Problem: Your program will implement the preparation routines used in a card game played among four players. First, the program shuffles a deck of cards, then it deals the cards to each of the four players, one at a time in clockwise rotation. After all cards are dealt to the players, the program organizes the cards in each player's hand by sorting their cards by suit. Then the program will display each player's cards. In this project, you are required to define a class called "CardClass". The 2 data members of this class are: the deck of cards implemented in terms of an array of type "CardType" of size 52, the count of how many cards are remaining in the deck. Each card is described by its suit, value, and points in game. Define a structured data type CardType with Following components: suit (CardSuitType), value (int), and points (int). For suit, create an enumeration type called "CardSuitType" that has the four values: DIAMOND, CLUB, HEART, SPADE. The class should include at least the following member functions: L. Default constructor which creates the deck of cards as the following: • The deck of cards should be represented as an array of CardType. The size of the array is 52. Each card is described by its suit, value, and points in game.
Problem: Your program will implement the preparation routines used in a card game played among four players. First, the program shuffles a deck of cards, then it deals the cards to each of the four players, one at a time in clockwise rotation. After all cards are dealt to the players, the program organizes the cards in each player's hand by sorting their cards by suit. Then the program will display each player's cards. In this project, you are required to define a class called "CardClass". The 2 data members of this class are: the deck of cards implemented in terms of an array of type "CardType" of size 52, the count of how many cards are remaining in the deck. Each card is described by its suit, value, and points in game. Define a structured data type CardType with Following components: suit (CardSuitType), value (int), and points (int). For suit, create an enumeration type called "CardSuitType" that has the four values: DIAMOND, CLUB, HEART, SPADE. The class should include at least the following member functions: L. Default constructor which creates the deck of cards as the following: • The deck of cards should be represented as an array of CardType. The size of the array is 52. Each card is described by its suit, value, and points in game.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:• A card value is the face value of the card which is from 2 to 14, i.e., 11 for Jack, 12 for Queen, 13 for
King, and 14 for Ace.
• For card points in game, all the cards of HEART suit have points: each HEARTS card of less than 10 face
value has 5 points; HERATS of 10, Jack, Queen, King and Ace have 10 points. All Spade, Diamond, and
Club cards have a value 0, except that the Queen of Spade has a point of 100, Jack of Diamond has a
point of -100.
• ShuffleCard randomizes the 52 cards in the deck
• DealCard deals out, i.e., returns, one card when the function is called. The card dealt out should be from
the top of the deck. It should also decrement the number of cards remaining in deck by 1.
• GetSize returns the number of cards currently in the deck
• IsEmpty which returns whether or not the deck is empty, e.g., no cards remaining
After you have defined the "CardClass", write a client program that :
• creates an object of CardClass;
• deals cards to 4 players (Each player is defined as a 1-D array of CardStruct type)
• Write a user defined function SortCards to sort one player's cards by suit, and optionally, sort by value
within each suit.
• Write a user defined function PrintCards to display the cards in one player's hand. The card suit, value and
points in game for each card are displayed. Output should be nicely formatted. Print suit and value with
meaningful name not numbers.
• Display which player has 2 of Club. This player will later start the first round of the game.
Make sure to include detailed description (description, pre-condition, post-condition) of each method in the
class in the header file.
Notes:
• Put the definition of "CardStruct" in CardClass.h,
• This is the first part of a larger program. If this part does not work properly, it can affect the next project.

Transcribed Image Text:Instructions
X
Failed to load "Picture1.png"
CSCI 3110 Project 1
Problem: Your program will implement the preparation routines used in a card game played among four
players. First, the program shuffles a deck of cards, then it deals the cards to each of the four players, one at
a time in clockwise rotation. After all cards are dealt to the players, the program organizes the cards in each
player's hand by sorting their cards by suit. Then the program will display each player's cards.
In this project, you are required to define a class called "CardClass". The 2 data members of this class are:
• the deck of cards implemented in terms of an array of type "CardType" of size 52,
• the count of how many cards are remaining in the deck.
Each card is described by its suit, value, and points in game. Define a structured data type CardType with
following components:
• suit (CardSuitType),
• value (int), and
• points (int).
For suit, create an enumeration type called "CardSuitType" that has the four values: DIAMOND, CLUB,
HEART, SPADE.
The class should include at least the following member functions:
1. Default constructor which creates the deck of cards as the following:
The deck of cards should be represented as an array of CardType. The size of the array is 52. Each card
is described by its suit, value, and points in game.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
Recommended textbooks for you
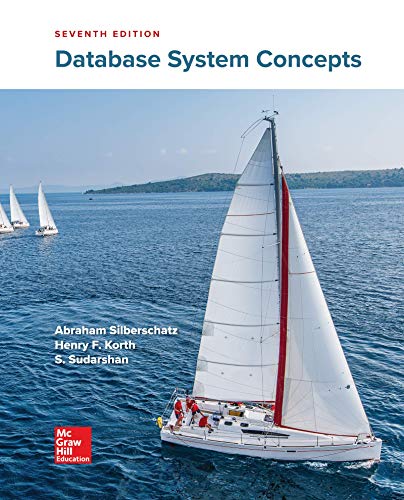
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
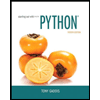
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
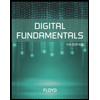
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
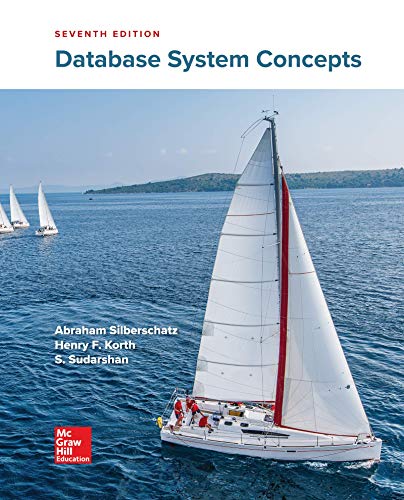
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
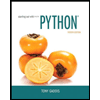
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
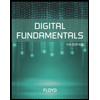
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
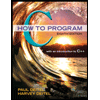
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
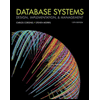
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
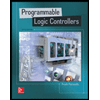
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education