Lab2.c #include #include /* contains fork prototype */ int main(void) { int pid; printf("Hello World!\n"); printf("I am the parent process and pid is : %d .\n",getpid()); printf("Here i am before use of forking\n"); pid = fork(); printf("Here I am just after forking\n"); if (pid == 0) printf("I am the child process and pid is :%d.\n",getpid()); else printf("I am the parent process and pid is: %d .\n",getpid()); } What is the process ID of the child process and parent process? Lab3.c (Multiple forks): #include #include /* contains fork prototype */ void main(void) { printf("Here I am just before first forking statement\n"); fork(); printf("Here I am just after first forking statement\n"); fork(); printf("Here I am just after second forking statement\n"); printf("\t\tHello World from process %d!\n", getpid(); } What is the output of the above program? How many child processes have been created in it?
Lab2.c #include #include /* contains fork prototype */ int main(void) { int pid; printf("Hello World!\n"); printf("I am the parent process and pid is : %d .\n",getpid()); printf("Here i am before use of forking\n"); pid = fork(); printf("Here I am just after forking\n"); if (pid == 0) printf("I am the child process and pid is :%d.\n",getpid()); else printf("I am the parent process and pid is: %d .\n",getpid()); } What is the process ID of the child process and parent process? Lab3.c (Multiple forks): #include #include /* contains fork prototype */ void main(void) { printf("Here I am just before first forking statement\n"); fork(); printf("Here I am just after first forking statement\n"); fork(); printf("Here I am just after second forking statement\n"); printf("\t\tHello World from process %d!\n", getpid(); } What is the output of the above program? How many child processes have been created in it?
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%

Transcribed Image Text:Lab2.c
#include <stdio.h>
#include <unistd.h> /* contains fork
prototype */ int main(void)
{
int pid;
printf("Hello World!\n");
printf("I am the parent process and pid is : %d .\n",getpid());
printf("Here i am before use of forking\n");
pid = fork();
printf("Here I am just after forking\n");
if (pid == 0)
printf("I am the child process and pid is :%d.\n",getpid());
else
printf("I am the parent process and pid is: %d .\n",getpid());
What is the process ID of the child process and parent process?
Lab3.c (Multiple forks):
#include <stdio.h>
#include <unistd.h> /* contains fork prototype */
void main(void)
printf("Here I am just before first forking statement\n");
fork();
printf("Here I am just after first forking statement\n");
fork();
printf("Here I am just after second forking statement\n");
printf("\t\tHello World from process %d!\n", getpid();
}
What is the output of the above program?
How many child processes have been created in it?

Transcribed Image Text:Lab4.c: Guarantees the child process will print its message before the paren
process, invoke wait(NULL) system call in parent process/
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <wait.h>/* contains prototype for wait */
void main(void)
{
int pid;
int status
printf("Hello World!\n");
pid = fork( );
if (pid == -1) /* check for error in fork */
{
perror("bad fork");
exit(1);
if (pid == 0)
printf("I am the child process. In");
e se
{
wait(NULL); /* parent waits for child to finish /
printf("I am the parent process.In");
Write Output:
Lab5.c:
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <wait.h>
main()
{
int forkresult;
printf("%d: I am the parent. Remember my number!\n", getpid());
printf("%d: I am now going to fork . In", getpid());
forkr
%3D
if (forkresult != 0)
{
printf("%d: My child's pid is %din", getpid(), forkresult);
}
else
{
printf("%d: Hi! I am the child.In", getpid());
printf("%d: like father like son. In", getpid());
What isthe output of child process?
What is the output of parent process?
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Recommended textbooks for you
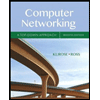
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
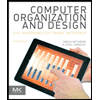
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
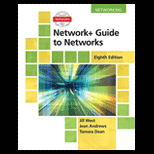
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
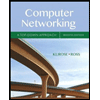
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
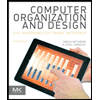
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
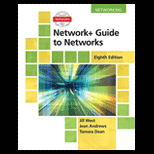
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
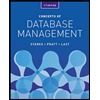
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
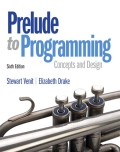
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
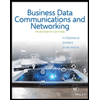
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY