The two classes you will create will implement the operations defined in the interface as shown in the UML class diagram above. In addition, BinarySearchArray will implement a static method testBinarySearchArray() that populates the lists, and lets the user interactively test the two classes by adding and removing elements. The parameter BinarySearch can represent either class and tells testBinarySearchArray which class to test. Steps to Implement: 1) To get started create a new project in IntelliJ called BinarySearch. Add a class to your project called BinarySearchArray. Then add another class BinarySearchArrayList and an interface called BinarySearch. The interface BinarySearch includes public method stubs as shown in the diagram. You are allowed to add BinarySearchArrayList to the same file as BinarySearch but don't add an access modifier to this class, or for easier reading, you can declare the classes in separate files with public access modifiers. Only the class containing the main method can be defined as public in files defining multiple classes. You should read up on interfaces if you are unfamiliar. An interface provides a template for defined behavior to a class that inherits from the interface. The implementation is left to the implementing class. A class that implements an interface must implement ALL the methods declared in the interface, unless the class inherits from another class that already implements one or more of those methods. This is convenient because different classes that implement the same interface solve a similar problem but in a different way. The interface is a promise of certain general behavior, and the concrete class provides the concrete specialized implementation. A GrazingMammal nurses (an abstraction or interface). A Cow nurses(a concrete object). 2) The two classes you will define are as follows: public class BinarySearchArray implements BinarySearch { } class BinarySearchArrayList implements BinarySearch{ } Since you are implementing the interface, as mentioned you must provide implementations of the interface methods exactly as defined in the interface. The interface has only the declarations. These methods will have the same signature as the interface but may be implemented very differently, as is the case in this assignment. This is logical since one class operates on arrays, the other on ArrayLists. Implementation Details (See the BinarySearch interface above - method name correspond to interface and class definitions)
The two classes you will create will implement the operations defined in the interface as shown in the UML class diagram above. In addition, BinarySearchArray will implement a static method testBinarySearchArray() that populates the lists, and lets the user interactively test the two classes by adding and removing elements. The parameter BinarySearch can represent either class and tells testBinarySearchArray which class to test. Steps to Implement: 1) To get started create a new project in IntelliJ called BinarySearch. Add a class to your project called BinarySearchArray. Then add another class BinarySearchArrayList and an interface called BinarySearch. The interface BinarySearch includes public method stubs as shown in the diagram. You are allowed to add BinarySearchArrayList to the same file as BinarySearch but don't add an access modifier to this class, or for easier reading, you can declare the classes in separate files with public access modifiers. Only the class containing the main method can be defined as public in files defining multiple classes. You should read up on interfaces if you are unfamiliar. An interface provides a template for defined behavior to a class that inherits from the interface. The implementation is left to the implementing class. A class that implements an interface must implement ALL the methods declared in the interface, unless the class inherits from another class that already implements one or more of those methods. This is convenient because different classes that implement the same interface solve a similar problem but in a different way. The interface is a promise of certain general behavior, and the concrete class provides the concrete specialized implementation. A GrazingMammal nurses (an abstraction or interface). A Cow nurses(a concrete object). 2) The two classes you will define are as follows: public class BinarySearchArray implements BinarySearch { } class BinarySearchArrayList implements BinarySearch{ } Since you are implementing the interface, as mentioned you must provide implementations of the interface methods exactly as defined in the interface. The interface has only the declarations. These methods will have the same signature as the interface but may be implemented very differently, as is the case in this assignment. This is logical since one class operates on arrays, the other on ArrayLists. Implementation Details (See the BinarySearch interface above - method name correspond to interface and class definitions)
Chapter10: Application Development
Section: Chapter Questions
Problem 9VE
Related questions
Question
100%
The two classes you will create will implement the operations defined in the interface as shown in the UML class diagram above.
In addition, BinarySearchArray will implement a static method testBinarySearchArray() that populates the lists, and lets the user interactively test the two classes by adding and removing elements. The parameter BinarySearch can represent either class and tells testBinarySearchArray which class to test.
Steps to Implement:
1) To get started create a new project in IntelliJ called BinarySearch. Add a class to your project called BinarySearchArray. Then add another class BinarySearchArrayList and an interface called BinarySearch. The interface BinarySearch includes public method stubs as shown in the diagram. You are allowed to add BinarySearchArrayList to the same file as BinarySearch but don't add an access modifier to this class, or for easier reading, you can declare the classes in separate files with public access modifiers. Only the class containing the main method can be defined as public in files defining multiple classes.
You should read up on interfaces if you are unfamiliar. An interface provides a template for defined behavior to a class that inherits from the interface. The implementation is left to the implementing class. A class that implements an interface must implement ALL the methods declared in the interface, unless the class inherits from another class that already implements one or more of those methods.
This is convenient because different classes that implement the same interface solve a similar problem but in a different way. The interface is a promise of certain general behavior, and the concrete class provides the concrete specialized implementation. A GrazingMammal nurses (an abstraction or interface). A Cow nurses(a concrete object).
