Suppose you are to design a payroll program where the user inputs the employee's name, number of hours worked, hourly pay rate, federal tax rate, and state tax rate and prints a summary of the employee's paycheck. Check the two sample runs and carefully read the instructions below. Two Sample Run: Enter employee's name: Smith Enter number of hours worked in a week: 10 Enter hourly pay rate: 9.75 Enter federal tax withholding rate: 0.2 Enter state tax withholding rate: 0.09 Employee Name: Smith Hours Worked: 10.00 Pay Rate: $9.75 Gross Pay: $97.50 Deductions: Net Pay: $69.23 ● ● Federal Withholding (20.00%): $19.50 State Withholding (9.00% ) : $8.78 Total Deduction: $28.28 ● ● Enter employee's name: John Enter number of hours worked in a week: 40 Enter hourly pay rate: 12.50 Enter federal tax withholding rate: 0.15 Enter state tax withholding rate: 0.08 Instructions: Prompt user for 5 values and read the values using Scanner O Use . nextLine() method to get the String for the name Use .nextDouble() method to get all other numeric values Employee Name: John Hours Worked: 40.00 Pay Rate: $12.50 Gross Pay: $500.00 Deductions: Federal Withholding (15.00%): $75.00 State Withholding (8.00%): $40.00 Total Deduction: $115.00 Net Pay: $385.00 O Calculating the Gross pay O Gross pay = hours worked * hourly pay rate Calculating the Federal withholding O Federal withholding = Gross pay* federal tax withholding rate Calculating the State withholding O State withholding = Gross pay* state tax withholding rate Calculating the Total deduction O Total deduction = Federal withholding+ State withholding Calculating the Net Pay O Net Pay = Gross pay- Total deduction Formatting the output same as the Sample run (Use ONLY printf) O Correctly format 2 decimals and show the dollar ($) sign. O Indenting (tab) the 3 items under Deductions. (Hint: \t) O Printing the percent (%) sign for state and federal withholding rate. Refer to the practice questions on the next page to help you code the program. Copy&Paste your code and screenshot your console output for the same 2 Sample Runs shown above.
Suppose you are to design a payroll program where the user inputs the employee's name, number of hours worked, hourly pay rate, federal tax rate, and state tax rate and prints a summary of the employee's paycheck. Check the two sample runs and carefully read the instructions below. Two Sample Run: Enter employee's name: Smith Enter number of hours worked in a week: 10 Enter hourly pay rate: 9.75 Enter federal tax withholding rate: 0.2 Enter state tax withholding rate: 0.09 Employee Name: Smith Hours Worked: 10.00 Pay Rate: $9.75 Gross Pay: $97.50 Deductions: Net Pay: $69.23 ● ● Federal Withholding (20.00%): $19.50 State Withholding (9.00% ) : $8.78 Total Deduction: $28.28 ● ● Enter employee's name: John Enter number of hours worked in a week: 40 Enter hourly pay rate: 12.50 Enter federal tax withholding rate: 0.15 Enter state tax withholding rate: 0.08 Instructions: Prompt user for 5 values and read the values using Scanner O Use . nextLine() method to get the String for the name Use .nextDouble() method to get all other numeric values Employee Name: John Hours Worked: 40.00 Pay Rate: $12.50 Gross Pay: $500.00 Deductions: Federal Withholding (15.00%): $75.00 State Withholding (8.00%): $40.00 Total Deduction: $115.00 Net Pay: $385.00 O Calculating the Gross pay O Gross pay = hours worked * hourly pay rate Calculating the Federal withholding O Federal withholding = Gross pay* federal tax withholding rate Calculating the State withholding O State withholding = Gross pay* state tax withholding rate Calculating the Total deduction O Total deduction = Federal withholding+ State withholding Calculating the Net Pay O Net Pay = Gross pay- Total deduction Formatting the output same as the Sample run (Use ONLY printf) O Correctly format 2 decimals and show the dollar ($) sign. O Indenting (tab) the 3 items under Deductions. (Hint: \t) O Printing the percent (%) sign for state and federal withholding rate. Refer to the practice questions on the next page to help you code the program. Copy&Paste your code and screenshot your console output for the same 2 Sample Runs shown above.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter6: Modularity Using Functions
Section6.4: A Case Study: Rectangular To Polar Coordinate Conversion
Problem 7E: (Simulation) Write a program to simulate the roll of two dice. If the total of the two dice is 7 or...
Related questions
Question

Transcribed Image Text:Suppose you are to design a payroll program where the user inputs the employee's name, number of hours worked,
hourly pay rate, federal tax rate, and state tax rate and prints a summary of the employee's paycheck. Check the
two sample runs and carefully read the instructions below.
Two Sample Run:
Enter employee's name: Smith
Enter number of hours worked in a week: 10
Enter hourly pay rate: 9.75
Enter federal tax withholding rate: 0.2
Enter state tax withholding rate: 0.09
Employee Name: Smith
Hours Worked: 10.00
Pay Rate: $9.75
Gross Pay: $97.50
Deductions:
Net Pay: $69.23
●
●
Federal Withholding (20.00%): $19.50
State Withholding (9.00% ) : $8.78
Total Deduction: $28.28
●
●
Enter employee's name: John
Enter number of hours worked in a week: 40
Enter hourly pay rate: 12.50
Enter federal tax withholding rate: 0.15
Enter state tax withholding rate: 0.08
Instructions:
Prompt user for 5 values and read the values using Scanner
O Use . nextLine() method to get the String for the name
Use .nextDouble() method to get all other numeric values
Employee Name: John
Hours Worked: 40.00
Pay Rate: $12.50
Gross Pay: $500.00
Deductions:
Federal Withholding (15.00%): $75.00
State Withholding (8.00%): $40.00
Total Deduction: $115.00
Net Pay: $385.00
O
Calculating the Gross pay
O Gross pay = hours worked * hourly pay rate
Calculating the Federal withholding
O Federal withholding = Gross pay* federal tax withholding rate
Calculating the State withholding
O State withholding = Gross pay* state tax withholding rate
Calculating the Total deduction
O Total deduction = Federal withholding+ State withholding
Calculating the Net Pay
O Net Pay = Gross pay- Total deduction
Formatting the output same as the Sample run (Use ONLY printf)
O Correctly format 2 decimals and show the dollar ($) sign.
O Indenting (tab) the 3 items under Deductions. (Hint: \t)
O Printing the percent (%) sign for state and federal withholding rate.
Refer to the practice questions on the next page to help you code the program.
Copy&Paste your code and screenshot your console output for the same 2 Sample Runs shown above.
AI-Generated Solution
Unlock instant AI solutions
Tap the button
to generate a solution
Recommended textbooks for you
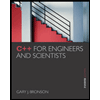
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
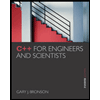
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage