In this project you will implement a student database using JavaFX. A GUI should be used as the program's interface. This program must consist of two classes. (1) The first class should define the GUI and handle the database interactions: a. Combo box should allow the user to select one of the four database actions (Insert, Delete, Find, and Update). b. The database should be implemented as a HashMap, with the ID field as the key and a student record consisting of a name and major as the value. c. The operation should be performed when the user clicks the Process Request button. d. If the user attempts to insert a key that is already in the database an error message should be displayed using a JOptionPane message dialog box. e. If the user attempts to delete, find or update a record that is not in the database, a message should also be displayed. f. After each successful operation is completed a JOptionPane window should be displayed confirming the success. g. In the case of a successful Find request, a window should pop up containing the student's ID, name, major and current GPA. h. When the user selects the Update request, the following JOptionPane windows should be displayed to gather information about a course that has just been completed: (2) The second class named Student, should define the student record. It must have instancevariables for the student name, major and two variables that are used to compute the GPA. Avariable that contains the total number of credits completed and a second variable that containsthe total quality points, which are the numeric value of the grade received in a course times thenumber of credit hours. It should not contain the student ID. The class should have the followingthree methods: a. A constructor that is used when new student records are created. It should accept the name and major as parameters and initialize the fields that are used to compute the GPA to zero. b. The second method courseCompleted should accept the course grade and credit hours and update the variables used to compute the GPA. It will be called when an Update request is made. c. The third method should override toString and return a labeled string containing the student name, major and GPA. Finally, when a student has not yet completed any course, the GPA should be displayed as 4.0. Any exceptions thrown by nonnumeric inputs should be properly handled. • Include Header Comments that include your name, date, and description of the program. • Include Body Comments. Include a test table with columns indicating the test case #, input values, expected output, actual output, and if the test case passed or failed. This table should contain 4 columns with appropriate labels and a row for each test case. Be sure to select enough different scenarios to completely test the program, and provide screenshots for every test case.
In this project you will implement a student database using JavaFX. A GUI should be used as the program's interface. This program must consist of two classes. (1) The first class should define the GUI and handle the database interactions: a. Combo box should allow the user to select one of the four database actions (Insert, Delete, Find, and Update). b. The database should be implemented as a HashMap, with the ID field as the key and a student record consisting of a name and major as the value. c. The operation should be performed when the user clicks the Process Request button. d. If the user attempts to insert a key that is already in the database an error message should be displayed using a JOptionPane message dialog box. e. If the user attempts to delete, find or update a record that is not in the database, a message should also be displayed. f. After each successful operation is completed a JOptionPane window should be displayed confirming the success. g. In the case of a successful Find request, a window should pop up containing the student's ID, name, major and current GPA. h. When the user selects the Update request, the following JOptionPane windows should be displayed to gather information about a course that has just been completed: (2) The second class named Student, should define the student record. It must have instancevariables for the student name, major and two variables that are used to compute the GPA. Avariable that contains the total number of credits completed and a second variable that containsthe total quality points, which are the numeric value of the grade received in a course times thenumber of credit hours. It should not contain the student ID. The class should have the followingthree methods: a. A constructor that is used when new student records are created. It should accept the name and major as parameters and initialize the fields that are used to compute the GPA to zero. b. The second method courseCompleted should accept the course grade and credit hours and update the variables used to compute the GPA. It will be called when an Update request is made. c. The third method should override toString and return a labeled string containing the student name, major and GPA. Finally, when a student has not yet completed any course, the GPA should be displayed as 4.0. Any exceptions thrown by nonnumeric inputs should be properly handled. • Include Header Comments that include your name, date, and description of the program. • Include Body Comments. Include a test table with columns indicating the test case #, input values, expected output, actual output, and if the test case passed or failed. This table should contain 4 columns with appropriate labels and a row for each test case. Be sure to select enough different scenarios to completely test the program, and provide screenshots for every test case.
Chapter8: Arrays
Section: Chapter Questions
Problem 7PE
Related questions
Question
In this project you will implement a student
This program must consist of two classes.
(1) The first class should define the GUI and handle the database interactions:
a. Combo box should allow the user to select one of the four database actions (Insert,
Delete, Find, and Update).
b. The database should be implemented as a HashMap, with the ID field as the key and a
student record consisting of a name and major as the value.
c. The operation should be performed when the user clicks the Process Request button.
d. If the user attempts to insert a key that is already in the database an error message
should be displayed using a JOptionPane message dialog box.
e. If the user attempts to delete, find or update a record that is not in the database, a
message should also be displayed.
f. After each successful operation is completed a JOptionPane window should be
displayed confirming the success.
g. In the case of a successful Find request, a window should pop up containing the student's
ID, name, major and current GPA.
h. When the user selects the Update request, the following JOptionPane windows should be
displayed to gather information about a course that has just been completed:
a. Combo box should allow the user to select one of the four database actions (Insert,
Delete, Find, and Update).
b. The database should be implemented as a HashMap, with the ID field as the key and a
student record consisting of a name and major as the value.
c. The operation should be performed when the user clicks the Process Request button.
d. If the user attempts to insert a key that is already in the database an error message
should be displayed using a JOptionPane message dialog box.
e. If the user attempts to delete, find or update a record that is not in the database, a
message should also be displayed.
f. After each successful operation is completed a JOptionPane window should be
displayed confirming the success.
g. In the case of a successful Find request, a window should pop up containing the student's
ID, name, major and current GPA.
h. When the user selects the Update request, the following JOptionPane windows should be
displayed to gather information about a course that has just been completed:
(2) The second class named Student, should define the student record. It must have instance
variables for the student name, major and two variables that are used to compute the GPA. A
variable that contains the total number of credits completed and a second variable that contains
the total quality points, which are the numeric value of the grade received in a course times the
number of credit hours. It should not contain the student ID. The class should have the following
three methods:
variables for the student name, major and two variables that are used to compute the GPA. A
variable that contains the total number of credits completed and a second variable that contains
the total quality points, which are the numeric value of the grade received in a course times the
number of credit hours. It should not contain the student ID. The class should have the following
three methods:
a. A constructor that is used when new student records are created. It should accept the
name and major as parameters and initialize the fields that are used to compute the GPA
to zero.
b. The second method courseCompleted should accept the course grade and credit hours
and update the variables used to compute the GPA. It will be called when an Update
request is made.
c. The third method should override toString and return a labeled string containing the
student name, major and GPA.
name and major as parameters and initialize the fields that are used to compute the GPA
to zero.
b. The second method courseCompleted should accept the course grade and credit hours
and update the variables used to compute the GPA. It will be called when an Update
request is made.
c. The third method should override toString and return a labeled string containing the
student name, major and GPA.
Finally, when a student has not yet completed any course, the GPA should be displayed as 4.0.
Any exceptions thrown by nonnumeric inputs should be properly handled.
• Include Header Comments that include your name, date, and description of the program.
• Include Body Comments.
Include a test table with columns indicating the test case #, input values, expected output, actual output, and if the test case passed or failed. This table should contain 4 columns with appropriate labels and a row for each test case. Be sure to select enough different scenarios to completely test the program, and provide screenshots for every test case.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
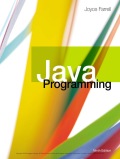
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
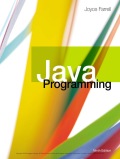
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT