So I have a p5js code that plays two diffrent audios when you click on two diffrent circles: let sound1, sound2; function preload() { sound1 = loadSound('Monster.mp3'); sound2 = loadSound('Blink.mp3'); } function setup() { createCanvas(400, 400); } function draw() { background(220); fill(0, 0, 255); ellipse(width/2, height/2, 100); fill(255, 0, 0); ellipse(width/4, height/4, 50); } function mouseClicked() { if (dist(mouseX, mouseY, width/2, height/2) < 50) { sound1.play(); } if (dist(mouseX, mouseY, width/4, height/4) < 25) { sound2.play(); } } And it works fine on its own. However, when I add it in my projects code with other aspects, the error, TypeError: undefined is not an object (evaluating 'sound1.play') and TypeError: undefined is not an object (evaluating 'sound2.play'), always pops up. Here it is, included in my projects code: let song; let img; var img2; var img3; var Frog, Herbs, Monster, Produce, Witch, Cauldron; //oval potion var x = 540; var y = 110; var w = 30; var h = 70; var radius = 20; //sound effects let sound1, sound2; function preload() { sound1 = loadSound('Monster.mp3'); sound2 = loadSound('Blink.mp3'); } function preload (){ img = loadImage('Door.jpg'); img3 = loadImage('Window.PNG'); Witch = createImg('Witch.gif',''); Cauldron = createImg('Cauldron.gif', ''); Herbs = createImg('Herbs.gif', ''); Monster = createImg('Monster.gif',''); Produce = createImg('Produce.gif',''); Frog = createImg('Frog.gif',''); img2 = loadImage('Wand.jpg'); } function setup() { createCanvas(700, 400); //background music song = createAudio('Music.mp3'); song.autoplay(true); song.loop(); song.volume(0.2); //se } function draw() { background(108, 93, 81); strokeWeight(6); //carpet {push(); fill(87, 47, 59); quad(79, 380, 152,300, 622,300, 550, 380); pop();} //right counter {push(); fill(37, 23, 30); square(620,190,80); rect(360,150,200,80); fill(37, 23, 30); quad(619,270,620,190,562,151,560,230); fill(0); beginShape(); vertex(360,150); vertex(360, 135); vertex(620, 135); vertex(700, 190); vertex(620,190); vertex(562,150); endShape(); pop();} //wall outline { line(620,135,620,5); line(360,230,80,230);} //shelfs {push(); fill(0); stroke(0); line(230,100,340,100); line(230,70,340,70); line(230,40,340,40); pop();} //potions {strokeWeight(1.5); push(); fill(200); rect(245,19,15,20); pop(); push(); fill(255,127,140); rect(268,24,20,15); pop(); push(); fill(75); rect(305,14,23,25); pop(); push(); fill(255,206,108); rect(252,54,30,15); pop(); push(); fill(139,255,121); rect(293,49,15,20); pop(); push(); fill(160); rect(240,81,23,18); pop(); push(); fill(108,195,255); rect(285,84,40,15); pop(); push(); fill(225,141,78); rect(250,15,5,5); rect(276,20,5,5); rect(310,10,5,5); rect(270,50,5,5); rect(298,45,5,5); rect(249,77,5,5); rect(290,80,5,5); pop();} //counter potions //{ellipse(x,y,w,h); //push() //strokeWeight(1); //fill(225,141,78); //rect(532,68,16,10); //pop();} //hangers {push(); stroke(0); fill(0); ellipse(250,120,10,10) ellipse(285,120,10,10); ellipse(320,120,10,10); pop();} //left counter {push(); fill(37, 23, 30); quad(0,365,80,260,80,151,0,255); fill(0); beginShape(); vertex(0,255); vertex(77, 150); vertex(0, 150); endShape(); pop();} //menu {push(); fill(117, 133, 101); rect(20,20,60,100); pop();} //window image(img3,400,30); //witch Witch.position(350,60); //cauldron Cauldron.position(265,218); //frog Frog.position(25,310); //monster Monster.position(5,130); //door image(img,92,13,125,220); //produce {push(); strokeWeight(1); fill(255); rect(527,230,23,20); pop(); Produce.position(465,155);} //herbs Herbs.position(600,90); //wand cursor image(img2,mouseX,mouseY,15,50); //sound effects fill(0, 0, 255); ellipse(width/2, height/2, 100); fill(255, 0, 0); ellipse(width/4, height/4, 50); } function mouseClicked() { if (dist(mouseX, mouseY, width/2, height/2) < 50) { sound1.play(); } if (dist(mouseX, mouseY, width/4, height/4) < 25) { sound2.play(); } } It always points out sound1.play and sound2.play as undefined, how can I fix this?
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
So I have a p5js code that plays two diffrent audios when you click on two diffrent circles:
let sound1, sound2;
function preload() {
sound1 = loadSound('Monster.mp3');
sound2 = loadSound('Blink.mp3');
}
function setup() {
createCanvas(400, 400);
}
function draw() {
background(220);
fill(0, 0, 255);
ellipse(width/2, height/2, 100);
fill(255, 0, 0);
ellipse(width/4, height/4, 50);
}
function mouseClicked() {
if (dist(mouseX, mouseY, width/2, height/2) < 50) {
sound1.play();
}
if (dist(mouseX, mouseY, width/4, height/4) < 25) {
sound2.play();
}
}
And it works fine on its own. However, when I add it in my projects code with other aspects, the error, TypeError: undefined is not an object (evaluating 'sound1.play') and TypeError: undefined is not an object (evaluating 'sound2.play'), always pops up.
