JAVA Problem description: You are to write a program called Seasons that prompts the user for a month and day and displays the season in which that date occurs. For reference, we will use the following date cutoffs for the various seasons: Winter December 21 – March 19 Spring March 20th – June 20th Summer June 21st – September 20th Fall September 21st – December 20th Data Validation For this program, you'll write a specific method to perform data validation based on the month and day entered. More specifically: Month – the month entered must be a valid month of the year (January – December). Day – the day entered must be a valid day for that month: January, March, May, July, August, October, December have 1-31 days. April, June, September, November have 1-30 days. February has 1-28 days (do not worry about leap years) Required program decomposition String getMonth(Scanner console) This method is called from the main method and should prompt for the month as a string and return it to the main method. Recall that you must declare the Scanner object in the main method and pass it as a parameter to the method. int getDay(Scanner console) This method is called from main method and should prompt for the day and return it to main. boolean validInput(String inputMonth, int inputDay) This method is called from main and is passed as parameters the month and day entered by the user. The month value may be a combination of uppercase and lowercase letters. The method should determine whether the month and day entered are valid dates based on ensuring that the month is a valid month and the number of days is a valid number of days for that month. This method returns a boolean true to indicate the month/day are valid and false if either the month or day are invalid. String calcSeason(String inputMonth, int inputDay) This method accepts a valid month and valid day from main and determines in what season the date occurs. The month value may be a combination of uppercase and lowercase letters. This method assumes the month/day value has been verified by the validInput method. Returns a string with one of the following values: "winter", "spring", "summer", "fall". void displaySeason(String season) This method displays the results indicating in what season the date entered by the user occurs. Yes, it will be a very small method with only a print statement.
JAVA
Problem description:
You are to write a program called Seasons that prompts the user for a month and day and displays the season in which that date occurs. For reference, we will use the following date cutoffs for the various seasons:
Winter | December 21 – March 19 |
Spring | March 20th – June 20th |
Summer | June 21st – September 20th |
Fall | September 21st – December 20th |
Data Validation
For this program, you'll write a specific method to perform data validation based on the month and day entered. More specifically:
-
Month – the month entered must be a valid month of the year (January – December).
-
Day – the day entered must be a valid day for that month:
- January, March, May, July, August, October, December have 1-31 days.
- April, June, September, November have 1-30 days.
- February has 1-28 days (do not worry about leap years)
Required program decomposition
String getMonth(Scanner console)
This method is called from the main method and should prompt for the month as a string and return it to the main method. Recall that you must declare the Scanner object in the main method and pass it as a parameter to the method.
int getDay(Scanner console)
This method is called from main method and should prompt for the day and return it to main.
boolean validInput(String inputMonth, int inputDay)
This method is called from main and is passed as parameters the month and day entered by the user. The month value may be a combination of uppercase and lowercase letters. The method should determine whether the month and day entered are valid dates based on ensuring that the month is a valid month and the number of days is a valid number of days for that month. This method returns a boolean true to indicate the month/day are valid and false if either the month or day are invalid.
String calcSeason(String inputMonth, int inputDay)
This method accepts a valid month and valid day from main and determines in what season the date occurs. The month value may be a combination of uppercase and lowercase letters. This method assumes the month/day value has been verified by the validInput method. Returns a string with one of the following values: "winter", "spring", "summer", "fall".
void displaySeason(String season)
This method displays the results indicating in what season the date entered by the user occurs. Yes, it will be a very small method with only a print statement.



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

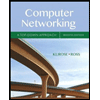
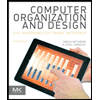
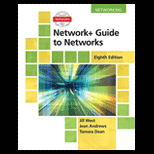
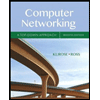
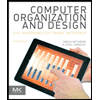
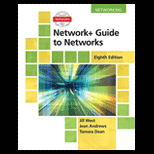
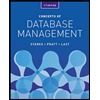
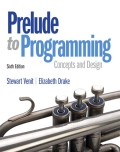
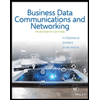