Please help me design a SimulationProject.cpp in C++. THANK YOU! For this assignment, we will simulate boarding procedures for Airworthy Airlines. The Airline's current procedure is as follows: pre-board in the following order: families with young children or people who need help (e.g., wheelchair) first class and/or business class passengers elite passengers (frequent fliers) and those passengers seated in exit rows conduct general boarding in reverse, from the back of the plane to the front in the following order: rows 23-26 rows 17-22 rows 11-16 rows 5-10 Airworthy is considering revising their boarding procedure such that general boarding is done randomly, meaning the first passenger in line for general boarding is the first passenger to board (i.e., general boarding passengers all have the same priority). Airworthy suspects this random general boarding method will improve the flow of passengers, getting them on board and seated more quickly. It is also less labor-intensive for Airworthy's customer service agents because it significantly reduces the number of boarding announcements required and eliminates confrontations with customers trying to board "out of turn." Note that the revision is to general boarding only. The pre-boarding procedures will not be changed. The SimulationProject.cpp file contains a main() method and a method named description() that provides a detailed description for the user, explaining what the program is doing, how it works, and the location of all output files. Note that the description() method does NOT substitute for Javadoc comments. The audience for the description() method consists of non-technical users who have no information at all about the program or the assignment. The main() method must support the following: call the description() method call methods of the Airworthy class to load the priority queues and run the simulation using both the previous boarding procedure and the new random boarding procedure for each of 3 different input files. Each input file contains, on each line, the last name of the passenger, the type of passenger, and the row number in which the passenger is seated. The input files are: airworthy100.txt which loads the plane at 100% of capacity airworthy85.txt, which loads the plane at 85% of capacity airworthy70.txt, which loads the plane at 70% of capacity call methods of the Airworthy class to create 3 different output files named results100.txt, results85.txt, and results70.txt. Each output file should contain the data related to its similarly named input file. Be sure to title each part of the output and label all data so it is easy to identify. Each file should contain: a list of each passenger read from the input file, showing the passenger's name, type, and row a list of each passenger in order as they are removed from the priority queue when running the simulation using the current boarding procedure, showing the passenger's name, type, row, and priority value (the key) the total number of minutes (shown with 2 decimal places) required to board the plane using the current boarding procedure, a list of each passenger in order as they are removed from the priority queue when running the simulation for using the new random boarding procedure, showing the passenger's name, type, row, and priority value (the key), and the total number of minutes (shown with 2 decimal places) required to board the plane using the new random boarding procedure. HERE IS ONE OF THE ARIWORTHY70 FILE: Whitley G 8 Knowles G 1 Rocha G 24 Boyle G 24 Wooten G 2 Forbes G 16 Vinson E 1 Valencia E 7 Lindsay E 16 Rasmussen E 5 Sargent G 11 Sosa G 23 Head G 3 Holcomb G 5 Carney G 4 Kirkland G 14 Levine E 9 Cash G 10 Kaufman G 6 Ratliff G 9 Macias G 4 Sharpe G 17 Sweet G 17 Delaney G 9 Emerson G 5 Castaneda E 9 Rutledge G 26 Stuart G 19 Rosales G 23 Baird G 2 Clemons G 8 Mcgowan G 18 Compton E 10 Albert G 15 Acevedo G 14 Mayer E 9 Fitzpatrick G 16 Chaney G 8 Jarvis G 3 Berger G 26 Britt E 11 Odonnell E 8 Levy E 9 Mullen G 6 Pollard G 22 Lott G 10 Cantrell G 15 Holder E 5 Vaughan E 11 Mccarty E 24 Wilder G 11 Mayo G 1 Pickett G 8 Sykes G 26 Bender G 13 Aguirre G 16 Bernard G 10 Hopper H 7 Melendez G 13 Macdonald H 18 Carver G 15 Gould E 26 Suarez G 6 Zamora G 15 Hinton G 13 Cabrera G 26 Dickson G 22 Salas G 24 Bentley G 13 Fuentes G 23 Terrell H 3 Holman E 7 Mcintyre G 16 Hebert G 13 Hendricks G 3 Jacobson G 14 Kline G 14 Faulkner G 5 Chan G 14 Mays G 1 Crosby G 25 Buck G 22 Maddox G 20 Buckley E 17 Kane G 10 Rivas E 26 Dudley G 22 Best G 12 Finley G 24 William G 18 Frost G 2 Ashley G 14 Mcconnell G 7 Blevins G 11 Middleton G 17 Bean G 18 Sheppard G 11 Estes E 7 Pugh G 8 Rivers E 6 Barr G 4 Landry E 10 Foley G 2
Please help me design a SimulationProject.cpp in C++. THANK YOU!
For this assignment, we will simulate boarding procedures for Airworthy Airlines. The Airline's current procedure is as follows:
- pre-board in the following order:
- families with young children or people who need help (e.g., wheelchair)
- first class and/or business class passengers
- elite passengers (frequent fliers) and those passengers seated in exit rows
- conduct general boarding in reverse, from the back of the plane to the front in the following order:
- rows 23-26
- rows 17-22
- rows 11-16
- rows 5-10
Airworthy is considering revising their boarding procedure such that general boarding is done randomly, meaning the first passenger in line for general boarding is the first passenger to board (i.e., general boarding passengers all have the same priority). Airworthy suspects this random general boarding method will improve the flow of passengers, getting them on board and seated more quickly. It is also less labor-intensive for Airworthy's customer service agents because it significantly reduces the number of boarding announcements required and eliminates confrontations with customers trying to board "out of turn." Note that the revision is to general boarding only. The pre-boarding procedures will not be changed.
The SimulationProject.cpp file contains a main() method and a method named description() that provides a detailed description for the user, explaining what the program is doing, how it works, and the location of all output files. Note that the description() method does NOT substitute for Javadoc comments. The audience for the description() method consists of non-technical users who have no information at all about the program or the assignment. The main() method must support the following:
- call the description() method
- call methods of the Airworthy class to load the priority queues and run the simulation using both the previous boarding procedure and the new random boarding procedure for each of 3 different input files. Each input file contains, on each line, the last name of the passenger, the type of passenger, and the row number in which the passenger is seated. The input files are:
- airworthy100.txt which loads the plane at 100% of capacity
- airworthy85.txt, which loads the plane at 85% of capacity
- airworthy70.txt, which loads the plane at 70% of capacity
- call methods of the Airworthy class to create 3 different output files named results100.txt, results85.txt, and results70.txt. Each output file should contain the data related to its similarly named input file. Be sure to title each part of the output and label all data so it is easy to identify. Each file should contain:
- a list of each passenger read from the input file, showing the passenger's name, type, and row
- a list of each passenger in order as they are removed from the priority queue when running the simulation using the current boarding procedure, showing the passenger's name, type, row, and priority value (the key)
- the total number of minutes (shown with 2 decimal places) required to board the plane using the current boarding procedure,
- a list of each passenger in order as they are removed from the priority queue when running the simulation for using the new random boarding procedure, showing the passenger's name, type, row, and priority value (the key), and
- the total number of minutes (shown with 2 decimal places) required to board the plane using the new random boarding procedure.
HERE IS ONE OF THE ARIWORTHY70 FILE:
Whitley G 8
Knowles G 1
Rocha G 24
Boyle G 24
Wooten G 2
Forbes G 16
Vinson E 1
Valencia E 7
Lindsay E 16
Rasmussen E 5
Sargent G 11
Sosa G 23
Head G 3
Holcomb G 5
Carney G 4
Kirkland G 14
Levine E 9
Cash G 10
Kaufman G 6
Ratliff G 9
Macias G 4
Sharpe G 17
Sweet G 17
Delaney G 9
Emerson G 5
Castaneda E 9
Rutledge G 26
Stuart G 19
Rosales G 23
Baird G 2
Clemons G 8
Mcgowan G 18
Compton E 10
Albert G 15
Acevedo G 14
Mayer E 9
Fitzpatrick G 16
Chaney G 8
Jarvis G 3
Berger G 26
Britt E 11
Odonnell E 8
Levy E 9
Mullen G 6
Pollard G 22
Lott G 10
Cantrell G 15
Holder E 5
Vaughan E 11
Mccarty E 24
Wilder G 11
Mayo G 1
Pickett G 8
Sykes G 26
Bender G 13
Aguirre G 16
Bernard G 10
Hopper H 7
Melendez G 13
Macdonald H 18
Carver G 15
Gould E 26
Suarez G 6
Zamora G 15
Hinton G 13
Cabrera G 26
Dickson G 22
Salas G 24
Bentley G 13
Fuentes G 23
Terrell H 3
Holman E 7
Mcintyre G 16
Hebert G 13
Hendricks G 3
Jacobson G 14
Kline G 14
Faulkner G 5
Chan G 14
Mays G 1
Crosby G 25
Buck G 22
Maddox G 20
Buckley E 17
Kane G 10
Rivas E 26
Dudley G 22
Best G 12
Finley G 24
William G 18
Frost G 2
Ashley G 14
Mcconnell G 7
Blevins G 11
Middleton G 17
Bean G 18
Sheppard G 11
Estes E 7
Pugh G 8
Rivers E 6
Barr G 4
Landry E 10
Foley G 2

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

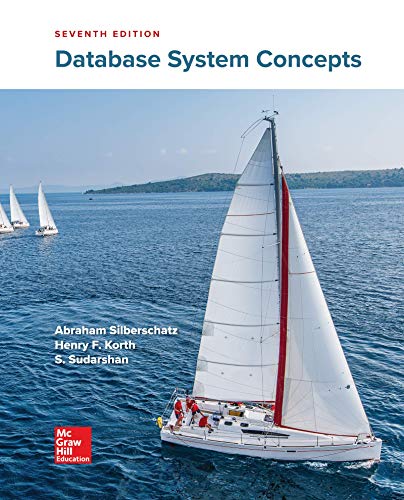
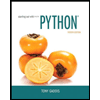
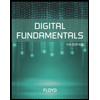
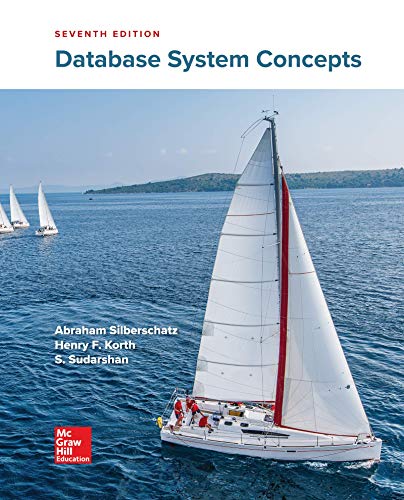
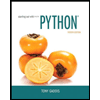
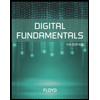
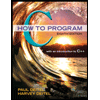
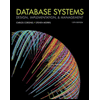
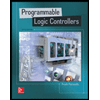