you are to implement a multithreaded program that will allow us tomeasure the performance (i.e., CPU utilization, Throughput, Turnaround time, and Waiting time inReady Queue) of the four basic CPU scheduling algorithms (namely, FIFO, SJF, PR, and RR). Yourprogram will be emulating/simulating the processes whose priority, sequence of CPU burst time(ms)and I/O burst time(ms) will be given in an input file.Your program will take the name of the scheduling algorithm, related parameters (if any), and aninput file name from command line. Here how your program should be executed:prog -alg [FIFO—SJF—PR—RR] [-quantum [integer(ms)]] -input [file name]The output of your program will be as follows:Input File Name : file nameCPU Scheduling Alg : FIFO—SJF—PR—RR (quantum)CPU utilization : ....Throughput : ....Avg. Turnaround time : ....Avg. Waiting time in R queue : ....Assume that all scheduling algorithms except RR will be non-preemptive, and all scheduling algorithmsexcept PR will ignore process priorities (i.e., all processes have the same priority in FIFO, SJF andRR). Also assume that there is only one IO device and all IO requests will be served using that devicein a FIFO manner.The input file is formatted such that each line starts with proc, sleep, stop keywords.Following proc, there will be a sequence of integer numbers: the first one represents the priority (1:lowest, ..., 5: normal, ..., 10: highest). The second number shows the number of remaning integersrepresenting CPU burst and I/O burst times (ms) in an alternating manner. The last number willbe the last CPU burst time after which that process exits. Following sleep, there will be an integernumber representing the time (ms) after which there will be another process.So one of the threads in your program (e.g., FileRead thread()) would be responsible for processingthis file as follows. As long as it reads proc, it will create a new process and put it in a ready queue(clearly this process is not an actual one, it will be just a simple data structure (similar to PCB) thatcontains the given priority and the sequence of CPU burst and I/O burst times, and other fields).When this thread reads sleep x, it will sleep x ms and then try to read new processes from the file.Upon reading stop, this thread will quit.Here is a sample input file (copy/paste this to create an input1.txt you can create other similar filestoo)proc 1 7 10 20 10 50 20 40 10proc 1 5 50 10 30 20 40sleep 50proc 2 3 20 50 20proc 3 3 20 50 20sleep 50proc 2 3 20 50 20stopIn this program you need at least three threads:As described above, one thread (say FileRead thread) will read the above file, create a process(not a real process just a data structure representing the characteristic of the process that willbe emulated/simulated), and puts it in a ready queue.CPU scheduler thread will check ready queue; if there is a process, it will pick one according tothe scheduling algorithm from ready queue and hold CPU resource for the given CPU burst time(or for quantum time if the scheduling algorithm is RR). That means CPU thread will simplysleep for the given CPU burst time to emulate/simulate the process. Then it will release CPUresource and put this process into IO queue (or ready queue if RR is used) or just terminate ifthis is the last CPU burst. Then CPU scheduler thread will check ready queue again and repeatthe same for the next process.I/O system thread will check IO queue; if there is a process, it will sleep for the given IO bursttime to emulate/simulate the usage of IO device. It then puts this process back into ready queue.Finally it will check IO queue and repeat the same
Oh no! Our experts couldn't answer your question.
Don't worry! We won't leave you hanging. Plus, we're giving you back one question for the inconvenience.
you are to implement a multithreaded program that will allow us to
measure the performance (i.e., CPU utilization, Throughput, Turnaround time, and Waiting time in
Ready Queue) of the four basic CPU scheduling algorithms (namely, FIFO, SJF, PR, and RR). Your
program will be emulating/simulating the processes whose priority, sequence of CPU burst time(ms)
and I/O burst time(ms) will be given in an input file.
Your program will take the name of the scheduling algorithm, related parameters (if any), and an
input file name from command line. Here how your program should be executed:
prog -alg [FIFO—SJF—PR—RR] [-quantum [integer(ms)]] -input [file name]
The output of your program will be as follows:
Input File Name : file name
CPU Scheduling Alg : FIFO—SJF—PR—RR (quantum)
CPU utilization : ....
Throughput : ....
Avg. Turnaround time : ....
Avg. Waiting time in R queue : ....
Assume that all scheduling algorithms except RR will be non-preemptive, and all scheduling algorithms
except PR will ignore process priorities (i.e., all processes have the same priority in FIFO, SJF and
RR). Also assume that there is only one IO device and all IO requests will be served using that device
in a FIFO manner.
The input file is formatted such that each line starts with proc, sleep, stop keywords.
Following proc, there will be a sequence of integer numbers: the first one represents the priority (1:
lowest, ..., 5: normal, ..., 10: highest). The second number shows the number of remaning integers
representing CPU burst and I/O burst times (ms) in an alternating manner. The last number will
be the last CPU burst time after which that process exits. Following sleep, there will be an integer
number representing the time (ms) after which there will be another process.
So one of the threads in your program (e.g., FileRead thread()) would be responsible for processing
this file as follows. As long as it reads proc, it will create a new process and put it in a ready queue
(clearly this process is not an actual one, it will be just a simple data structure (similar to PCB) that
contains the given priority and the sequence of CPU burst and I/O burst times, and other fields).
When this thread reads sleep x, it will sleep x ms and then try to read new processes from the file.
Upon reading stop, this thread will quit.
Here is a sample input file (copy/paste this to create an input1.txt you can create other similar files
too)
proc 1 7 10 20 10 50 20 40 10
proc 1 5 50 10 30 20 40
sleep 50
proc 2 3 20 50 20
proc 3 3 20 50 20
sleep 50
proc 2 3 20 50 20
stop
In this program you need at least three threads:
As described above, one thread (say FileRead thread) will read the above file, create a process
(not a real process just a data structure representing the characteristic of the process that will
be emulated/simulated), and puts it in a ready queue.
CPU scheduler thread will check ready queue; if there is a process, it will pick one according to
the scheduling algorithm from ready queue and hold CPU resource for the given CPU burst time
(or for quantum time if the scheduling algorithm is RR). That means CPU thread will simply
sleep for the given CPU burst time to emulate/simulate the process. Then it will release CPU
resource and put this process into IO queue (or ready queue if RR is used) or just terminate if
this is the last CPU burst. Then CPU scheduler thread will check ready queue again and repeat
the same for the next process.
I/O system thread will check IO queue; if there is a process, it will sleep for the given IO burst
time to emulate/simulate the usage of IO device. It then puts this process back into ready queue.
Finally it will check IO queue and repeat the same
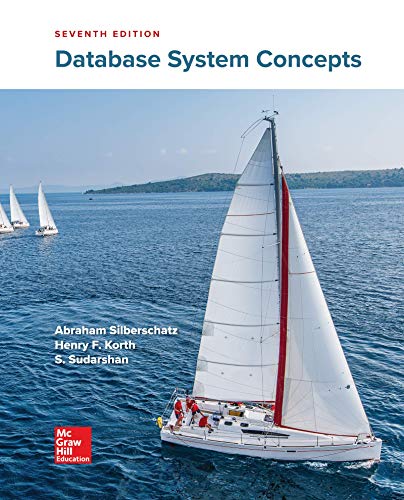
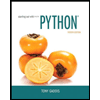
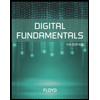
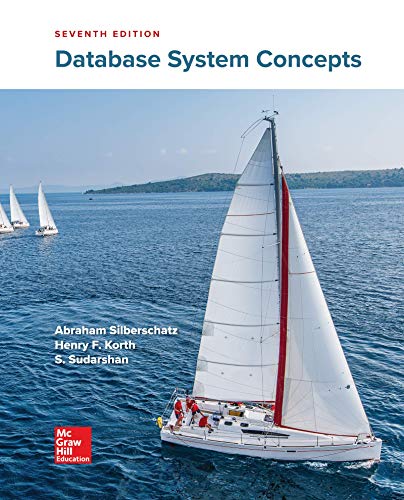
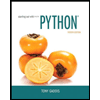
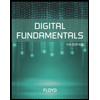
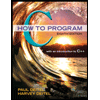
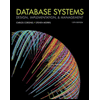
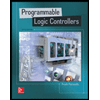