Part 1: Your classmate Yurtle has a sticky keyboard and sometimes he presses down on a letter key for too long and so'a' will be typed as 'aa' or 'aaaa'. (For some reason the space bar and period keys work fine and all hose characters he wants to keep.) Create a java file with a class called StringHelper. In it write the method as described below. public static String clean5tring(String input) Write a static recursive method that creates a "clean string" getting rid of any of the adjacent duplicate letters. Your method may not contain any for loops. (Adjacent means "next to each other") Examples: . input: "wattteer in thhhhhhhheee lllakke" → output: "water in the lake" • input: "ggrreattt dayyy!!" → output: "great day!" input: "so. whhhaaaat diiidd you sayy?" → output: "so. what did you say?"
Part 1: Your classmate Yurtle has a sticky keyboard and sometimes he presses down on a letter key for too long and so'a' will be typed as 'aa' or 'aaaa'. (For some reason the space bar and period keys work fine and all hose characters he wants to keep.) Create a java file with a class called StringHelper. In it write the method as described below. public static String clean5tring(String input) Write a static recursive method that creates a "clean string" getting rid of any of the adjacent duplicate letters. Your method may not contain any for loops. (Adjacent means "next to each other") Examples: . input: "wattteer in thhhhhhhheee lllakke" → output: "water in the lake" • input: "ggrreattt dayyy!!" → output: "great day!" input: "so. whhhaaaat diiidd you sayy?" → output: "so. what did you say?"
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Note: The code needs to be in Java and the starter code needs to be used as well.
![Part 1:
Your classmate Yurtle has a sticky keyboard and sometimes he presses down on a letter key for too long and so 'a' will
be typed as 'aa' or 'aaaaa'. (For some reason the space bar and period keys work fine and all those characters he wants
to keep.)
Create a java íle with a class called 5tringHelper. In it write the method as described below.
public static 5tring clean5tring(String input)
Write a static recursive method that creates a "clean string" getting rid of any of the adjacent duplicate letters. Your
method may not contain any for loops. (Adjacent means "next to each other")
Examples:
input: "wattteer in thhhhhhhheee Illakke" → output: "water in the lake"
input: "egrreattt dayyy!!" → output: "great day!"
input: "so.. whhhaaaat diiidd you sayy?" → output: "so. what did you say?"
Hint: You will need to use the 5tring substring method.
Part 2:
Create a java file with a class name Recursive5ort. In it write an implementation of the recursive merge sort algorithm
discussed in class.
public static int] merge5ort(int] list)
Hint: You may want to make a separate helper mergelint] a, into b) method that will merge two sorted arrays into one
larger sorted array. A helper merge can contain a for loop.
Hint: You may also find the Arrays copyOfRange function useful. It creates a copy of an array between two indexes.
int] copiedArray = Arrays.copyOfRange(array, 0, 4);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd496c25d-6621-40aa-914c-10320ef32b36%2Fdbf51552-0f8e-4a79-930b-1ebf1c2b6427%2Fu6kgbv_processed.png&w=3840&q=75)
Transcribed Image Text:Part 1:
Your classmate Yurtle has a sticky keyboard and sometimes he presses down on a letter key for too long and so 'a' will
be typed as 'aa' or 'aaaaa'. (For some reason the space bar and period keys work fine and all those characters he wants
to keep.)
Create a java íle with a class called 5tringHelper. In it write the method as described below.
public static 5tring clean5tring(String input)
Write a static recursive method that creates a "clean string" getting rid of any of the adjacent duplicate letters. Your
method may not contain any for loops. (Adjacent means "next to each other")
Examples:
input: "wattteer in thhhhhhhheee Illakke" → output: "water in the lake"
input: "egrreattt dayyy!!" → output: "great day!"
input: "so.. whhhaaaat diiidd you sayy?" → output: "so. what did you say?"
Hint: You will need to use the 5tring substring method.
Part 2:
Create a java file with a class name Recursive5ort. In it write an implementation of the recursive merge sort algorithm
discussed in class.
public static int] merge5ort(int] list)
Hint: You may want to make a separate helper mergelint] a, into b) method that will merge two sorted arrays into one
larger sorted array. A helper merge can contain a for loop.
Hint: You may also find the Arrays copyOfRange function useful. It creates a copy of an array between two indexes.
int] copiedArray = Arrays.copyOfRange(array, 0, 4);
![Starter code:
// RecursionHomework.java
// Your name here
//
import java.util.Arrays;
class stringHelper {
Your classmete Yurtle has a sticky keyboard and sometimes he presses
down on a letter key for too long and so 'a' will be typed
as 'aa' or 'aaaaa'. (For some reason the space bar and period
keys work fine and all those characters he wants to keep.)
Create a java file with a class called stringHelper.
In it write the method as described below.
public static string cleanstring(string input)
krite a static recursive method that creates a "clean string"
getting rid of any of the adjacent duplicate letters.
Your method may not contain any for loops.
Examples:
input : "watttteer in thhhhhhhheee 111lakke" + out put : "water in the lake"
input: "egrreatttt dayyy!!" + out put : "great day!"
input: "so. whihhaaaat diiidd you sayy?" - out put : "so. what did you say?"
*/
public static string cleanstring(string input){
}
}
class Recursivesort {
Create a java file with a class name Recursivesort.
In it write an implementat ion of the recursive merge sort
algorithm discussed in class.
public static int [] mergesort(int [] list)
Hint: You may want to make a separate helper
merge(int [] a, int [] b) method that will merge two
sorted arrays into one larger sorted array.
*/
public static int [] mergesort(int [] list){
}
Testing:
Make sure you test your work. Include tests for base cases and recursive cases. (You have to make sure all branches of
your conditional logic work!)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd496c25d-6621-40aa-914c-10320ef32b36%2Fdbf51552-0f8e-4a79-930b-1ebf1c2b6427%2Fexo8x_processed.png&w=3840&q=75)
Transcribed Image Text:Starter code:
// RecursionHomework.java
// Your name here
//
import java.util.Arrays;
class stringHelper {
Your classmete Yurtle has a sticky keyboard and sometimes he presses
down on a letter key for too long and so 'a' will be typed
as 'aa' or 'aaaaa'. (For some reason the space bar and period
keys work fine and all those characters he wants to keep.)
Create a java file with a class called stringHelper.
In it write the method as described below.
public static string cleanstring(string input)
krite a static recursive method that creates a "clean string"
getting rid of any of the adjacent duplicate letters.
Your method may not contain any for loops.
Examples:
input : "watttteer in thhhhhhhheee 111lakke" + out put : "water in the lake"
input: "egrreatttt dayyy!!" + out put : "great day!"
input: "so. whihhaaaat diiidd you sayy?" - out put : "so. what did you say?"
*/
public static string cleanstring(string input){
}
}
class Recursivesort {
Create a java file with a class name Recursivesort.
In it write an implementat ion of the recursive merge sort
algorithm discussed in class.
public static int [] mergesort(int [] list)
Hint: You may want to make a separate helper
merge(int [] a, int [] b) method that will merge two
sorted arrays into one larger sorted array.
*/
public static int [] mergesort(int [] list){
}
Testing:
Make sure you test your work. Include tests for base cases and recursive cases. (You have to make sure all branches of
your conditional logic work!)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
![bartleby.com
O + 88
Homework 2 - Recursion
b Answered: Part 1: Your classmate Yurtle has a. | bartleby
Get live help whenever you need from online tutors! Try bartleby tutor today →
bartleby
Part 1:
QB
Your classmate Yurtle has a sticky keyboard and sometimes he presses down on a letter key for too long and so 'a' will
Homework help starts here!
be typed as 'aa' or 'aaaaa'. (For some reason the space bar and period keys work fine and all those characters he wants
CHAT V× MATH SOLVER
to keep.)
Create a java file with a class called 5tringHelper. In it write the method as described below.
Engineering » Computer Engineering » public static 5tring clean5tring(5tring input)
o long and so'a' will be typed as 'aa' or '...
Question
seen this question also like:
Write a static recursive method that creates a "clean string" getting rid of any of the adjacent duplicate letters. Your
method may not contain any for loops. (Adjacent means "next to each other")
Examples:
puter Networking: A Top-D... W
Note: The code needs to be in Jay
input: "wattteer in thhhhhhhheee Illlakke" → output: "water in the lake"
ition
input: "ggrreattt dayyy!!" → output: "great day!"
9780133594140
input: "so.. whhhaaaat diiidd you sayy?" → output: "so. what did you say?"
Part 1:
r: James Kurose, Keith Ross
her: PEARSON
Your classmate Yurtle Hint: You will need to use the 5tring substring method.
be typed as 'aa' or 'aaa
to keep.)
Create a java file with.
Section: Ch...
public static 5tring clea
Part 2:
Write a static recursiv
What is the difference...
method may not conta
Examples:
. input. "wattteer in Create a java file with a class name Recursive5ort. In it write an implementation of the recursive merge sort algorithm
• input: "egrreattt d discussed in class.
• input: "so. whhha
See similar books
public static int] merge5ortlint] list)
Hint: You will need to
Hint: You may want to make a separate helper merge(int] a, int] b) method that will merge two sorted arrays into one
Part 2:
larger sorted array. A helper merge can contain a for loop.
ick on your
mework?
Create a java fle with
Hint: You may also find the Arrays copyOfRange function useful. It creates a copy of an array between two indexes.
discussed in class.
public static int merg
int] copiedArray = Arrays.copyOfRange(array, 0, 4);
%3D
and get an expertly curated
Hint: You may want to
ist as 30 minutes.*
larger sorted array. A H
Hint: You may also find the Arrays copyOfRange function useful. It creates a copy of an array between two indexes.
int] copiedArray = Arrays.copyOf Range(array, 0, 4);
ASK AN EXPERT](https://content.bartleby.com/qna-images/question/2b8ea39f-2c16-4aa2-a1f4-bd4bf6254aad/d7ec5fab-317b-4f2a-b110-237274bc5d29/wz66v2d_thumbnail.png)
Transcribed Image Text:bartleby.com
O + 88
Homework 2 - Recursion
b Answered: Part 1: Your classmate Yurtle has a. | bartleby
Get live help whenever you need from online tutors! Try bartleby tutor today →
bartleby
Part 1:
QB
Your classmate Yurtle has a sticky keyboard and sometimes he presses down on a letter key for too long and so 'a' will
Homework help starts here!
be typed as 'aa' or 'aaaaa'. (For some reason the space bar and period keys work fine and all those characters he wants
CHAT V× MATH SOLVER
to keep.)
Create a java file with a class called 5tringHelper. In it write the method as described below.
Engineering » Computer Engineering » public static 5tring clean5tring(5tring input)
o long and so'a' will be typed as 'aa' or '...
Question
seen this question also like:
Write a static recursive method that creates a "clean string" getting rid of any of the adjacent duplicate letters. Your
method may not contain any for loops. (Adjacent means "next to each other")
Examples:
puter Networking: A Top-D... W
Note: The code needs to be in Jay
input: "wattteer in thhhhhhhheee Illlakke" → output: "water in the lake"
ition
input: "ggrreattt dayyy!!" → output: "great day!"
9780133594140
input: "so.. whhhaaaat diiidd you sayy?" → output: "so. what did you say?"
Part 1:
r: James Kurose, Keith Ross
her: PEARSON
Your classmate Yurtle Hint: You will need to use the 5tring substring method.
be typed as 'aa' or 'aaa
to keep.)
Create a java file with.
Section: Ch...
public static 5tring clea
Part 2:
Write a static recursiv
What is the difference...
method may not conta
Examples:
. input. "wattteer in Create a java file with a class name Recursive5ort. In it write an implementation of the recursive merge sort algorithm
• input: "egrreattt d discussed in class.
• input: "so. whhha
See similar books
public static int] merge5ortlint] list)
Hint: You will need to
Hint: You may want to make a separate helper merge(int] a, int] b) method that will merge two sorted arrays into one
Part 2:
larger sorted array. A helper merge can contain a for loop.
ick on your
mework?
Create a java fle with
Hint: You may also find the Arrays copyOfRange function useful. It creates a copy of an array between two indexes.
discussed in class.
public static int merg
int] copiedArray = Arrays.copyOfRange(array, 0, 4);
%3D
and get an expertly curated
Hint: You may want to
ist as 30 minutes.*
larger sorted array. A H
Hint: You may also find the Arrays copyOfRange function useful. It creates a copy of an array between two indexes.
int] copiedArray = Arrays.copyOf Range(array, 0, 4);
ASK AN EXPERT
Solution
Recommended textbooks for you
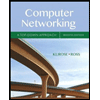
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
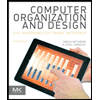
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
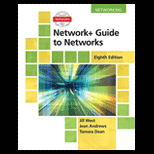
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
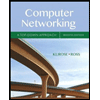
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
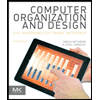
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
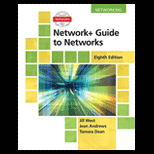
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
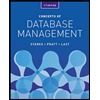
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
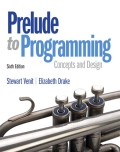
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
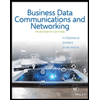
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY