Write the function with the following name twobody_dynamics_first_order_EoMs(t, states) that describes the equations of motion you have derived above in Question 1.1. states represents the 6-dimensional column vector X in Equation (1.1). ]: # Run this cell, should import everything you need import numpy as np from scipy. integrate import solve_ivp from math import pi, sin, cos, sqrt, acos, asin, tan, atan import matplotlib.pyplot as plotter ]: # YOUR CODE HERE raise NotImplementedError() NotImplementedError Cell In [2], line 2 1 # YOUR CODE HERE 2 raise NotImplementedError() Not ImplementedError: Traceback (most recent call last)
Write the function with the following name twobody_dynamics_first_order_EoMs(t, states) that describes the equations of motion you have derived above in Question 1.1. states represents the 6-dimensional column vector X in Equation (1.1). ]: # Run this cell, should import everything you need import numpy as np from scipy. integrate import solve_ivp from math import pi, sin, cos, sqrt, acos, asin, tan, atan import matplotlib.pyplot as plotter ]: # YOUR CODE HERE raise NotImplementedError() NotImplementedError Cell In [2], line 2 1 # YOUR CODE HERE 2 raise NotImplementedError() Not ImplementedError: Traceback (most recent call last)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
![Write the function with the following name twobody_dynamics_first_order_EoMs(t, states) that
describes the equations of motion you have derived above in Question 1.1. states represents the 6-dimensional
column vector X in Equation (1.1).
L]: # Run this cell, should import everything you need
import numpy as np
from scipy.integrate import solve_ivp
from math import pi, sin, cos, sqrt, acos, asin, tan, atan
import matplotlib.pyplot as plotter
21: # YOUR CODE HERE
raise Not ImplementedError()
Not ImplementedError
Cell In [2], line 2
1 # YOUR CODE HERE
2 raise NotImplementedError()
Not ImplementedError:
Traceback (most recent call last)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7830619d-3fbe-414c-9d12-28af960fe618%2Faccaf603-22de-43e1-b5e0-ac4e5230ba88%2F8pi7eqj_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Write the function with the following name twobody_dynamics_first_order_EoMs(t, states) that
describes the equations of motion you have derived above in Question 1.1. states represents the 6-dimensional
column vector X in Equation (1.1).
L]: # Run this cell, should import everything you need
import numpy as np
from scipy.integrate import solve_ivp
from math import pi, sin, cos, sqrt, acos, asin, tan, atan
import matplotlib.pyplot as plotter
21: # YOUR CODE HERE
raise Not ImplementedError()
Not ImplementedError
Cell In [2], line 2
1 # YOUR CODE HERE
2 raise NotImplementedError()
Not ImplementedError:
Traceback (most recent call last)

Transcribed Image Text:1. solve a system of ordinary differential equations of first order numerically using solve_ivp.
2. generate plots after "solving" the differential equations using matplotlib.pyplot. In that tutorial, the
equations of motion described the dynamics of a simple pendulum. In this problem, you will investigate the
motion of a satellite orbiting the Earth. This is modelled as a two-body dynamics system. A brief outline of
what you need to do in this problem set is below and that is followed by a systematic set of questions towards
achieving specific objectives. You will find the tutorial a handty reference here.
In this problem, you will have to first create a Python function called twobody_dynamics_first_order_EOMS.
Given a time t and a state vector X, this function will return the derivatives of the state vector. Mathematically,
this means you are computing X using some dynamics equation X = f(t, X).
Once you have this function in Python, you can solve the differential equations it contains by using solve_ivp.
The command will be similar to, but not necessarily exactly, what is shown below:
solve_ivp (simple_pendulum_first_order_EoMS, t_span, initial_conditions,
args=constants, rtol = 1e-8, atol = 1e-8)
which integrates the differential equations of motion to give us solutions to the states (i.e., position and velocity of
a satellite). In the above, t_span contains the initial time to and final time tƒ and it will compute the solution at
every instant of time (you will define this later in Problem 1.3 below).
The integration is done with initial state vector Xo which defines the initial position and velocity. Xo needs to be a
column vector.
You will need to use the following constants in arriving at your answers:
• Earth gravitational parameter = 3.986 × 105 km³/s²
• Earth mean radius = 6378.14 km
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
![Plot the orbit in 3D using a suitably dimensioned sphere for the Earth.
[6]: # YOUR CODE HERE
raise NotImplementedError()
Not Implemented Error
Cell In [6], line 2
1 # YOUR CODE HERE
----> 2 raise NotImplementedError()
Not ImplementedError:
Problem 1.6
Plot the Specific Energies (i.e., all three Kinetic, Potential and Total Energy) in the same plot as a function of time.
The x-axis must show time (in hours) and y axis must show the Energy (in km² /s²).
[7] # YOUR CODE HERE
Traceback (most recent call last)
raise NotImplementedError()](https://content.bartleby.com/qna-images/question/7830619d-3fbe-414c-9d12-28af960fe618/5e15e086-efb6-4211-bedc-0aad6f43debe/naevtsp_thumbnail.jpeg)
Transcribed Image Text:Plot the orbit in 3D using a suitably dimensioned sphere for the Earth.
[6]: # YOUR CODE HERE
raise NotImplementedError()
Not Implemented Error
Cell In [6], line 2
1 # YOUR CODE HERE
----> 2 raise NotImplementedError()
Not ImplementedError:
Problem 1.6
Plot the Specific Energies (i.e., all three Kinetic, Potential and Total Energy) in the same plot as a function of time.
The x-axis must show time (in hours) and y axis must show the Energy (in km² /s²).
[7] # YOUR CODE HERE
Traceback (most recent call last)
raise NotImplementedError()
Solution
Follow-up Question
Please guide
![Problem 1.3
Plot the magnitude of the position vector versus time. The x-axis must show time (in hours) and y axis must show
the radius (in km).
[4]: # YOUR CODE HERE
raise NotImplementedError()
Not ImplementedError
Cell In [4], line 2
1 # YOUR CODE HERE
----> 2 raise NotImplementedError()
Not ImplementedError:
Problem 1.4
Plot the magnitude of the velocity vector versus time. The x-axis must show time (in hours) and y axis must show
the radius (in km/s).
[5]: # YOUR CODE HERE
raise NotImplementedError()
Not ImplementedError
Cell In [5], line 2
Traceback (most recent call last)
1 # YOUR CODE HERE
----> 2 raise NotImplementedError()
Traceback (most recent call last)](https://content.bartleby.com/qna-images/question/7830619d-3fbe-414c-9d12-28af960fe618/ca1a8aea-3763-4f32-8744-4c5d3a4c74f4/0qreaj_thumbnail.jpeg)
Transcribed Image Text:Problem 1.3
Plot the magnitude of the position vector versus time. The x-axis must show time (in hours) and y axis must show
the radius (in km).
[4]: # YOUR CODE HERE
raise NotImplementedError()
Not ImplementedError
Cell In [4], line 2
1 # YOUR CODE HERE
----> 2 raise NotImplementedError()
Not ImplementedError:
Problem 1.4
Plot the magnitude of the velocity vector versus time. The x-axis must show time (in hours) and y axis must show
the radius (in km/s).
[5]: # YOUR CODE HERE
raise NotImplementedError()
Not ImplementedError
Cell In [5], line 2
Traceback (most recent call last)
1 # YOUR CODE HERE
----> 2 raise NotImplementedError()
Traceback (most recent call last)
Solution
Follow-up Question
![Problem 1.2
Use solve_ivp to integrate the equations of motion of the two-body problem. The initial position and velocity
state vector Xo = (r, v)™ is:
and
3]: # YOUR CODE HERE
r =
raise NotImplementedError()
V =
The duration of the simulation is for one day but you should compute the solutuion for every ten seconds. We
show you how to control this below but you will have to make changes to this code to get it to work correctly for
this simiualtion.
Not ImplementedError
Cell In [3], line 2
Lastly, when using solve_ivp make sure that you use rtol=1e-12 and atol
[6510.759869015321
2676.16546382759 km
333.334029373109
[-2.232024608624287
9.49860960555864 km/s
1.18311436869621
1 # YOUR CODE HERE
----> 2 raise NotImplementedError()
= 1e-12.
Traceback (most recent call last)](https://content.bartleby.com/qna-images/question/7830619d-3fbe-414c-9d12-28af960fe618/17d05dcf-faaf-4dc1-b767-cbe87ed94449/suadwnm_thumbnail.jpeg)
Transcribed Image Text:Problem 1.2
Use solve_ivp to integrate the equations of motion of the two-body problem. The initial position and velocity
state vector Xo = (r, v)™ is:
and
3]: # YOUR CODE HERE
r =
raise NotImplementedError()
V =
The duration of the simulation is for one day but you should compute the solutuion for every ten seconds. We
show you how to control this below but you will have to make changes to this code to get it to work correctly for
this simiualtion.
Not ImplementedError
Cell In [3], line 2
Lastly, when using solve_ivp make sure that you use rtol=1e-12 and atol
[6510.759869015321
2676.16546382759 km
333.334029373109
[-2.232024608624287
9.49860960555864 km/s
1.18311436869621
1 # YOUR CODE HERE
----> 2 raise NotImplementedError()
= 1e-12.
Traceback (most recent call last)
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
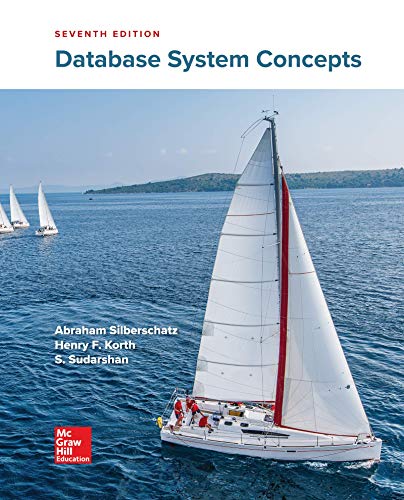
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
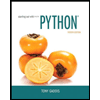
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
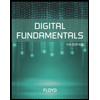
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
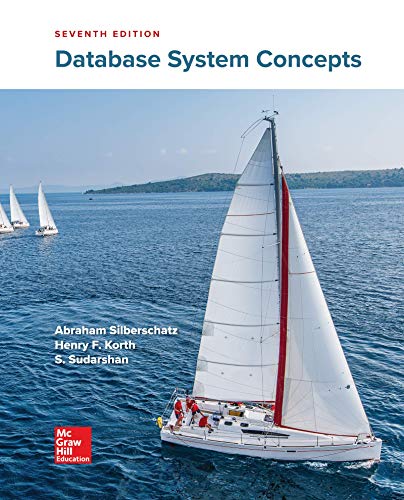
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
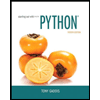
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
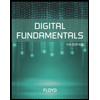
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
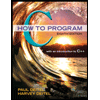
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
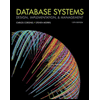
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
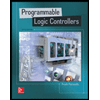
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education