2) The two classes you will define are as follows:
public class BinarySearchArray implements BinarySearch {
}
class BinarySearchArrayList implements BinarySearch{
}
Since you are implementing the interface, as mentioned you must provide implementations of the interface methods exactly as defined in the interface. The interface has only the declarations. These methods will have the same signature as the interface but may be implemented very differently, as is the case in this assignment. This is logical since one class operates on arrays, the other on ArrayLists.
Implementation Details (See the BinarySearch interface above - method name correspond to interface and class definitions)

Transcribed Image Text:IntelliJ IDEA
File
Edit
View Navigate
Code
Analyze
Refactor
Build
Run
Tools
Vcs
Window
Help
7 ? 5%
Sun 9:26 AM
A delaGATE - Delawar x
O Project 4: Binary Se x
b Answered: Create
O (2) Navigation in Int x
G toyota yaris 2021 -
Iccc.edu/courses/27620/assignments/513564
Use binarySearch or
op
Average of 3 #
GEScreen Shotals
binarySearch return a
negative number if not
present, otherwise returns
a for loop to
2021-04a5.62 AM
arrlist.contains(Integer.valu
e0f(value))
boolean
boolean contains(value)
determine if key is
present in the array.
nd
contains(value)
NG ima
index.
NG ima
No api method.
NG imprld
C++ 1
An "empty" element is
NG ima
NG ima
NG ima
NG ima
marked by a zero value. If
No api method
arrlist.add(value);
add(int value)
no zeros, print an error
add(int value)
available
message "no space
available"
NG ima
NG im input
Cat's age
Area of Square
ers
NG ima
initializeArray
NG ima
initializeArray()
Same as array class.
NG ima
NG ima
Prints the elements of the
NG ima
Prints the elements of the array
f Two
Decimal pointer
NG ima
array
array on a single line
delimited by spaces and
er
No api method
on a single line delimited by
NG ima
printElementsO
printElements()
NG ima
spaces and newline at end of
line. Uses arrList.
available
newline at end of line.
NG ima
NG ima
Uses arr.
ky Number
Differences
asignment
public static v
oid testBinarys
earchArray(Bina
Test driver method to be
called from main. Takes
rySearch search
Object)
This method is
part of Binarys implement.
See the discussion
parameter of BinarySearch
which indicates the method
Use static method
BinarySearchArray.
testBinarySearchArray(bsArrList)
below on how to
Same as array version
ScreenShot
0...13.34 PM
FirstProject
asignment 1
earchArray clas
s. Use bsArr fo
r parameter
which class to test.

Transcribed Image Text:IntelliJ IDEA
File
Edit
View Navigate
Code
Analyze
Refactor
Build
Tools
Window
7 ? 5%
Sun 9:25 AM
Run
Vcs
Help
S delaGATE - Delawar X
O Project 4: Binary Se x
b Answered: Create
O (2) Navigation in Int x G toyota yaris 2021 -
Iccc.edu/courses/27620/assignments/513564
This is convenient because different classes that implement the same
op
Average of 3 #
GEScreen Shotals
interface solve a similar problem but in a different way. The interface is a
2021-04a:5.44 AM
promise of certain general behavior, and the concrete class provides the
nd
concrete specialized implementation. A GrazingMammal nurses (an
NG ima
NG ima
abstraction or interface). A Cow nurses(a concrete object).
NG imprld
C++ 1
2) The two classes you will define are as follows:
NG ima
NG ima
NG ima
public class BinarySearchArray implements BinarySearch {
NG ima
class BinarySearchArrayList implements BinarySearch{
}
NG ima
NG im input
Cat's age
Area of Square
ers
NG ima
NG ima
NG ima
NG ima
Since you are implementing the interface, as mentioned you must
provide implementations of the interface methods exactly as defined in
the interface. The interface has only the declarations. These methods
NG ima
f Two
Decimal pointer
array
NG ima
er
NG ima
will have the same signature as the interface but may be implemented
very differently, as is the case in this assignment. This is logical since
NG ima
one class operates on arrays, the other on ArrayLists.
NG ima
Implementation Details (See the BinarySearch interface above - method
name correspond to interface and class definitions)
NG ima
ky Number
Differences
asignment
Arrays - How to
Method Name
Comments
ArrayList
Comments
implement
sort
Arrays.sort()
import java.util.Arrays
Collections.sort
import java.util.Collections
Arrays.binarySearch(arr,key).
Returns a negative number
ScreenShot
0...13.34 PM
FirstProject
asignment 1
Collections.binarySearch(arrList,
binarySearch
Arrays.binarySearch()
Collections.binarySearch()
key)
if not present, otherwise
return index.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
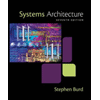
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
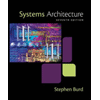
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning