to assess the student’s ability to provide
I'm asking for help with different perspectives on purpose. Also, you do not have to complete the code, I’m posting the entire question in order for the question to be clear. Sorry if you see this question ask more than once.
Objectives:
The main objective of this assignment is to assess the student’s ability to provide a complete Black-Box testing plan for a computer
Description:
you will need to design a complete Black-Box testing plan for the following program. Instead of changing passwords every semester, students will now change passwords once a month. Unfortunately, the school has realized that many students are bad at picking their passwords. Ideally, passwords alternate between letters, numbers, and symbols. However, most students and faculty pick passwords such as “12345password”. To make matters worse, when students and faculty are forced to change their password, usually only a single character is added to the end of their previous password. Due to this problem, the University has created a program to help students and faculty create strong, new passwords. Although the program has been created, it has not been tested. You are tasked with created a complete Black-Box testing plan for the program. The password generation program is somewhat simple. First, the user will enter their last name. In this program, students that have last names beginning with A-M will change their passwords between the 15th and the 20th of every month. Students that have last names beginning with N-Z will change their passwords between the 20th and the 25th of every month. Next the user will input a multi-digit integer. Afterwards, the user will input a string. Lastly the user will select how many passwords they would like to generate with this information. The program then uses this information to randomly generate passwords using this data. The program will not execute if the current date is not correct. Using this information, please create a Black-Box testing plan for the program.
Task:
You must design a complete Black-Box testing plan for the given program. Your task is to apply what you have learned about black-box testing techniques to develop a full suite of test data for this program.
** I have access to “passwordgeneration.cbp,.depend, and .layout…. however I cannot access it at the moment. let me know if this would help.
- main.cpp attached
-solution template attached
![#include <iostream>
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <string>
using namespace std;
int main()
{
int numPasswords = 0;
int randNum = 0;
int originalLength
int month = 0;
int day = 0;
char trash =
= 0;
';
char firstLetter
string inputInt =
string inputstring =
string allInput =
string tmpInput =
string password = "";
string lastName =
cout << "Please enter your last name" « endl;
cin >> lastName;
cout <« "Please enter the month and day i.e.(1/25)" << endl;
cin >> month >> trash >> day;
srand (time ( NULL));
for (int i = '0; i < numPasswords; i++)
{
originalLength = tmpInput.length();
for (int j = 0; j < originalLength; j++)
{
randNum = rand() % tmpInput.length ( );
password += tmpInput[randNum];
tmpInput.erase (tmpInput.begin ()+randNum);
}
cout << "Password
password = "";
tmpInput = allInput;
}
Second Half of main.cpp
firstLetter = lastName[0];
if( (firstLetter > 64 && firstLetter < 78) || (firstLetter > 96 && firstLetter < 110))
{
if (day > 14 && day < 21)
cout << "Date Verified" << endl;
else
{
cout <« "Error: Names that begin with A-M register between the 15th and the 20th!" « endl;
return 1;
}
}
else if( (firstLetter > 77 && firstLetter < 91) || (firstLetter > 108
{
if (day > 19 && day < 26)
cout << "Date Verified" << endl;
<« i « ":\t" << password << endl;
%3D
firstLetter < 123))
return 0;
}
else
{
cout <« "Error: Names that begin with N-Z register between the 20th and the 25th!" « endl;
return 1;
}
else
{
cout << "Invalid last name!" << endl;
return 1;
}
cout <« "Please enter a multi-digit integer" << endl;
cin >> inputInt;
cout << "Please enter a string" << endl;
cin >> inputString;
cout << "How many passwords would you like to generate?" << endl;
cin >> numPasswords;
cout << "\n\n";
allInput = inputInt + inputString;
tmpInput = inputInt + inputString;
%3D](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6d702b96-16c9-42c6-953e-90c469a3b56f%2Fa9f49a99-30bb-41db-919b-dfd3da5f0e0c%2Ffhvt15_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

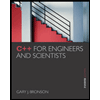
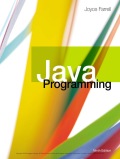
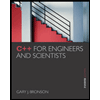
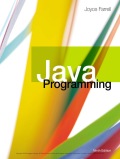