Define and implement a class named WordList similar to the MyArray class in the lectures the past 2 weeks. Your WordList class should have the following data members and methods: data members: String[] list : array of n words (assume 1 ≤ n ≤ 20) int n : number of words in list methods: 1. WordList() - constructor for WordList class 2. read() – read a sentence consisting of English words using a .nextLine() statement, break each word off the sentence, and save the words in array with one word in each array element keeping the relative order of the words unchanged. 3. print() – use the array contents to print the word list 4. sort() – sort the words in the array in alphabetical order using the insertion sort method. For example, if "To be or not to be, that is the question." is entered for the initial sentence: 1. read() will build array {"To", "be", "or", "not", "to", "be", "that", "is", "the", "question"} 2. print() will use the array contents to print: To be or not to be that is the question 3. sort() will sort the words in the array to {"be", "be", "is", "not", "or", "question", "that", "the", "To", "to"} You may assume input sentences consist of only alphabet letters, commas, periods and blanks. 1. You must use For loops. 2. You may not use the Array class or any of its methods. 3. If appropriate for this algorithm, you should validate any input from the user to make sure the data input is an appropriate value to work in your program’s logic. You don’t have to worry about validating that it is the correct data type. For now, assume the user is only giving you the correct data type and just worry about validating the value given is usable in your program.
Define and implement a class named WordList similar to the MyArray class in the lectures the
past 2 weeks. Your WordList class should have the following data members and methods:
data members:
String[] list : array of n words (assume 1 ≤ n ≤ 20)
int n : number of words in list
methods:
1. WordList() - constructor for WordList class
2. read() – read a sentence consisting of English words using a .nextLine() statement,
break each word off the sentence, and save the words in array with one word in each
array element keeping the relative order of the words unchanged.
3. print() – use the array contents to print the word list
4. sort() – sort the words in the array in alphabetical order using the insertion sort
method.
For example, if "To be or not to be, that is the question." is entered for the initial sentence:
1. read() will build array {"To", "be", "or", "not", "to", "be", "that", "is", "the",
"question"}
2. print() will use the array contents to print:
To be or not to be that is the question
3. sort() will sort the words in the array to {"be", "be", "is", "not", "or", "question",
"that", "the", "To", "to"}
You may assume input sentences consist of only alphabet letters, commas, periods and blanks.
1. You must use For loops.
2. You may not use the Array class or any of its methods.
3. If appropriate for this
sure the data input is an appropriate value to work in your program’s logic. You don’t
have to worry about validating that it is the correct data type. For now, assume the user
is only giving you the correct data type and just worry about validating the value given is
usable in your program.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

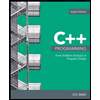
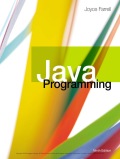
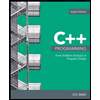
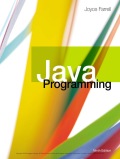