The topic of this homework is operator overloading. The class ElectronicDevice is given with the corresponding functions as show below. class ElectronicDevice { friend std::ostream& operator<<(std::ostream&, const ElectronicDevice& device_ref); public: ElectronicDevice(const std::string& name_ref, double consumption, double price, bool repairable) : name_(name_ref), consumption_(consumption), price_(price), repairable_(repairable){}; double operator+(const ElectronicDevice& rhs_ref) const; double operator-(const ElectronicDevice& rhs_ref) const; ElectronicDevice operator<(const ElectronicDevice& rhs_ref) const; std::string name() const { return name_; }; double consumption() const { return consumption_; }; double price() const { return price_; }; bool repairable() const { return repairable_; }; private: std::string name_; double consumption_; double price_; bool repairable_; }; A short main() program is also provided. All necessary libraries have been included. int main(void) { ElectronicDevice television("Sony", 100.0, 899.99, false), smartphone("GalaxyS9", 10.5, 799.99, true); std::cout << television; std::cout << television + smartphone << std::endl; std::cout << television - smartphone << std::endl; std::cout << (television < smartphone).name(); return 0; } Task: Overload the stream insertion operator (<<), a friend function is to be used. It displays the ElectronicDevice's name (name_), its price (price_) and its power consumption (consumption_). In addition, if repairable_ == true, the text "repairable" is to be displayed. If repairable_ == false, no output should be made. The output values should be separated by a blank character. The output should end with a new line (std::endl). Example for output of television in main(): Sony 899.99 100
The topic of this homework is operator overloading. The class ElectronicDevice is given with the corresponding functions as show below.
class ElectronicDevice
{
friend std::ostream& operator<<(std::ostream&, const ElectronicDevice& device_ref);
public:
ElectronicDevice(const std::string& name_ref, double consumption, double price, bool repairable)
: name_(name_ref), consumption_(consumption), price_(price), repairable_(repairable){};
double operator+(const ElectronicDevice& rhs_ref) const;
double operator-(const ElectronicDevice& rhs_ref) const;
ElectronicDevice operator<(const ElectronicDevice& rhs_ref) const;
std::string name() const { return name_; };
double consumption() const { return consumption_; };
double price() const { return price_; };
bool repairable() const { return repairable_; };
private:
std::string name_;
double consumption_;
double price_;
bool repairable_;
};
A short main() program is also provided. All necessary libraries have been included.
int main(void)
{
ElectronicDevice television("Sony", 100.0, 899.99, false), smartphone("GalaxyS9", 10.5, 799.99, true);
std::cout << television;
std::cout << television + smartphone << std::endl;
std::cout << television - smartphone << std::endl;
std::cout << (television < smartphone).name();
return 0;
}
Task:
Overload the stream insertion operator (<<), a friend function is to be used. It displays the ElectronicDevice's name (name_), its price (price_) and its power consumption (consumption_). In addition, if repairable_ == true, the text "repairable" is to be displayed. If repairable_ == false, no output should be made. The output values should be separated by a blank character. The output should end with a new line (std::endl).
Example for output of television in main(): Sony 899.99 100

Step by step
Solved in 4 steps with 2 images

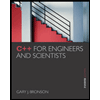
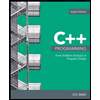
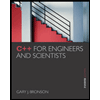
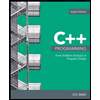