment;
// ArrayList to hold a collections of payments
static ArrayList paymentslist = new ArrayList();
//2. Create an ArrayList ListOfPayments type
//to maintain a list of payments
//Create a perameter to pass the payment to, of Payment type
public PaymentBatchProcessor(Payment payment) {
this.payment = payment;
PaymentBatchProcessor.paymentslist = new ArrayList();
}
//A.//add()adds the appropriate payment type to the ArrayList.
public static int add(int payment)
{
return payment;
}
//B.getMax() getMax() that returns the payment with the largest amount.
public static double getMax(double amount) {
double getAmount = 0.0;
for (Payment payment : paymentslist) {
// System.out.println(payment.getAmount());
if (payment.getAmount(getAmount)) > getAmount) {
getAmount = payment.getAmount();
}
}
return getAmount;
}
//C.getTotal()that returns the sum of all the payments
public static int getTotal (int sum)
{
double totalamount = 0;
for (Payment payment : paymentslist) {
totalamount += payment.getAmount(totalamount);
}
return (int) totalamount;
}
//D.getSize()that returns how many payments are in the ArrayList.
public static int getSize(int howManyPayments) {
int howManyPayments1 = paymentslist.size();
return howManyPayments1;
}
public static void main(String[] args) {
Payment payment = new Check(25000.63);
Payment payment1 = new IOU(3600.56);
Payment payment2 = new Check(250000.64);
Payment payment3 = new IOU(36000.56);
Payment payment4 = new Check(2500.63);
Payment payment5 = new IOU(36007.56);
PaymentBatchProcessor <> processor = new PaymentBatchProcessor(payment);
// add to the list
processor.paymentslist.add(payment);
processor.paymentslist.add(payment1);
processor.paymentslist.add(payment2);
processor.paymentslist.add(payment3);
processor.paymentslist.add(payment4);
processor.paymentslist.add(payment5);
System.out.println(processor.paymentslist);
double amount = processor.getMax(0);
System.out.println("the max payment is" + amount);
double total = processor.getTotal();
System.out.println("the total payment is" + total);
int size = processor.getSize();
System.out.println("the no of payments are=" + size);
}
//are these needed for the payment class ?
@Override
public void setAmount(double amount) {
// TODO Auto-generated method stub
}
//are these needed for the payment class ?
@Override
public double getAmount(double amount) {
// TODO Auto-generated method stub
return 0;
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
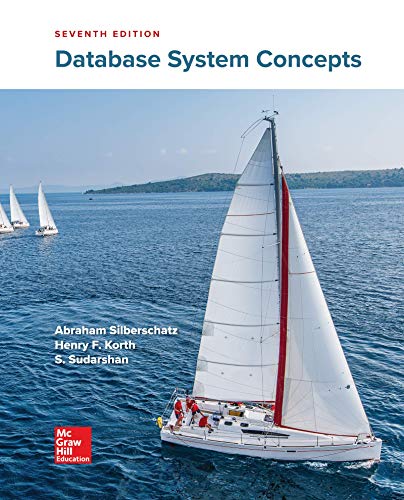
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
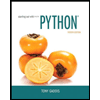
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
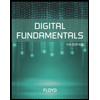
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
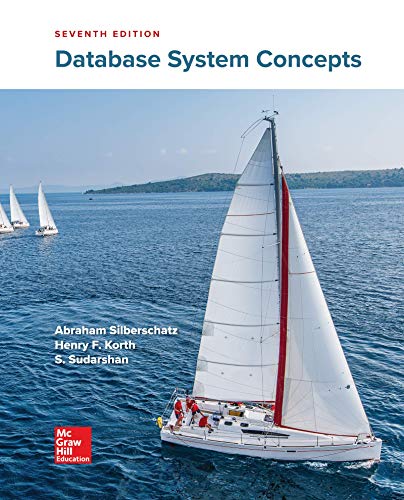
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
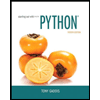
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
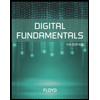
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
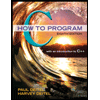
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
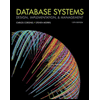
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
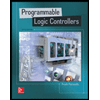
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education