Provide c++ code for main.cpp, dynamicarray.h and dynamicarray.cpp Step 1a: Preparation The suite of
Provide c++ code for main.cpp, dynamicarray.h and dynamicarray.cpp
Step 1a: Preparation
- The suite of Part 1 tests has been moved to its own function.
- The code in main() is focused on testing the new operators you'll write in this part.
- main() relies on a change you're going to make to the DynamicArray::print() member function (coming up in Step 1b). Instead of directly using cout, this member function is now going to print to a stringstream. This allows my test code to examine the output and check it automatically.
3 representative lines of the new main program work:
// Use stringstream to capture your print function's output.
stringstream testoutput;
// Use the same testoutput variable over and over, so we reset it to the empty string between tests using its str() member function.
testoutput.str(""); // Reset test output string
// Call the print function on your array, and it prints to testoutput instead of cout.
a.print(testoutput);
// Use the RunIndividualTest function also included in main.cpp to compare the output you got to the desired output.
// We call the str member function with no parameters as it converts the stringstream's contents to a normal string.
pass &= RunIndividualTest("sampledesiredoutput", testoutput.str(), "NameOfTest");
Step 1b: Modify some methods
Make changes to DynamicArray code:
- Add the word const at the end of the at, sum, print, and cap function headers in both the .cpp and the .h files. For example:
- int sum() const;
- int cap() const { return capacity; }
- Modify print to take an output stream as a parameter instead of assuming cout. The new prototype for the .h file is:
-
- void print(std::ostream& s) const;
Change the implementation in the .cpp file by replacing "cout" with "s" then recompile your code.
Compile and run the new program and verify that it shows you still pass the Part 1 tests.
Step 2: Copy Constructor
Do the following for each member variable:
- If the member variable is not a pointer: copy it directly from the other object
- If the member variable IS a pointer to dynamically allocated memory: allocate your own space in memory, and copy contents from the other object as needed.
Modify it for this class and add it to the header file.
- Classname(const Classname& source);
For definition, follow the prompts:
// capacity and len are ints, so they're covered by case 1
capacity =
len =
// arr points to dynamically allocated memory.
// Directly copying arr = other.arr doesn't actually
// COPY that memory
// objects referencing the same underlying storage.
// First allocate new memory for this new object and then copy over the values from the other object.
arr =
// Copy the elements from other array to our new space
for (int i = 0; i < len; i++) {
}
Then un-comment these lines in main.cpp:
DynamicArray b(a);
…
cout << "*** Lab 6 (Part 1) tests on array created with copy constructor: "
<< (RunPart1Tests(b) ? "passed" : "failed") << endl;
What's happening in your code in order:
- Created an empty DynamicArray, a.
- Created a DynamicArray, b, as a copy of a (also empty).
- Ran the Part 1 tests on a, which added and removed a bunch of values; leaving the array with only one element: 152.
- Run the same tests on b, with the same result.
Compile this code and verify that you passed the tests.
Step 3: Equality Comparison Operator
We don't need arr == other.arr for the arrays to be equal.
For declaration: The general form of the prototype is below. Modify it for this class and add it to the header file.
- bool operator==(const Classname& source) const;
Follow the prompts below for definition:
// Check for matching length
// Check to make sure that each element matches
// Be sure you've returned true if and only if the length matches and every individual element up to the length also matches. Otherwise, make sure you've returned false.
When you've defined this operator, un-comment the equality comparison test in main (to just before some append and remove calls), recompile, and rerun.
Step 4: Assignment Operator
Modify general form of the prototype for this class and add it to the header file:
- Classname& operator= (const Classname& source);
The last line of your assignment operator definition will always be:
return *this; // return the thing "this" points to; i.e. the current object
Follow the prompts below for definition:
// For member variables that are not pointers, do the same as the copy constructor
// Before deallocating current array, double-check that the client isn't doing something like myarr = myarr;
// 1. Deallocate current object's array
// 2. Reallocate array to the new capacity
// 3. Copy data from old array
// The safest thing is to just return now in this case is already equal to itself and there's no work to do.
if (this == &source) {
// get out!!
}
// Reallocate and copy (same code as copy constructor)
// Need a return statement

Step by step
Solved in 3 steps

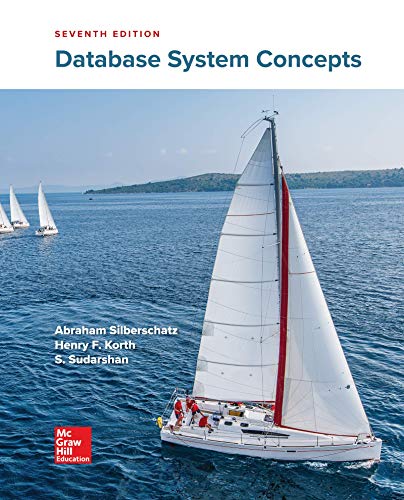
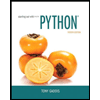
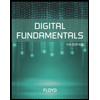
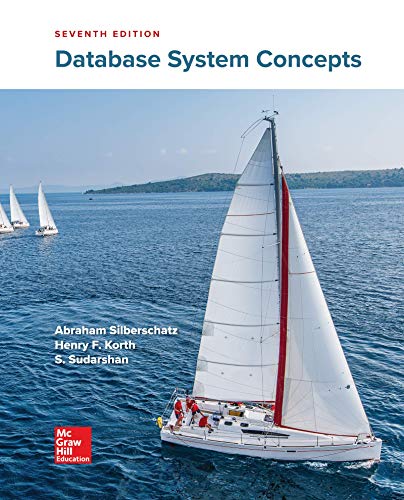
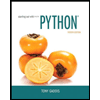
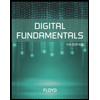
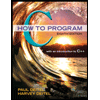
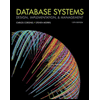
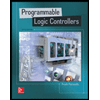