In this lab a grocery list editor with undo functionality is implemented. Step 1: Inspect the UndoCommand abstract base class The read-only UndoCommand.java file has a declaration for the UndoCommand abstract base class. Access UndoCommand.java by clicking on the orange arrow next to LabProgram.java at the top of the coding window. The UndoCommand class represents a command object: an object that stores all needed information to execute an action at a later point in time. For this lab, a command object stores information to undo a grocery list change made by the user. Step 2: Inspect the incomplete Grocery List class The GroceryList class is declared in Grocery List.java. Two protected fields are declared: • An ArrayList of strings for list items • A stack of UndoCommand references for undo commands Note that the addWithUndo() method is already implemented. The method adds a new item to the list and pushes a new RemoveLastCommand object onto the undo stack. Step 3: Implement RemoveLastCommand's execute() method The RemoveLastCommand class inherits from UndoCommand and is declared in RemoveLastCommand.java. When a RemoveLastCommand object is executed, the string ArrayList's last element is removed. So when the user appends a new item to the grocery list, a RemoveLastCommand is pushed onto the stack of undo commands. Popping and executing the RemoveLastCommand then removes the item most recently added by the user. RemoveLastCommand's sourceList field and constructor are already declared: ⚫ sourceList is a reference to a GroceryList object's ArrayList of strings. • The constructor takes a reference to an ArrayList of strings as a parameter, and assigns sourceList with the reference. Implement RemoveLastCommand's execute() method to remove sourceList's last element. Step 4: Implement Grocery List's execute Undo() method Implement Grocery List's executeUndo() method to do the following: • Pop an UndoCommand off the undo stack • Execute the popped undo command File LabProgram.java has code that reads in a list of commands, one per line, that allow for basic testing of basic operations. So after implementing executeUndo(), run your program with the following input: add bananas add grapes add strawberries print undo print undo print quit Verify that the corresponding output is: 0. bananas 1. grapes 2. strawberries 0. bananas 1. grapes 0. bananas The program's output does not affect grading, so additional test cases can be added, if desired. Submitting code written so far to obtain partial credit is recommended before proceeding to the next step. Step 5: Implement the Swap Command class and GroceryList's swap With Undo() method Implement the SwapCommand class in SwapCommand.java. The class itself is declared, but no class members yet exist. Add necessary fields and methods so that the command can undo swapping two items in the grocery list. Implement Grocery List's swap With Undo() method. The method swaps list items at the specified indices, then pushes a SwapCommand, to undo that swap, onto the undo stack. Step 6: Implement the InsertAtCommand class and Grocery List's removeAtWith Undo() method Implement the InsertAtCommand class in InsertAtCommand.java. Add necessary fields and methods so that the command can undo removing a grocery list item at an arbitrary index. Implement Grocery List's removeAtWith Undo() method. The method removes the list item at the specified index, then pushes an InsertAtCommand, to undo that removal, onto the undo stack.
I need help with this Java problem as it's explained in the images below:
(Don't edit)
import java.io.PrintWriter;
import java.util.Scanner;
import java.util.ArrayList;
import java.util.Arrays;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
GroceryList groceryList = new GroceryList();
PrintWriter testFeedback = new PrintWriter(System.out);
String command;
boolean quit = false;
while (!quit) {
command = scnr.nextLine();
// Process user input
if (command.equals("print")) {
groceryList.print(testFeedback);
} else if (0 == command.indexOf("add ")) {
groceryList.addWithUndo(command.substring(4));
} else if (0 == command.indexOf("removeat ")) {
int index = Integer.parseInt(command.substring(9));
groceryList.removeAtWithUndo(index);
} else if (0 == command.indexOf("swap ")) {
int index1 = -1, index2 = -1;
String str = command.substring(5);
if (str.indexOf(" ") != -1) {
index1 = Integer.parseInt(str.substring(0, 1));
index2 = Integer.parseInt(str.substring(2, 3));
groceryList.swapWithUndo(index1, index2);
} else {
System.out.print("\"swap\" command requires two indices, separated ");
System.out.println("by a space. Ex: swap 2 5");
}
} else if (command.equals("undo")) {
if (0 == groceryList.getUndoStackSize()) {
System.out.println("Cannot execute undo because undo stack is empty");
} else {
groceryList.executeUndo();
}
} else if (command.equals("quit")) {
quit = true;
} else {
System.out.println("Unknown command: " + command);
}
testFeedback.flush();
}
}
}
(Don't edit)
public abstract class UndoCommand {
public abstract void execute();
}
(need's editing)
import java.util.ArrayList;
public class RemoveLastCommand extends UndoCommand {
private ArrayList<String> sourceList;
public RemoveLastCommand(ArrayList<String> lst) {
this.sourceList = lst;
}
@Override
public void execute() {
// Your code here
}
}
(Need's editing)
import java.util.ArrayList;
public class SwapCommand extends UndoCommand {
// Your field declarations here
// Your constructor code here
@Override
public void execute() {
// Your code here
}
}
(Need's editing)
import java.util.ArrayList;
public class InsertAtCommand extends UndoCommand {
// Your field declarations here
// Your constructor code here
@Override
public void execute() {
// Your code here
}
}
(Need's editing)
import java.util.*;
import java.io.PrintWriter;
public class GroceryList {
protected ArrayList<String> listItems = new ArrayList<String>();
protected Stack<UndoCommand> undoStack = new Stack<UndoCommand>();
public void addWithUndo(String newItemName) {
// Add the new list item
listItems.add(newItemName);
// Make an undo command that removes the last item and push onto stack
undoStack.push(new RemoveLastCommand(listItems));
}
public void removeAtWithUndo(int removalIndex) {
// Your code here
}
public void swapWithUndo(int index1, int index2) {
// Your code here
}
// Pop and execute the command at the top of the stack
public void executeUndo() {
// Your code here
}
final int getListSize() {
return listItems.size();
}
final int getUndoStackSize() {
return undoStack.size();
}
final ArrayList<String> getVectorCopy() {
return listItems;
}
public void print(PrintWriter outputStream) {
for (int i = 0; i < listItems.size(); i++) {
outputStream.write(i + ". " + listItems.get(i) + "\n");
}
}
}



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

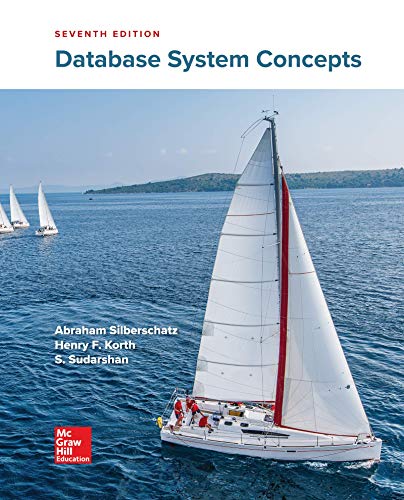
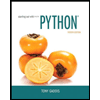
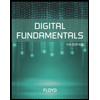
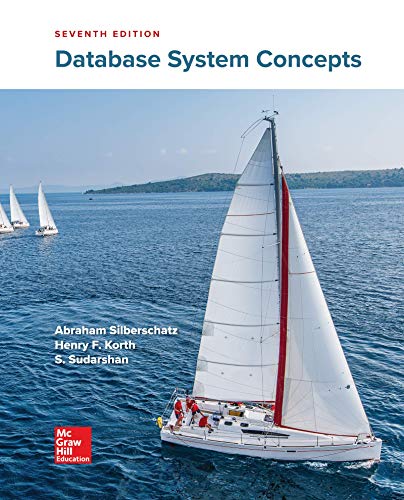
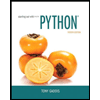
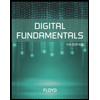
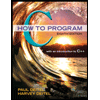
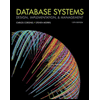
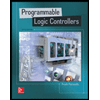