Priority Queue Lab - Emergency Room Write a class called WaitingRoom that simulates a Hospital Emergency Room. We will need two objects to complete this project. Patients and Beds. Patients will be represented as a class. Every Patient has a name and a category to indicate the severity of injury. There are three categories of patients: critical, major, and minor. The bed object itself is very simple. It only needs to know whether a bed is available, or which patient is using the bed. A reference to a Patient object can encode both pieces of information a null reference indicates that the bed is available. The Emergency Room will have ten available beds, therefore an array of ten Patients will server as our beds. Patients who arrive at the Emergency Room will stand in line at the admissions desk. They will be assigned a bed on a priority basis. Critical patients will be assigned first, then major injuries will be admitted followed by minor injuries. We can use a MaxHeapPriorityQueue to represent the waiting line. When a new patient is admitted, if the first person in the waiting line is in critical condition and no beds are available then a non-critical Patient should be bumped from his bed and the critical Patient assigned that bed. Patients that are bumped should be added to a list of bumped patients. When a new Patient is admitted, if there are no critical Patients waiting then the first person in the bumped list should be admitted. If there are no bumped patients then the first person in the waiting list should be assigned a bed. Write the Patient class. Each patient should have a name and a condition (injury). The condition is an integer value where 3 is critical, 2 is major, and I is minor. It would be helpful if you included three public constants to simplify writing this class and the main class. public static final int MINOR - 1; public static final int MAJOR-2; public static final int CRITICAL - 3; Patient should have a constructor that receives the patient's name and condition. It should have an accessor method for the condition (injury). Patient should implement the Comparable interface (this is required so that we can offer Patient objects to a PriorityQueue). The compare To method should be based on the condition. In addition Patient should have a toString method that returns the patient's name and condition, L.E. Mary Smith: Major or Bill Munroe: Critical. Write the Waiting Room class. Double click on WaitingRoomDocs.pdf for a list of fields and methods that should be included in your class. Open and run AdmissionsDesk.java (Main java in Repl.it) to test your WaitingRoom class.
package priorityqueue;
import java.util.Scanner;
public class AdmissionsDesk
{
private WaitingRoom hosp = new WaitingRoom();
public static void main(String[] args) {
AdmissionsDesk desk = new AdmissionsDesk();
}
public AdmissionsDesk() {
queuePatients();
fillBeds();
admissionDesk();
}
public void queuePatients()
{
Scanner reader = null;
try
{
reader = new Scanner(new File("patients.dat"));
}
catch (IOException e)
{
System.out.println(e);
System.exit(0);
}
while(reader.hasNextLine())
{
String str = reader.nextLine();
Scanner parser = new Scanner(str);
parser.useDelimiter("/");
// hosp.queuePatient(new Patient(parser.next(), parser.nextInt()));
}
}
public void fillBeds()
{
// for (int i = 0; i < 10; i++)
// hosp.admitPatient();
}
public void dischargePatient()
{
Scanner reader = new Scanner(System.in);
System.out.print("\nEnter The Bed Number To Be Discharged: ");
int bedNr = reader.nextInt();
while (bedNr < 1 || bedNr > 10)
{
System.out.println("Invalid Bed Number!");
System.out.println("Enter The Bed Number To Be Discharged: ");
bedNr = reader.nextInt();
}
System.out.println();
// hosp.dischargePatient(bedNr);
}
public void queuePatient()
{
Scanner reader = new Scanner(System.in);
System.out.print("\nEnter The Patient's Name: ");
String name = reader.nextLine();
System.out.println("\nCondition");
System.out.println(" 1 = Minor Injury");
System.out.println(" 2 = Major Injury");
System.out.println(" 3 = Critical Injury");
System.out.print("\nEnter the Patient's Condition: ");
int condition = reader.nextInt();
while (condition < 1 || condition > 3)
{
System.out.println("Invalid Condition!");
System.out.print("Enter the Patient's Condition: ");
condition = reader.nextInt();
}
System.out.println();
// hosp.queuePatient(new Patient(name, condition));
}
public void admissionDesk()
{
/* int option = getOption();
while (option != 0)
{
switch (option)
{
case 1: if (hosp.admitPatient())
System.out.println("\n Patient Admitted!\n");
else
System.out.println("\nNo Available Beds!\n");
break;
case 2: dischargePatient();
break;
case 3: queuePatient();
break;
case 4: showBeds();
break;
case 5: showBumpedPatients();
break;
case 6: showWaitingList();
break;
}
option = getOption();
}
System.out.println("Bye..."); */
}
public int getOption()
{
Scanner reader = new Scanner(System.in);
System.out.println("... EMERGENCY ROOM ...");
System.out.println("\n0 - Exit The Program");
System.out.println("1 - Admit A New Patient");
System.out.println("2 - Discharge A Patient");
System.out.println("3 - Queue A New Patient");
System.out.println("4 - Show Occupied Beds");
System.out.println("5 - Show Bumped Patients");
System.out.println("6 - Show Waiting Patients");
System.out.println();
System.out.print("Select An Option: ");
int option = reader.nextInt();
while (option < 0 || option > 6)
{
System.out.println("Invalid Option!");
System.out.print("Select An Option: ");
option = reader.nextInt();
}
return option;
}
public void showWaitingList()
{
System.out.println("\nWaiting In Line In The Emergency Room: ");
/* List<Patient> list = hosp.getWaitingList();
for (Patient p : list)
System.out.println(p + " ");
System.out.println(); */
}
public void showBeds()
{ /*
System.out.println("\nHospital Beds:");
List<Patient> beds = hosp.getPatients();
for (int i = 0; i < beds.size(); i++)
{
if (beds.get(i) != null)
System.out.println("Bed " + (i + 1) + ": " + beds.get(i));
}
System.out.println(); */
}
public void showBumpedPatients()
{ /*
System.out.println("\nBumped Patients:");
List<Patient> list = hosp.getBumpedPatients();
for (Patient p : list)
System.out.println(p + " ");
System.out.println(); */
}



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Thank you for the code, I am just wondering how the implementation works for Admissions.java, did you code an entirely new Admissions, or are those pieces of code made to be put into the already existing Admissions desk, if so where?
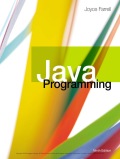
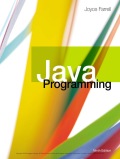