Use java programming language 1.1 Create a Student Object project. Create a Student class and use the provided UML diagram to code the instance fields, constructors and methods. Note: The module mark is the average of the participation mark and the examination mark - calculate this using the calcModuleMark() method. The determineStNumber() method should compile a student number with the first 3 letters of the student's name, a '#' symbol, and a 3-digit randomly generated number (make use of a for-loop to concatenate these 3-digits). The stNumber data field should be set in the constructor by calling the determineStNumber() method. The toString() method must compile a string to display the student number, participation mark, examination mark, and module mark, formatted in table format. Student number PMark EMark MMark Mar#9186 81 64 72.00 1.2 Create a testStudents class. Do the following: -Write a static method called display() which receives the Student object as a parameter, and displays the necessary heading, as well as the toString() method from the Object class. -In the main method, you should ask for the following input: the student's name, participation mark and an examination mark. -Make use of a while-loop to input multiple student details, and use a sentinel control to stop input. -After each student's details have been entered, instantiate an object of the Student class by calling the classes constructor. -Use the display() method to display the student's results before entering another student's details. -See the example of output below: Enter the name of a student: Mary Enter the student's participation mark: 81 Enter the student's examination mark: 64 Student number PMark EMark MMark Mar#9186 81 64 72.00 Enter the name of another student: (X to stop): Peter Enter the student's participation mark: 42 Enter the student's examination mark: 67 Student number PMark EMark MMark Pet#9043 42 67 54.00 Enter the name of another student: (X to stop): John Enter the student's participation mark: 55 Enter the student's examination mark: 70 Student number PMark EMark MMark Joh#1726 55 70 62.00 Enter the name of another student: (X to stop): x
Use java programming language
1.1 Create a Student Object project.
Create a Student class and use the provided UML diagram to code the instance fields, constructors and methods.
Note:
The module mark is the average of the participation mark and the examination mark - calculate this using the calcModuleMark() method.
The determineStNumber() method should compile a student number with the first 3 letters of the student's name, a '#' symbol, and a 3-digit randomly generated number (make use of a for-loop to concatenate these 3-digits).
The stNumber data field should be set in the constructor by calling the determineStNumber() method.
The toString() method must compile a string to display the student number, participation mark, examination mark, and module mark, formatted in table format.
Student number PMark EMark MMark
Mar#9186 81 64 72.00
1.2 Create a testStudents class. Do the following:
-Write a static method called display() which receives the Student object as a parameter, and displays the necessary heading, as well as the toString() method from the Object class.
-In the main method, you should ask for the following input: the student's name, participation mark and an examination mark.
-Make use of a while-loop to input multiple student details, and use a sentinel control to stop input.
-After each student's details have been entered, instantiate an object of the Student class by calling the classes constructor.
-Use the display() method to display the student's results before entering another student's details.
-See the example of output below:
Enter the name of a student: Mary
Enter the student's participation mark: 81
Enter the student's examination mark: 64
Student number PMark EMark MMark
Mar#9186 81 64 72.00
Enter the name of another student: (X to stop): Peter
Enter the student's participation mark: 42
Enter the student's examination mark: 67
Student number PMark EMark MMark
Pet#9043 42 67 54.00
Enter the name of another student: (X to stop): John
Enter the student's participation mark: 55
Enter the student's examination mark: 70
Student number PMark EMark MMark
Joh#1726 55 70 62.00
Enter the name of another student: (X to stop): x


Step by step
Solved in 5 steps with 5 images

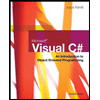
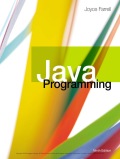
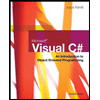
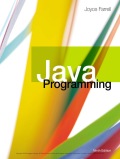