understanding
Your understanding of importing modules, using lists and dictionaries and creating objects from a class will be exercised in this lab.
Be prepared to use your solution for the “Classes” lab (see Blackboard for the link) where you wrote the PlayingCard class.
In this lab, you will have to generate a random list of PlayingCard objects for where the suit and rank combinations of each card are randomly choosen. Using this list of randomly created playing cards, you will use dictionaries and lists to store important data about these playing cards, particularly the frequency distribution of each rank-suit combination you created (e.g. like if 10 queen of hearts cards are generated and counted.)
Thanks to the clearly described interface used to communicate to any PlayingCard object, specifically the get_rank() and get_suit() methods, you can just import your “Classes” lab as a module and use your previously written code!
Note: You’ll definitely need to make sure your solutions work for this lab, and if not, please consult the professor or TAs for assistance, ASAP.
-
I provide a starter (skeleton) code file for your solution. You are required to use it. Download lab_data_collections.py and complete it with your code.
-
Your lab assignment requires the use of your solution from the Classes lab. You will import your code as a module and use your PlayingCard class.
The skeleton code includes the necessary import line, just provide your file by placing a copy of your lab_classes.py file in the same directory as your solution code for this lab.
-
The docstrings inside this file describe what each function should do.
-
Replace and complete missing code wherever you see::
## REPLACE WITH YOUR CODE.#Do not change anything else like function names, parameters or return values. Do not remove the if statements at the start of some functions as they are needed to make sure you are passing the correct values. You will lose points you if you change anything you are not supposed to change.
Output
This is what you should see (your numbers will be different):
Note: All printed strings must match the above output. To otherwise deviate will cause the Gradescope autograder to deduct points.
Skeleton Code
from random import randrange
from lab_classes import PlayingCard
from math import sqrt
suit_size = 13 # Number of cards in a suit.
deck_size = 52 # Number of cards in a deck.
num_cards = 260 # Number of cards to create with random rank & suit values
def make_random_cards():
"""Generate num_cards number of random PlayingCard objects.
This function will generate num_cards RANDOM playing cards
using your PlayingCard class. That means you will have to choose a random
suit and rank for a card num_cards times.
Printing:
Nothing
Positional arguments:
None
Returns:
cards_list -- a list of PlayingCard objects.
"""
#
# REPLACE WITH YOUR CODE.
#
return cards_list
def freq_count(cards_list):
"""Count every suit-rank combination.
Returns a dictionary whose keys are the card suit-rank and value is the
count.
Printing:
Nothing
Positional arguments:
cards_list -- A list of PlayingCard objects.
Returns:
card_freqs -- A dictionary whose keys are the single letter in the set
'd', 'c', 'h', 's' representing each card suit. The value for each key
is a list containing the number of cards at each rank, using the index
position to represent the rank. For example, {'s': [0, 3, 4, 2, 5]}
says that the key 's', for 'spades' has three rank 1's (aces), four
rank 2's (twos), two rank 3's (threes) and 5 rank 4's (fours). Index
position 0 is 0 since no cards have a rank 0, so make note.
"""
# DO NOT REMOVE BELOW
if type(cards_list) != list or \
list(filter(lambda x: type(x) != PlayingCard, cards_list)):
raise TypeError("cards_list is required to be a list of PlayingCard \
objects.")
# DO NOT REMOVE ABOVE
#
# REPLACE WITH YOUR CODE.
#
return card_freqs
def std_dev(counts):
"""Calculate the standard deviation of a list of numbers.
Positional arguments:
counts -- A list of ints representing frequency counts.
Printing:
Nothing
Returns:
_stdev -- The standard deviation as a single float value.
"""
# DO NOT REMOVE BELOW
if type(counts) != list or \
list(filter(lambda x: type(x) != int, counts)):
raise TypeError("counts is required to be a list of int values.")
# DO NOT REMOVE ABOVE
(The image attached has the continued part of the code)
![def print_stats (card_freqs):
"""Print the final stats of the PlayingCard objects.
Positional arguments:
card freqs -- A dictionary whose keys are the single letter in the set
'dchs' representing each card suit. The value for each key is a list of
numbers where each index position is a card rank, and its value is its
card frequency.
You will probably want to call th std dev function in somewhere in
here.
Printing:
Prints the statistic for each suit to the screen,
for an example output.
see assignment page
Returns:
None
II II II
# DO NOT REMOVE BELOW
if type (card_freqs) != dict or \
list (filter (lambda x: type (card_freqs [x]) != list, card_freqs)):
raise TypeError("card_freqs is required to be a dict where each value is a list of ints.")
# DO NOT REMOVE ABOVE
# REPLACE WITH YOUR CODE.
def main ():
cards = make random cards ()
suit_counts = freq_count (cards)
print_stats (suit_counts)
if
name
main
":
==
main()](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe8f6e8df-fe29-4e31-a491-f3152d4002a8%2F8141917c-ce44-4a4e-b58e-0e4f3f429324%2F4et4arf_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

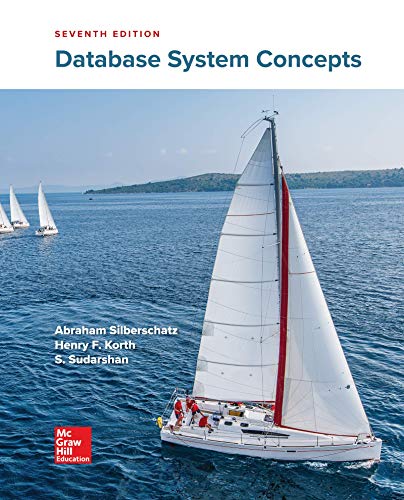
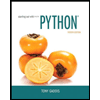
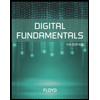
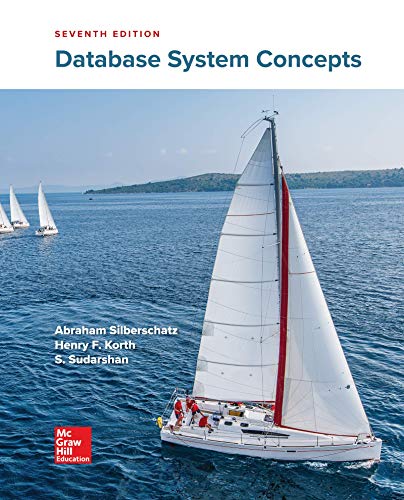
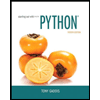
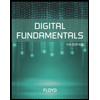
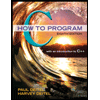
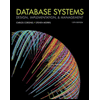
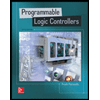