Write a function costume_rating that takes in two parameters: costume_color (a string) - the color of your costume. • bling_value (a positive integer) - represents how shiny your costume is. First, you need to convert the costume_color to a value based on the table below costume_color blue red green yellow purple orange numerical value 7 3 9 0 This function should return an inner function that takes one parameter: • phrase (a string) - the phrase said by a trick-or-treater. and returns the score (as an int, by flooring the result) based on the following formula: The final formula for the number of candies to return is the following >>> costume_rater ('seven c') 1 5 2 floor(- (costume color value × bling value) (length of phrase)/10 10 def costume_rating (costume_color, bling_value): "" >>> costume_rater = costume_rating('blue', 5) >>> costume_rater ('19 Character Phrase') 85 >>> costume_rater ('e') 0 >>> costume_rater = costume_rating('orange', 8) >>> costume_rater('hello i want candy') 14 >>> costume_rater = costume_rating('yellow', 5) >>> costume_rater('please give me candy i really need candy') 0
# Question 4
def costume_rating(costume_color, bling_value):
"""
Returns a function that calculates the number of candies to return based on
the costume color, bling value, and phrase length.
Args:
costume_color (str): The color of your costume. Must be one of 'blue',
'red', 'green', 'yellow', 'purple', or 'orange'.
bling_value (int): Represents how shiny your costume is. Must be a
positive integer.
Returns:
A function that takes in one parameter:
phrase (str): The phrase said by the trick-or-treater.
>>> costume_rater = costume_rating('blue', 5)
>>> costume_rater('19 Character Phrase')
85
>>> costume_rater('e')
0
>>> costume_rater('seven c')
1
>>> costume_rater = costume_rating('orange', 8)
>>> costume_rater('hello i want candy')
14
>>> costume_rater = costume_rating('yellow', 5)
>>> costume_rater('please give me candy i really need candy')
0
"""
# YOUR CODE GOES HERE #
color_values = {
'blue': 7,
'red': 3,
'green': 9,
'yellow': 0,
'purple': 5,
'orange': 2
}
assert costume_color in color_values, "Invalid costume color"
def inner_function(phrase):
return int((costume_color_value - bling_value) * len(phrase) / 1010)
return inner_function
fix the forumla


Step by step
Solved in 4 steps with 1 images

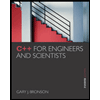
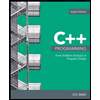
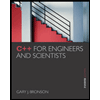
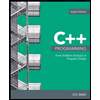