The following is Java pseudocode. For this exercise, you can assume the input function provides an appropriate integer value. Line numbers are provided on the left. System.out.print will print the information to the same line. System.out.println will print the information and then go to the next line. // is a comment. Do not worry about public static. Code execution starts with the main method. For each method used, make sure to provide a separate table showing what the values are and how they change (i.e. tracing through the code). All variables passed in have values. Don’t worry about showing how the input() method works. 7 public static int test1(int x1, int x2){ 8 9 if(x1==0){ 10 x1=10; 11 } 12 if(x2==0){ 13 x2=10 14 } 15 16 return x1*x2; 17 } 18 19 public static int test2(int x2, int x1){ 20 return x2-x1; 21 } 22 public static int input(){ //Don’t need to trace through //this method to show how it works. Just assume it pulls //the value for the user. 23 Scanner keyboard = new Scanner(new BufferedReader(new InputStreamReader(System.in))); 24 int counter=0; 25 System.out.print("Please enter an integer: "); 26 while(counter<3 && !keyboard.hasNextInt()) 27 { 28 System.out.println(); 29 String temp=keyboard.next(); 30 System.out.println(temp+" is not an integer. Please reenter an integer."); 31 counter++; 32 33 } 34 return keyboard.nextInt(); 35 } 36 37 38 39 public static void doesSomething(int a, int c, int b){ 40 41 while(a
Part 4: Methods
The following is Java pseudocode. For this exercise, you can assume the input function provides an appropriate integer value. Line numbers are provided on the left. System.out.print will print the information to the same line. System.out.println will print the information and then go to the next line. // is a comment. Do not worry about public static. Code execution starts with the main method.
For each method used, make sure to provide a separate table showing what the values are and how they change (i.e. tracing through the code). All variables passed in have values. Don’t worry about showing how the input() method works.
7 public static int test1(int x1, int x2){
8
9 if(x1==0){
10 x1=10;
11 }
12 if(x2==0){
13 x2=10
14 }
15
16 return x1*x2;
17 }
18
19 public static int test2(int x2, int x1){
20 return x2-x1;
21 }
22 public static int input(){ //Don’t need to trace through
//this method to show how it works. Just assume it pulls
//the value for the user.
23 Scanner keyboard = new Scanner(new BufferedReader(new InputStreamReader(System.in)));
24 int counter=0;
25 System.out.print("Please enter an integer: ");
26 while(counter<3 && !keyboard.hasNextInt())
27 {
28 System.out.println();
29 String temp=keyboard.next();
30 System.out.println(temp+" is not an integer. Please reenter an integer.");
31 counter++;
32
33 }
34 return keyboard.nextInt();
35 }
36
37
38
39 public static void doesSomething(int a, int c, int b){
40
41 while(a<c)
42 {
43 if(b<0){
44 a=a+c;
45 }
46 else{
47 a=a+b;
48 }
49
50 a=a+1;
51 }
52 System.out.println("a="+a+" c="+c+" b="+b);
53
54 }
55
56 public static void doesWhat(int a, int b, int c){
57
58 while(a<5)
59 {
60 if(b<0){
61 a=a+test1(a, c);
62 }
63 else{
64 a=a+test2(c, a);
65 }
66
67 a=a+1;
68 }
69 System.out.println("a="+a+" c="+c+" b="+b);
70
71 }
72
73
74 public static void main(String[] args)
75
76 { int x=input();
77 System.out.println(x);
78 int y=input();
79 System.out.println(y);
80 int z=input();
81 System.out.println(z);
82 doesSomething(x, y, z);
83 doesWhat(x, y, z);
84 //test1(a,a)
85 }
86 public static void test3(){
87 int x = 15;
88 do
89 {
90 x += 3;
91 }
92 while (x < 25);
93 System.out.println(x);
94 }
Instructor Example 1: user input: x=5, y=7, z=2
MAIN |
||
x |
y |
z |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
**Remember to include additional tables with the name of the method and their appropriate variables.
Instructor Example 2: user input: x= -10, y= -3, z=0
MAIN |
||
x |
y |
z |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
**Remember to include additional tables with the name of the method and their appropriate variables.
Methods Test Case 1: user input: x=31, y= -15, z=0
MAIN |
||
x |
y |
z |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
**Remember to include additional tables with the name of the method and their appropriate variables.
Methods Test Case 2: user input: x=0, y= -4, z= 0
MAIN |
||
x |
y |
z |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
**Remember to include additional tables with the name of the method and their appropriate variables.
Optional Methods Test Case 3: user input: x=2, y=2, z=2
MAIN |
||
x |
y |
z |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
**Remember to include additional tables with the name of the method and their appropriate variables.

Step by step
Solved in 5 steps with 3 images

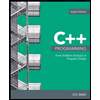
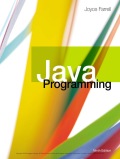
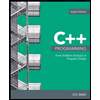
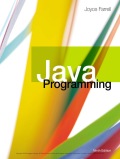
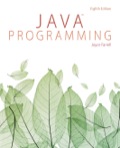