In Java Use inheritance to create the class PassengerPlane. An object of this class includes a model, range, and number of seats. Include a constructor, the appropriate get and set method(s), and a toString method that returns a string containing the model, range, and number of seats for the plan.
In Java
Use inheritance to create the class PassengerPlane. An object of this class includes a model, range, and number of seats. Include a constructor, the appropriate get and set method(s), and a toString method that returns a string containing the model, range, and number of seats for the plan.


Introduction of the Program:
The Java Program uses the concept of inheritance. Here the Airplane class has two fields and the Passenger Plane inherits the fields of the Airplane class and the passenger Plane class has one field and then the program displays the message using the toString method.
Source code of the Program: save the below code as Main.java
//Main.java
//Java program to illustrate the concept of inheritance
// base class
class Airplane
{
// the Airplane class has two fields
private String model;
private double range;
// the Airplane class constructor
public Airplane(String nModel, double nRange) {
this.model = nModel;
this.range = nRange;
}
public String getModel(){return model;}
public double getRange(){return range;}
// toString() method to print info of Bicycle
public String toString()
{
return("Airplane model "+model
+"\n"
+ "Range "+range+" miles");
}
}
// derived class PassengerPlane inherits Airplane class
class PassengerPlane extends Airplane
{
// the PassengerPlane subclass adds one more field
public int seat;
// the PassengerPlane subclass has one constructor
public PassengerPlane(String model,double range,
int startHeight)
{
// invoking base-class(Airplane) constructor
super(model, range);
seat = startHeight;
}
// the PassengerPlane subclass adds one more method
public void setHeight(int newValue)
{
seat = newValue;
}
// overriding toString() method
// of Airplane to print more info
@Override
public String toString()
{
return (super.toString()+
"\nNumber of seat is "+seat);
}
}
// driver class
public class Main
{
public static void main(String args[])
{
PassengerPlane obj = new PassengerPlane("Boeing 767", 450.90, 280);
System.out.println(obj.toString());
}
}
Step by step
Solved in 3 steps with 1 images

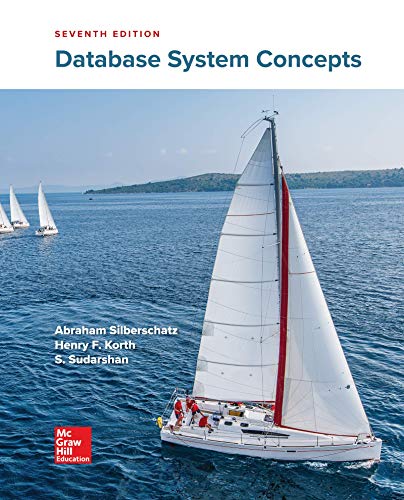
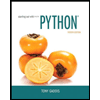
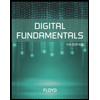
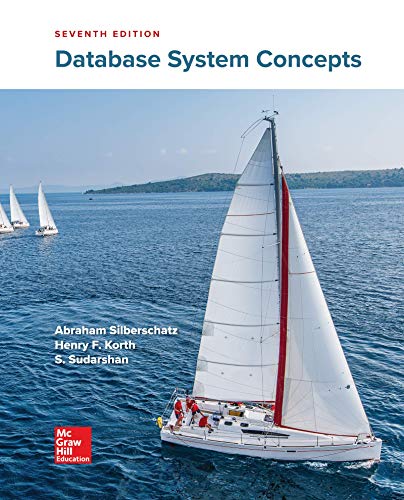
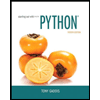
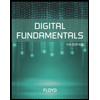
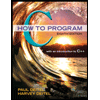
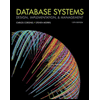
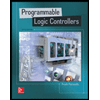