Here it is, included in my projects code:
let song;
let img;
var img2;
var img3;
var Frog, Herbs, Monster, Produce, Witch, Cauldron;
//oval potion
var x = 540;
var y = 110;
var w = 30;
var h = 70;
var radius = 20;
//sound effects
let sound1, sound2;
function preload() {
sound1 = loadSound('Monster.mp3');
sound2 = loadSound('Blink.mp3');
}
function preload (){
img = loadImage('Door.jpg');
img3 = loadImage('Window.PNG');
Witch = createImg('Witch.gif','');
Cauldron = createImg('Cauldron.gif', '');
Herbs = createImg('Herbs.gif', '');
Monster = createImg('Monster.gif','');
Produce = createImg('Produce.gif','');
Frog = createImg('Frog.gif','');
img2 = loadImage('Wand.jpg');
}
function setup() {
createCanvas(700, 400);
//background music
song = createAudio('Music.mp3');
song.autoplay(true);
song.loop();
song.volume(0.2);
//se
}
function draw() {
background(108, 93, 81);
strokeWeight(6);
//carpet
{push();
fill(87, 47, 59);
quad(79, 380, 152,300, 622,300, 550, 380);
pop();}
//right counter
{push();
fill(37, 23, 30);
square(620,190,80);
rect(360,150,200,80);
fill(37, 23, 30);
quad(619,270,620,190,562,151,560,230);
fill(0);
beginShape();
vertex(360,150);
vertex(360, 135);
vertex(620, 135);
vertex(700, 190);
vertex(620,190);
vertex(562,150);
endShape();
pop();}
//wall outline
{ line(620,135,620,5);
line(360,230,80,230);}
//shelfs
{push();
fill(0);
stroke(0);
line(230,100,340,100);
line(230,70,340,70);
line(230,40,340,40);
pop();}
//potions
{strokeWeight(1.5);
push();
fill(200);
rect(245,19,15,20);
pop();
push();
fill(255,127,140);
rect(268,24,20,15);
pop();
push();
fill(75);
rect(305,14,23,25);
pop();
push();
fill(255,206,108);
rect(252,54,30,15);
pop();
push();
fill(139,255,121);
rect(293,49,15,20);
pop();
push();
fill(160);
rect(240,81,23,18);
pop();
push();
fill(108,195,255);
rect(285,84,40,15);
pop();
push();
fill(225,141,78);
rect(250,15,5,5);
rect(276,20,5,5);
rect(310,10,5,5);
rect(270,50,5,5);
rect(298,45,5,5);
rect(249,77,5,5);
rect(290,80,5,5);
pop();}
//counter potions
//{ellipse(x,y,w,h);
//push()
//strokeWeight(1);
//fill(225,141,78);
//rect(532,68,16,10);
//pop();}
//hangers
{push();
stroke(0);
fill(0);
ellipse(250,120,10,10)
ellipse(285,120,10,10);
ellipse(320,120,10,10);
pop();}
//left counter
{push();
fill(37, 23, 30);
quad(0,365,80,260,80,151,0,255);
fill(0);
beginShape();
vertex(0,255);
vertex(77, 150);
vertex(0, 150);
endShape();
pop();}
//menu
{push();
fill(117, 133, 101);
rect(20,20,60,100);
pop();}
//window
image(img3,400,30);
//witch
Witch.position(350,60);
//cauldron
Cauldron.position(265,218);
//frog
Frog.position(25,310);
//monster
Monster.position(5,130);
//door
image(img,92,13,125,220);
//produce
{push();
strokeWeight(1);
fill(255);
rect(527,230,23,20);
pop();
Produce.position(465,155);}
//herbs
Herbs.position(600,90);
//wand cursor
image(img2,mouseX,mouseY,15,50);
//sound effects
fill(0, 0, 255);
ellipse(width/2, height/2, 100);
fill(255, 0, 0);
ellipse(width/4, height/4, 50);
}
function mouseClicked() {
if (dist(mouseX, mouseY, width/2, height/2) < 50) {
sound1.play();
}
if (dist(mouseX, mouseY, width/4, height/4) < 25) {
sound2.play();
}
}
It always points out sound1.play and sound2.play as undefined, how can I fix this?

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

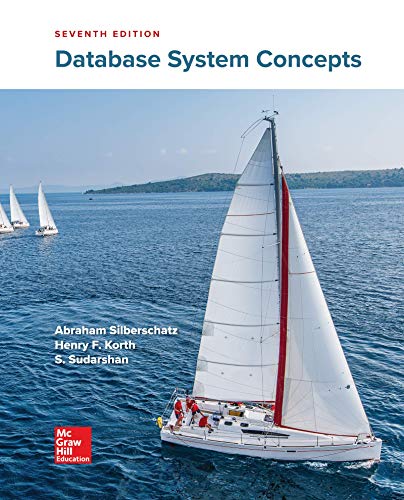
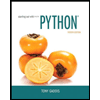
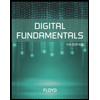
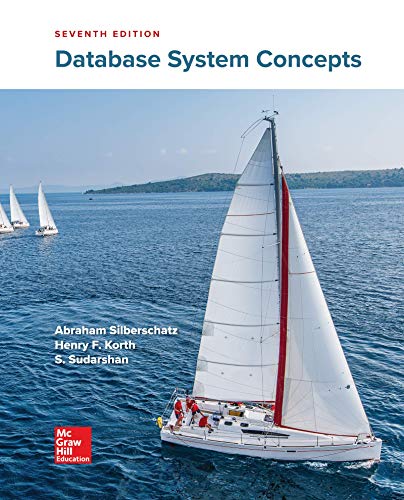
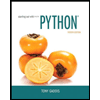
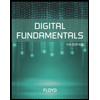
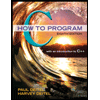
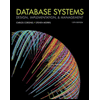
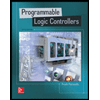