Implement your own indexOf(String s) and lastIndexOf(String s) methods without using the built-in ones available in Java String class. Complete the following program: //course: CSC190 //project: Lab 12 //date: (today’s date) //author: (your name) //purpose: import java.util.Scanner; class MyString { String s; //default constructor which sets s to a null string //your code here //additional constructor which sets s to the string passed from the caller //your code here //get and set methods for s //your code here //return the index within s of the first occurrence of str. //return -1 when str is not found on s. //if s = "aabababb", s = "aba", return 1 //if s = "aabababb", s = "abaa", return -1 int indexOf(String str) { //your code here } //return the index within s of the last occurrence of str. //return -1 when s is not found on str. //if s = "aabababb", s = "aba", return 3 //if s = "aabababb", s = "abaa", return -1 int lastIndexOf(String s) { //your code here } } class Lab12FirstnameLastname { public static void main(String [] args) { Scanner in = new Scanner(System.in); MyString myS = new MyString(); String s1, s2; //input variables System.out.print("Enter string 1:"); s1 = in.nextLine(); System.out.print("Enter string 2:"); s2 = in.nextLine(); myS.setS(s1); int p = myS.indexOf(s2); if (p == -1) System.out.println(s2+" not found on "+myS.getS()); else System.out.println(s2+" found at "+p+" on "+myS.getS()); p = myS.lastIndexOf(s2); if (p == -1) System.out.println(s2+" not found on "+myS.getS()); else System.out.println(s2+" found at "+p+" on "+myS.getS()); } You may not use any built in methods from the string class in the Java library other than charAt() and length()
Implement your own indexOf(String s) and lastIndexOf(String s) methods without using the built-in ones available in Java String class. Complete the following program:
//course: CSC190
//project: Lab 12
//date: (today’s date)
//author: (your name)
//purpose:
import java.util.Scanner;
class MyString {
String s;
//default constructor which sets s to a null string
//your code here
//additional constructor which sets s to the string passed from the caller
//your code here
//get and set methods for s
//your code here
//return the index within s of the first occurrence of str.
//return -1 when str is not found on s.
//if s = "aabababb", s = "aba", return 1
//if s = "aabababb", s = "abaa", return -1
int indexOf(String str) {
//your code here
}
//return the index within s of the last occurrence of str.
//return -1 when s is not found on str.
//if s = "aabababb", s = "aba", return 3
//if s = "aabababb", s = "abaa", return -1
int lastIndexOf(String s) {
//your code here
} }
class Lab12FirstnameLastname {
public static void main(String [] args) {
Scanner in = new Scanner(System.in);
MyString myS = new MyString();
String s1, s2; //input variables
System.out.print("Enter string 1:");
s1 = in.nextLine();
System.out.print("Enter string 2:");
s2 = in.nextLine();
myS.setS(s1);
int p = myS.indexOf(s2);
if (p == -1)
System.out.println(s2+" not found on "+myS.getS());
else
System.out.println(s2+" found at "+p+" on "+myS.getS());
p = myS.lastIndexOf(s2);
if (p == -1)
System.out.println(s2+" not found on "+myS.getS());
else
System.out.println(s2+" found at "+p+" on "+myS.getS());
}
You may not use any built in methods from the string class in the Java library other than charAt() and length()

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

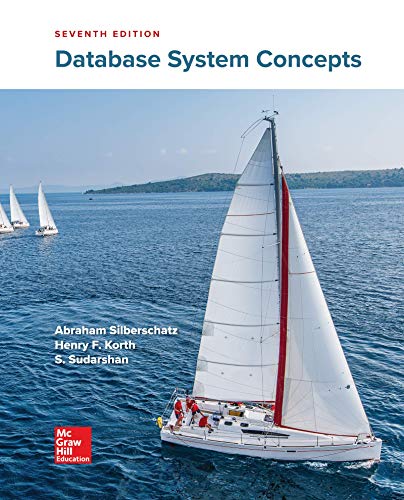
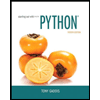
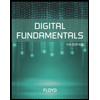
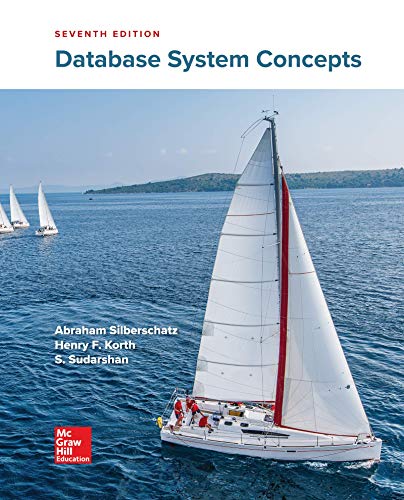
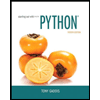
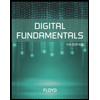
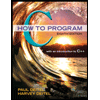
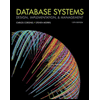
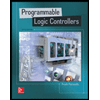