• Write a JAVA program to build an appropriate implementation for the UML diagram below, which models an airline flight management system. AirLine - name: String - flights: ArrayList + AirLine(name: String, fileName: String) + sortFlightsNumOfPassengers(): void + listFlightsFromTo(origin: String, destination: String):void + reserveFlight (flightNumber: int): boolean + cancelReservation(flightNumber:int, ticketNo:int): void <> java.lang.Comparable + compareTof:Flight):int Flight - passengers: ArrayList
• Write a JAVA program to build an appropriate implementation for the UML diagram below, which models an airline flight management system. AirLine - name: String - flights: ArrayList + AirLine(name: String, fileName: String) + sortFlightsNumOfPassengers(): void + listFlightsFromTo(origin: String, destination: String):void + reserveFlight (flightNumber: int): boolean + cancelReservation(flightNumber:int, ticketNo:int): void <> java.lang.Comparable + compareTof:Flight):int Flight - passengers: ArrayList
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
It is a Java program

Transcribed Image Text:* Write a JAVA program to build an appropriate implementation for the UML diagram below, which models an
airline flight management system.
AirLine
- name: String
|- flights: ArrayList<Flight>
+ AirLine(name: String, fileName: String)
+ sortFlightsNumOfPassengers(): void
+ listFlightsFromTo(origin: String, destination: String):void
+ reserveFlight (flightNumber: int): boolean
+ cancelReservation(flightNumber:int, ticketNo:int): void
<<interface>>
java.lang.Comparable<Flight>
+ compareTo(f:Flight):int
Flight
- passengers: ArrayList<Passenger>
- departureTime: Date
- arrivalTime: Date
- origin: Airport
- destination: Airport
- number: int
Italic
Passenger
# name: String
- luggage: Luggage
- passNo: int
- ticketNo: int
- ticketPrice: double
normalPrice: double
+ Flight(AlIDataFieldsExceptPassengers)
+ addPassenger(): boolean
+ removePassenger(): boolean
+ Passenger(ticketNo:int, ticketPrice: double)
cancelTicket(): void
+ loungeAccess(): boolean
+ getLuggage(): Luggage
Italic
Airport
- code: String
- location: String
+ Airport(code:String, location:String)
+ getCode(): String
+ getLocation(): String
Economy
+ Economy( ticketNo:int, ticketPrice: double)
+ cancelTicket(): void
+ loungeAccess(): boolean
Luggage
- numOfBags: int=1
- weight: double-25
FirstClass
- extraLuggage: Luggage
+ FirstClass( ticketNo:int, ticketPrice: double)
+ cancelTicket(): void
+ lounge Access(): Boolean
+ getLuggage(): Luggage
+ Luggage()
+ Luggage(numOfBags:int, weight: double)
+ getNumOfBags(): int
+ getWeight(): double

Transcribed Image Text:4. Economy:
The constructor should invoke the super class constructor with the passed parameter ticketNo and ticketPrice.
Override the cancelTicket method such that it displays a dialog box with the message: "Please note that there's
no money refund for your ticket!".
a.
b.
Override the loungeAccess method such that it returns false.
d. Override the toString method such that it returns a String that begins with the word Economy, then the
passenger info retrieved using the super class toString method.
c.
5. FirstClass
The constructor should invoke the super class constructor with the passed parameter ticketNo and ticketPrice.
Then create a luggage object with default number of bags and weight for the extraluggage.
Override the cancelTicket method such that it displays a dialog box with the message: ""Please note you have
a refund amount ---ticketPrice-".
b. Override the loungeAccess method such that it returns true.
c. Override the toString method such that it returns a String that begins with the word FirstClass, then the
a.
е.
passenger info retrieved using the super class toString method.
Override the getLuggage method such that it returns a luggage object that consists of the summation of bags
and weight in the luggage and extraluggage data fields.
d.
6. Flight
The constructor should initialize the ArrayList such that it's empty, and initialize all other data fields with
corresponding arguments with same names.
The method addPassenger should ask the user about the type of ticket to buy: Economy or FirctClass as in the
following dialog box.
a.
b.
Input
? Enter number of choice:
1.Economy class
2.First class
OK
Cancel
Then create and add a passenger of the selected type to the ArrayList of passengers.
If the user enters an option other than 1 or 2 (another number), or press cancel, the following dialog box
should appear:
Error
X Sorry could not add passenger, incorrect input
OK
If the passenger is added successfully, the method returns true. Otherwise, it returns false.
The method removePassenger should ask the user to enter the ticket number and the name of the passenger,
then search for the passenger with the entered ticket number and passenger name (ignoring case sensitivity
when matching names).
If the passenger is found, it should invoke the cancelTicket method for that passenger, then remove him
from the passengers ArrayList, then return true.
If the passenger is not found, it should return false.
C.
d. Override the equals method such that two flights are equal if they are from the same origin airport and going
to the same destination airport. Note that you should compare airports by invoking the equals method of the
Airport class.
e. Override the compareTo method such that it returns 1 if the first flight has more passengers than the second,
O if they have the same number, and -1 if the first flight has less passengers than the second.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 6 steps

Recommended textbooks for you
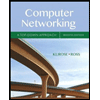
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
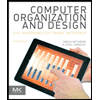
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
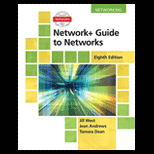
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
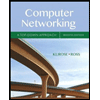
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
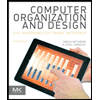
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
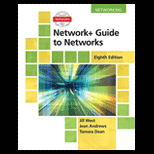
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
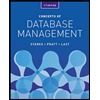
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
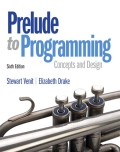
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
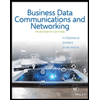
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY