Write a program that will read in N integers from keyboard input into an array. The size of the array N will be entered on the keyboard. The program will tabulate the output as shown below: the first column should show the list of distinct array elements sorted in ascending order, while the second column should show the number of occurrences of each element. Sample input: 9, 15, 7, 9, 3, 7, 3, 3,7, 6, 3, 20 Expected output: count 3. 4 6 1 7 3 15 1 20 1
This is my C++ Codes for the problem but it has errors when I run it. Can you fix the errors? Please still use header files iostream. This is a topic under Data Structures: Arrays.
//Start of program
#include <iostream>
#define TRUE 0
#define FALSE 1
using namespace std;
int main()
{
//Declaration of array
int A[50];
//Display the first element of the array A
cout << "The value of the first element of the array A is: " << A[0] << endl;
//Store the value 26 in 25th element of array A
A[24] = 26;
//Store the computed value in 10th element of array A
A[9] = (A[39] * 3) + 10;
cout << "The value of the 10th element of the array A is: " << A[9] << endl;
int n = 0; //Subscript of array A
//For loop
for (int i = 0; i < n; i++)
{
if (n / 2 == 0 || n / 3 == 0) //If subscript is a multiple of 2 or 3
{
cout << A[i] << " " << endl; //Display the value of an element of array A
}
if (n / 2 != 0 || n / 3 != 0) //If subscript is not a multiple of 2 or 3
break; //Break out of loop
}
//End of program
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

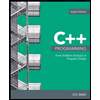
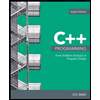