write a C++ program that consumes integer values as command line arguments and returns the arithmetic mean of these values. To increase the flexibility of the program, there should be no set number of arguments. To overcome this, we will require the argument argv[1] to be the number of integers the user enters. For example, if the user wants to calculate the arithmetic mean of 4 numbers, they would pass in 4 as the first argument and then the 4 numbers as the remaining arguments.
- Let’s experiment1 with command line arguments some more. Suppose we need integers as command line arguments. We will need to use the atoi() function provided in h to convert the char* argv[] values to integers. atoi() stands for “ASCII to int.” Here is an example
program that prints the sum of three integers entered as command line arguments.
#include <iostream>
#include <stdlib.h>
using namespace std;
void printUsage(char exeName[])
{
cout << "\n\tUsage: " << exeName << " [int] [int] [int]\n";
cout << "\n\tReturns the sum of the 3 int command line arguments.\n\n";
}
int main(int argc, char* argv[])
{
if (argc != 4)
{
printUsage(argv[0]);
return 1;
}
int a = atoi(argv[1]);
int b = atoi(argv[2]);
int c = atoi(argv[3]);
int sum = a + b + c;
cout << sum << endl;
return 0;
}
A more elegant implementation would be:
int sum = 0;
for (int i = 1; i < 4; i++)
{
sum += atoi(argv[i]);
}
Your task is to write a C++ program that consumes integer values as command line arguments and returns the arithmetic mean of these values. To increase the flexibility of the program, there should be no set number of arguments. To overcome this, we will require the argument argv[1] to be the number of integers the user enters. For example, if the user wants to calculate the arithmetic mean of 4 numbers, they would pass in 4 as the first argument and then the 4 numbers as the remaining arguments. Here is an example of how the program should look in execution:
In this example, avg is the name of the executable and the user wants the average of 4 numbers, which are 5, 9, 2, and 11. The program then prints the arithmetic mean (average). The benefits of command line arguments should now be obvious. The user simply enters in the name of the executable program followed by the arguments. This saves time for the user by reducing interactivity. It also enables programs to be more easily scripted for automating tasks.
Include a usage statement as shown in the above addition example. Since we will need to perform division between two integers, be cautious of type casting. Your program must make use of functions. Show your full source code and a screenshot of your running program.
![. C:\WINDOWS\system32\cmd.exe
C:\Users\Adam\Deskt op\CYBR210 Code>count
Usage: count [-option] [filename]
Opt ions :
[-1]
[-wi
lettercount
wordcount
C:\Users\Adam\Desktop\CYBR210 Code>count -1 hi.txt
Lettercount : 8
C: \Users\Adam\DesktopCYBR210 Code>count -w hi.txt
Wordcount : 3
C: \Users\Adam\DesktopCYBR210 Code>_
>](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F851a813d-a7ba-41bf-bed6-32eb13d55094%2Fd48dcacf-c38a-4976-910e-f07479070d9d%2Fzrrbu0n_processed.png&w=3840&q=75)
![C: \Users \AdamDesktop\CYBR210 Code>add 4 5
Usage: add Cint] Cint] Cint ]
Returns the sum of the 3 int command line arguments.
C:\Users \AdamDesktop\CYBR210 Code>add 4 59
18](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F851a813d-a7ba-41bf-bed6-32eb13d55094%2Fd48dcacf-c38a-4976-910e-f07479070d9d%2Fbpuu8j_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

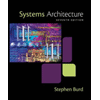
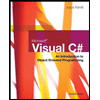
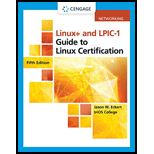
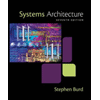
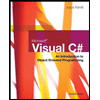
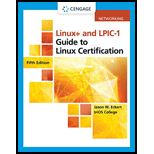