Private Member Functions The functions declared private above, is Leap, daysPerMonth, name, number, are helper functions - member functions that will never be needed by a user of the class, and so do not belong to the public interface (which is why they are "private"). They are, however, needed by the interface functions (public member functions), which use them to test the validity of arguments and construct valid dates. For example, the constructor that passes in the month as a string will call the number function to assign a value to the unsigned member variable month. isLeap: The rule for whether a year is a leap year is: • (year % 4 == 0) implies leap year • except (year % 100 == 0) implies NOT leap year • except (year % 400 == 0) implies leap year So, for instance, year 2000 is a leap year, but 1900 is NOT a leap year. Years 2004, 2008, 2012, 2016, etc. are all leap years. Years 2005, 2006, 2007, 2009, 2010, etc. are NOT leap years. Output Specifications Read the specifications for the print function carefully. The only cout statements within your Date member functions should be: 1. the "Invalid Date" warnings in the constructors 2. in your two print functions Required Main Function You must use this main function and global function getDate as they are here. You may not change these functions at all. Copy-and-paste these into your main.cpp file and then add the Date class. Date getDate(); int main() { } Date test Date; test Date = getDate(); cout << endl; cout << "Numeric: "; testDate.printNumeric (); cout << endl; cout << "Alpha: "; testDate.printAlpha (); cout << endl; return 0; Date getDate() { int choice; unsigned monthNumber, day, year; string monthName; cout << "Which Date constructor? (Enter 1, 2, or 3)" << endl << "1 Month Number" << endl << "2 Month Name" << endl << "3 default" << endl; cin >> choice; cout << endl;
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
There's additional code that's required. I have attached the instructions below. It's continuous.



Trending now
This is a popular solution!
Step by step
Solved in 5 steps

Can you please explain this part again?
Date::Date :day(1),month(1),year(2000)
{
}
Is it written this way? I am a little confused about how to write this portion at the start in the Date.cpp file, can you please rewrite this part more clearly?
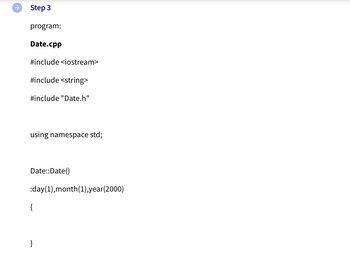
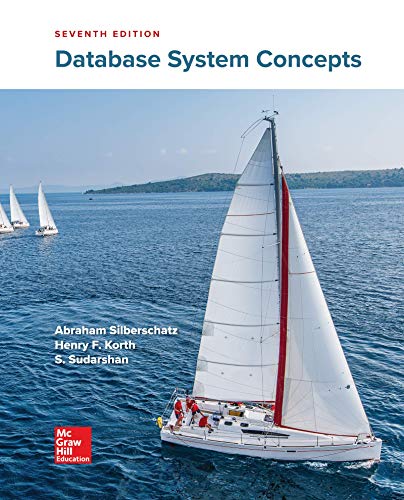
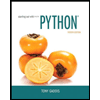
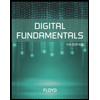
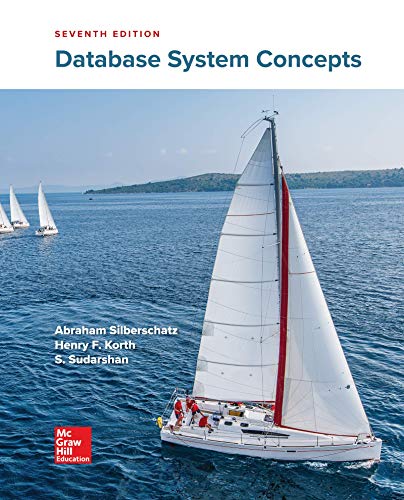
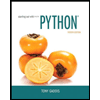
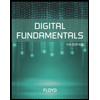
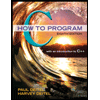
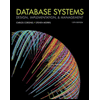
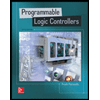