Part 2: Dynamic Stack In this part, you are going to design a dynamic stack of characters implemented as a linked list. Complete the code below as specified by the comments below. typedef char el_t; class Stack { private: struct StackNode { el_t element; StackNode *next; // letter in the node // Pointer to the next node } ; StackNode *top; // Pointer to the stack top public: // Constructor Stack() { top = nullptr; } // Destructor -Stack () ; // Stack operations void push (el_t); void pop (el t &); void getTop (el_t &) const; void displayAll () const; bool isEmpty() const; } ; // implement the public methods highlighted in bold here int main () { Stack s; el_t c; cout << " initial stack contents" << endl; s.displayAl1(); s.pop (c); s.push('a'); cout << endl << " stack contents after pushing a: << endl; s.displayAl1(); s.push( 'b'); cout << endl << " stack contents after pushing b: s.displayAl1(); << endl; s.push('c'); s.push('d'); s.push('e'); s.push ('f'); s.push ( 'g') ; cout << endl << " stack contents after pushing c-g: " << endl; s.displayAl1(); s.getTop(c); cout << endl << "top elment is " << c < endl; s.pop (c); cout << endl << c << endl; cout << endl << stack contents after popping one element: << endl; s.displayAll(); s.pop (c); cout << endl << " " << c << endl; popped element: " stack contents after popping another element: cout << endl << << endl; s.displayAl1(); s.pop(c); s.pop (c); if (!s.isEmpty()) { s.getTop(c); cout << endl << "top elment is " << c << endl; } s.pop (c); cout << endl << stack contents after popping 3 more elements: << endl; s.displayAl1(); s.pop(c); s.pop(c); s.push('a'); s.push( 'b'); cout << endl << " final stack contents << endl; s.displayAll(); return 0;
Part 2: Dynamic Stack In this part, you are going to design a dynamic stack of characters implemented as a linked list. Complete the code below as specified by the comments below. typedef char el_t; class Stack { private: struct StackNode { el_t element; StackNode *next; // letter in the node // Pointer to the next node } ; StackNode *top; // Pointer to the stack top public: // Constructor Stack() { top = nullptr; } // Destructor -Stack () ; // Stack operations void push (el_t); void pop (el t &); void getTop (el_t &) const; void displayAll () const; bool isEmpty() const; } ; // implement the public methods highlighted in bold here int main () { Stack s; el_t c; cout << " initial stack contents" << endl; s.displayAl1(); s.pop (c); s.push('a'); cout << endl << " stack contents after pushing a: << endl; s.displayAl1(); s.push( 'b'); cout << endl << " stack contents after pushing b: s.displayAl1(); << endl; s.push('c'); s.push('d'); s.push('e'); s.push ('f'); s.push ( 'g') ; cout << endl << " stack contents after pushing c-g: " << endl; s.displayAl1(); s.getTop(c); cout << endl << "top elment is " << c < endl; s.pop (c); cout << endl << c << endl; cout << endl << stack contents after popping one element: << endl; s.displayAll(); s.pop (c); cout << endl << " " << c << endl; popped element: " stack contents after popping another element: cout << endl << << endl; s.displayAl1(); s.pop(c); s.pop (c); if (!s.isEmpty()) { s.getTop(c); cout << endl << "top elment is " << c << endl; } s.pop (c); cout << endl << stack contents after popping 3 more elements: << endl; s.displayAl1(); s.pop(c); s.pop(c); s.push('a'); s.push( 'b'); cout << endl << " final stack contents << endl; s.displayAll(); return 0;
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 3PE
Related questions
Question
in c++ language please

Transcribed Image Text:Part 2: Dynamic Stack
In this part, you are going to design a dynamic stack of characters implemented as a linked
list. Complete the code below as specified by the comments below.
typedef char el_t;
class Stack {
private:
struct StackNode
{
el_t element;
StackNode *next;
// letter in the node
// Pointer to the next node
} ;
StackNode *top;
// Pointer to the stack top
public:
// Constructor
Stack() {
top = nullptr; }
// Destructor
-Stack () ;
// Stack operations
void push (el_t);
void pop (el t &);
void getTop (el_t &) const;
void displayAll () const;
bool isEmpty() const;
} ;
// implement the public methods highlighted in bold here
int main ()
{
Stack s;
el_t c;
cout << " initial stack contents" << endl;
s.displayAl1();
s.pop (c);
s.push('a');
cout << endl << "
stack contents after pushing a:
<< endl;
s.displayAl1();
s.push( 'b');
cout << endl << " stack contents after pushing b:
s.displayAl1();
<< endl;

Transcribed Image Text:s.push('c');
s.push('d');
s.push('e');
s.push ('f');
s.push ( 'g') ;
cout << endl << "
stack contents after pushing c-g: "
<< endl;
s.displayAl1();
s.getTop(c);
cout << endl << "top elment is "
<< c < endl;
s.pop (c);
cout << endl << c << endl;
cout << endl <<
stack contents after popping one element:
<< endl;
s.displayAll();
s.pop (c);
cout << endl << "
" << c << endl;
popped element:
" stack contents after popping another element:
cout << endl <<
<< endl;
s.displayAl1();
s.pop(c);
s.pop (c);
if (!s.isEmpty())
{
s.getTop(c);
cout << endl << "top elment is " << c << endl;
}
s.pop (c);
cout << endl <<
stack contents after popping 3 more elements:
<< endl;
s.displayAl1();
s.pop(c);
s.pop(c);
s.push('a');
s.push( 'b');
cout << endl << " final stack contents << endl;
s.displayAll();
return 0;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
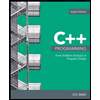
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
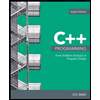
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning