returns: { } "error": "Topic 'success' not found" Step 1: Fetch the quotes The fetchQuotes() function in quote.js is called with the selected topic and count when the Fetch Quotes button is clicked. Currently, fetchQuotes() calls showAnonymousQuotes() to display example quotes in an ordered list. Modify fetchQuotes() to use the XMLHttpRequest object to request quotes from the quote web API. Set the XMLHttpRequest's responseType to expect a JSON response. Register response Received Handler() as the XMLHttpRequest's load event handler. Step 2: Display the quotes Implement responseReceived Handler() to extract the quotes from the XMLHttpRequest response and display the quotes in an ordered list. Each quote should be followed by a space, a dash, a space, and the source. You may find it helpful to repurpose the code in showAnonymousQuotes() to create the quote list. Ex: If the user chooses "love" and "3" and clicks Fetch Quotes, response Received Handler() should place the returned quotes in an ordered list inside the : - Hermann Hesse Elie Wiesel If I know what love is, it is because of you. The opposite of love is not hate, it's indifference. Suffering passes, while love is eternal. Laura Ingalls Wilder If an error message is received, the error message should be displayed in the . Ex: Topic success' not found - Quote web API A quote web API returns a collection of randomly selected quotes related to a given topic. The API supports two query string parameters: topic - Specifies the requested topic. Valid topics are love, motivational, wisdom, and humor. ⚫ count - Specifies the number of quotes requested and must be a number from 1 to 5. Ex: The API request: https://wp.zybooks.com/quotes.php?topic=love&count=3 returns 3 quotes about love, formatted in JSON: { }, { }, { "quote": "If I know what love is, it is because of you.", "source": "Hermann Hesse" "quote": "The opposite of love is not hate, it's indifference.", "source": "Elie Wiesel" "quote": "Suffering passes, while love is eternal.", "source": "Laura Ingalls Wilder" If the topic is not given or not recognized, the API returns an error message. Ex: The request for a "success" quote: https://wp.zybooks.com/quotes.php?topic=success&count=1 returns: { 'error": "Topic 'success' not found"
returns: { } "error": "Topic 'success' not found" Step 1: Fetch the quotes The fetchQuotes() function in quote.js is called with the selected topic and count when the Fetch Quotes button is clicked. Currently, fetchQuotes() calls showAnonymousQuotes() to display example quotes in an ordered list. Modify fetchQuotes() to use the XMLHttpRequest object to request quotes from the quote web API. Set the XMLHttpRequest's responseType to expect a JSON response. Register response Received Handler() as the XMLHttpRequest's load event handler. Step 2: Display the quotes Implement responseReceived Handler() to extract the quotes from the XMLHttpRequest response and display the quotes in an ordered list. Each quote should be followed by a space, a dash, a space, and the source. You may find it helpful to repurpose the code in showAnonymousQuotes() to create the quote list. Ex: If the user chooses "love" and "3" and clicks Fetch Quotes, response Received Handler() should place the returned quotes in an ordered list inside the : - Hermann Hesse Elie Wiesel If I know what love is, it is because of you. The opposite of love is not hate, it's indifference. Suffering passes, while love is eternal. Laura Ingalls Wilder If an error message is received, the error message should be displayed in the . Ex: Topic success' not found - Quote web API A quote web API returns a collection of randomly selected quotes related to a given topic. The API supports two query string parameters: topic - Specifies the requested topic. Valid topics are love, motivational, wisdom, and humor. ⚫ count - Specifies the number of quotes requested and must be a number from 1 to 5. Ex: The API request: https://wp.zybooks.com/quotes.php?topic=love&count=3 returns 3 quotes about love, formatted in JSON: { }, { }, { "quote": "If I know what love is, it is because of you.", "source": "Hermann Hesse" "quote": "The opposite of love is not hate, it's indifference.", "source": "Elie Wiesel" "quote": "Suffering passes, while love is eternal.", "source": "Laura Ingalls Wilder" If the topic is not given or not recognized, the API returns an error message. Ex: The request for a "success" quote: https://wp.zybooks.com/quotes.php?topic=success&count=1 returns: { 'error": "Topic 'success' not found"
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter7: File Handling And Applications
Section: Chapter Questions
Problem 1PE
Question
here is the javascript code that has to be modified
window.addEventListener("DOMContentLoaded", function () {
document.querySelector("#fetchQuotesBtn").addEventListener("click", function () {
// Get values from drop-downs
const topicDropdown = document.querySelector("#topicSelection");
const selectedTopic = topicDropdown.options[topicDropdown.selectedIndex].value;
const countDropdown = document.querySelector("#countSelection");
const selectedCount = countDropdown.options[countDropdown.selectedIndex].value;
// Get and display quotes
fetchQuotes(selectedTopic, selectedCount);
});
});
function showAnonymousQuotes(count) {
let html = "<ol>";
for (let c = 1; c <= count; c++) {
html += `<li>Quote ${c} - Anonymous</li>`;
}
html += "</ol>";
document.querySelector("#quotes").innerHTML = html;
}
function fetchQuotes(topic, count) {
// TODO: Modify to use XMLHttpRequest
// TODO: Remove the call to showAnonymousQuotes()
showAnonymousQuotes(count);
}
function responseReceivedHandler() {
// TODO: Complete the function
}

Transcribed Image Text:returns:
{
}
"error": "Topic 'success' not found"
Step 1: Fetch the quotes
The fetchQuotes() function in quote.js is called with the selected topic and count when the Fetch Quotes button is clicked. Currently,
fetchQuotes() calls showAnonymousQuotes() to display example quotes in an ordered list.
Modify fetchQuotes() to use the XMLHttpRequest object to request quotes from the quote web API. Set the XMLHttpRequest's
responseType to expect a JSON response. Register response Received Handler() as the XMLHttpRequest's load event handler.
Step 2: Display the quotes
Implement responseReceived Handler() to extract the quotes from the XMLHttpRequest response and display the quotes in an ordered
list. Each quote should be followed by a space, a dash, a space, and the source. You may find it helpful to repurpose the code in
showAnonymousQuotes() to create the quote list.
Ex: If the user chooses "love" and "3" and clicks Fetch Quotes, response Received Handler() should place the returned quotes in an
ordered list inside the <div>:
<div id="quotes">
<ol>
-
Hermann Hesse</li>
Elie Wiesel</li>
<li>If I know what love is, it is because of you.
<li>The opposite of love is not hate, it's indifference.
<li>Suffering passes, while love is eternal. Laura Ingalls Wilder</li>
</ol>
</div>
If an error message is received, the error message should be displayed in the <div>. Ex:
<div id="quotes">
Topic success' not found
</div>
-

Transcribed Image Text:Quote web API
A quote web API returns a collection of randomly selected quotes related to a given topic. The API supports two query string
parameters:
topic - Specifies the requested topic. Valid topics are love, motivational, wisdom, and humor.
⚫ count - Specifies the number of quotes requested and must be a number from 1 to 5.
Ex: The API request:
https://wp.zybooks.com/quotes.php?topic=love&count=3
returns 3 quotes about love, formatted in JSON:
{
},
{
},
{
"quote": "If I know what love is, it is because of you.",
"source": "Hermann Hesse"
"quote": "The opposite of love is not hate, it's indifference.",
"source": "Elie Wiesel"
"quote": "Suffering passes, while love is eternal.",
"source": "Laura Ingalls Wilder"
If the topic is not given or not recognized, the API returns an error message.
Ex: The request for a "success" quote:
https://wp.zybooks.com/quotes.php?topic=success&count=1
returns:
{
'error": "Topic 'success' not found"
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
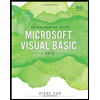
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
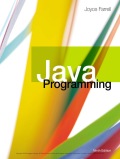
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
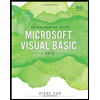
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
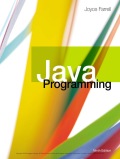
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT