Write a Java program that stores information about a company. You should define two classes: Employee which represents the employees in the company, and Department which represents the departments the employees are in. Class definition The classes should have the following fields: Department String name Employee String firstName String lastName double salary Department department Notes: 1. All fields should be private. 2. You should define a constructor that takes as parameters all fields in each class. 3. You should define a getter and a setter for all fields in each class. 4. The field salary on class Employee should never be a negative number. 5. You should define a method getFullName on class Employee that returns the employees full name as a String. Main Program after you have defined the classes, In the main method define two objects of type Department as follows: # name 1 Sales 2 Accounting Then define an array of type Employee and fill it with the following Employee objects: firstName lastName salary department Department object 1 (Sales) Department object 1 (Sales) Department object 1 (Sales) Department object 2 (Accounting) Department object 2 (Accounting) 1 John White 1000 2 David Ford 1500 Sophie Mary Веech 1200 4 Howe 1300 Susan Brand 1400 the program should show the user the following menu and wait for input from the user: 1. Show all employees 2. Show employees for department 3. Show employee with max salary Option 1: shows the employee's full name, salary and department name for all employees 1. show all employees 2. show employees for department 3. show employee with max salary 1 Name Salary Department John White 1000.0 Sales Sophie Beech 1200.0 Sales David Ford 1500.0 Sales Mary Howe 1300.0 Accounting Susan Brand 1400.0 Accounting Process finished with exit code 0 Option 2: asks the user for a department name then shows the employee's full name, salary and department name for that department.
Write a Java program that stores information about a company. You should define two classes: Employee which represents the employees in the company, and Department which represents the departments the employees are in. Class definition The classes should have the following fields: Department String name Employee String firstName String lastName double salary Department department Notes: 1. All fields should be private. 2. You should define a constructor that takes as parameters all fields in each class. 3. You should define a getter and a setter for all fields in each class. 4. The field salary on class Employee should never be a negative number. 5. You should define a method getFullName on class Employee that returns the employees full name as a String. Main Program after you have defined the classes, In the main method define two objects of type Department as follows: # name 1 Sales 2 Accounting Then define an array of type Employee and fill it with the following Employee objects: firstName lastName salary department Department object 1 (Sales) Department object 1 (Sales) Department object 1 (Sales) Department object 2 (Accounting) Department object 2 (Accounting) 1 John White 1000 2 David Ford 1500 Sophie Mary Веech 1200 4 Howe 1300 Susan Brand 1400 the program should show the user the following menu and wait for input from the user: 1. Show all employees 2. Show employees for department 3. Show employee with max salary Option 1: shows the employee's full name, salary and department name for all employees 1. show all employees 2. show employees for department 3. show employee with max salary 1 Name Salary Department John White 1000.0 Sales Sophie Beech 1200.0 Sales David Ford 1500.0 Sales Mary Howe 1300.0 Accounting Susan Brand 1400.0 Accounting Process finished with exit code 0 Option 2: asks the user for a department name then shows the employee's full name, salary and department name for that department.
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter11: More Object-oriented Programming Concepts
Section: Chapter Questions
Problem 1GZ
Related questions
Question

Transcribed Image Text:Homework 2
Write a Java program that stores information about a company. You should define two classes:
Employee which represents the employees in the company, and Department which represents
the departments the employees are in.
Class definition
The classes should have the following fields:
Department
• String name
Employee
• String firstName
• String lastName
• double salary
Department department
Notes:
1. All fields should be private.
2. You should define a constructor that takes as parameters all fields in each class.
3. You should define a getter and a setter for all fields in each class.
4. The field salary on class Employee should never be a negative number.
5. You should define a method getFullName on class Employee that returns the
employees full name as a String.
Main Program
after you have defined the classes, In the main method define two objects of type Department
as follows:
#
name
Sales
2
Accounting
Then define an array of type Employee and fill it with the following Employee objects:
firstName
lastName
salary
1000
department
Department object 1 (Sales)
Department object 1 (Sales)
Department object 1 (Sales)
Department object 2 (Accounting)
Department object 2 (Accounting)
#
1
John
White
2
David
Ford
1500
3
|Sophie
Beech
1200
1300
1400
4
Mary
Susan
Howe
5
Brand
the program should show the user the following menu and wait for input from the user:
1. Show all employees
2. Show employees for department
3. Show employee with max salary
Option 1: shows the employee's full name, salary and department name for all employees
1. show all employees
2. show employees for department
3. show employee with max salary
1
Name
Salary Department
John White
1000.0
Sales
Sophie Beech
1200.0
Sales
David Ford
1500.0
Sales
Mary Howe
1300.0
Accounting
Susan Brand 1400.0
Accounting
Process finished with exit code 0
Option 2: asks the user for a department name then shows the employee's full name, salary
and department name for that department.

Transcribed Image Text:1. show all employees
2. show employees for department
3. show employee with max salary
input department name
Accounting
Name
Salary Department
Mary Howe
1300.0 Accounting
Susan Brand 1400.0 Accounting
Option 3: shows the full name, salary and department name for the employee with the
maximum salary in the company
1. show all employees
2. show employees for department
3. show employee with max salary
3
Name
Salary Department
David Ford 1500.0
Sales
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 7 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
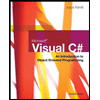
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
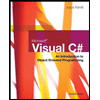
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,