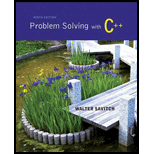
Write a
bool isLeapYear(int year);
This function should return true if year is a leap year and false if it is not. Here is pseudocode to determine a leap year:
leapYear = (year divisible by 400) or (year divisible by 4 and year not divisible by 100))
int getCenturyValue(int year);
This function should take the first two digits of the year (that is. the century), divide by 4. and save the remainder. Subtract the remainder from 3 and return this value multiplied by 2. For example, the year 2008 becomes: (20/4) = 5 with a remainder of 0. 3 - 0 = 3. Return 3*2 = 6.
int getYearValue(int year);
This function computes a value based on the years since the beginning of the century. First, extract the last two digits of the year. For example, 08 is extracted for 2008. Next, factor in leap years. Divide the value from the previous Step by 4 and discard the remainder. Add the two results together and return this value. For example, from 2008 we extract 08. Then (8/4) = 2 with a remainder of 0. Return 2 + 8=10.
int getMonthVa0lue(int month, int year);
This function should return a value based on the table below and will require invoking the isLeapYear function.
Month | Return Value |
January | 0 (6 if year is a leap year) |
February | 3 (2 if year is a leap year) |
March | 3 |
April | 6 |
May | 1 |
June | 4 |
July | 6 |
August | 2 |
September | 5 |
October | 0 |
November | 3 |
December | 5 |
Finally, to compute the day of the week, compute the sum of the date’s day plus the values returned by getMonthValue, getYearValue, and getCenturyValue. Divide the sum by 7 and compute the remainder. A remainder of 0 corresponds to Sunday, 1 corresponds to Monday, etc., up to 6, which corresponds to Saturday. For example, the date July 4, 2008 should be computed as (day of month) 1 (getMonthValue) 1 (getYearValue) 1 (getCenturyValue) = 4 + 6 + 10 + 6 = 26. 26/7 = 3 with a remainder of 5.
The fifth day of the week corresponds to Friday.
Your program should allow the user to enter any date and output the corresponding day of the week in English.
This program should include a void function named getInput that prompts the user for the date and returns the month, day, and year using pass-by-reference Parameters. You may choose to have the user enter the date’s month as either a number (1–12) or a month name.

Want to see the full answer?
Check out a sample textbook solution
Chapter 5 Solutions
Problem Solving with C++ (9th Edition)
Additional Engineering Textbook Solutions
Starting Out with C++: Early Objects
Starting Out with Java: From Control Structures through Objects (6th Edition)
Software Engineering (10th Edition)
Experiencing MIS
Introduction To Programming Using Visual Basic (11th Edition)
Concepts of Programming Languages (11th Edition)
- A prime number is an integer greater than 1 that is evenly divisible by only 1 and itself. For example, the number 5 is prime because it can only be evenly divided by 1 and 5. The number 6, however, is not prime because it can be divided by 1, 2, 3, and 6. Write a Boolean function named isPrime, which takes an integer as an argument and returns true if the argument is a prime number, and false otherwise. Demonstrate the function in a complete program.arrow_forwardWrite a DECIMATOR function that takes a unit of character and minimizes it (i.e. removes the last 1/10 of characters). Always zoom in: if a bullet unit has 21 characters, 1/10 of the characters will be 2.1 characters, so DECIMATOR removes 3 characters. DECIMATOR is not kind! Examples decimator ("1234567890") 12 "123456789" # 10 characters, taken out 1. decimator ("1234567890AB") # 12 characters, 2 are released. "1234567890" decimator ("123") "12" # 3 characters, taken out 1. decimator ("123456789012345678901") # 21 characters, issued 3. Solve in Ruby "123456789012345678"arrow_forwardfor python program 1. A prime number is a number that is only evenly divisible by itself and 1. For example, the number 5 is prime because it can only be evenly divided by 1 and 5. The number 6, however, is not prime because it can be divided evenly by 1, 2, 3, and 6.Write a Boolean function named is_prime which takes an integer as an argument and returns true if the argument is a prime number, or false otherwise. Use the function in a program that prompts the user to enter a number then displays a message indicating whether the number is prime.Tip:Recall that the % operator divides one number by another and returns the remainder of the division. In an expression such as num1 % num2, the % operator will return 0 if num1 is evenly divisible by num2arrow_forward
- Going missing Some calculations involving blank values may give different results to what you might expect. For example, when you pass a blank value into the AND() function, it is treated as TRUE. This is often unhelpful. To make blanks behave in a sensible way in calculations, you must first convert them to be "not available" using NA(). This function takes no inputs, and returns a missing value. To convert a blank value to a missing value, use this pattern. =IF(ISBLANK(cell), NA(), cell) Instruction In column H, use AND() to find women who have kids and get benefits. In column I, convert the blanks in column G to missing values. In column J, again find women who have kids and get benefits, this time using column I rather than G. please show the formula used to "has kids and gets benefits" , "gets benefits with missing values", has kids and gets benefits". Thank you Is non-white Has over 12 years of school? Is married? Has kids? Has young kids? Is head of household? Gets…arrow_forwardRolling a single six-sided die produces a random number between 1 and 6 with a uniform distribution. Rolling two dice produces a number between 2 and 12 with a binomial distribution. In this lab you will simulate a number of dice rolls and graph the number of occurrences of each value. 1) Create a function called rollDie() that takes no parameters, and returns a random value between 1 and 6. Do not seed the random number generator in this function. int rollDie() 2) Create a function called initArray() that takes a data array and the size of the array as parameters and fills each element with the summed results of rolling two dice. Do not assume that the data array has been initialized. void initArray(int data[], int size) 3) Create a function called sortArray() that takes an array and the size of the array as parameters, and sorts the array using the sediment sort. void sortArray(int data[], int size) The sediment sort works as follows: start with the second element in the array if…arrow_forwardWrite a python function that takes a positive integer n as an argument. This function will return the number of positive integers less than n that are co-prime to n. Co-prime Numbers: Two numbers are coprime to each other if they don't have any common divisor except 1. Sample Input 1: 1 Sample Output 1: Reason: Since 1 is the smallest positive integer, there exists no positive integer that is less than and coprime to 1. Sample Input 2: 2 Sample Output 2: 1 Reason: Only 1 doesn't share any common divisor (except 1 itself) with 2. Also, 1 < 2. Sample Input 3: Sample Output 3: Reason: Namely, the two coprime numbers to 6 are 1 and 5. Sample Input 4: 36 Sample Output 4: 12 Reason: The numbers coprime to 36 are 1, 5, 7, 11, 13, 17, 19, 23, 25, 29, 31 and 35arrow_forward
- .Prime NumbersIn this task, you will implement a program that takes as input a number n and produces alist of integers less than or equal to n that are prime. The way to think about a problem isto break it down into individual parts. Start by creating a function isPrime() that checkswhether it’s argument is prime or not. The pseudocode for checking isPrime is givenbelow, there are better ways to do it… this is just one.6Step 1: count = 2Step 2: while count < numberStep 3: if count divides the number evenly return falseStep 4: add 1 to countStep 5: Goto step 2Step 6: return truearrow_forwardWrite a program in python with a function repeatPhrase(phrase, n), which prints the given phrase n times, alternating between lowercase and uppercase. For example, repeat('The sky is yellow', 5) would print: the sky is yellow THE SKY IS YELLOW the sky is yellow THE SKY IS YELLOW the sky is yellow Use the function in a program where you ask the user for the phrase and the value of narrow_forwardCode should be in Python, please Write a program that takes in a positive integer as input, and outputs a string of 1's and 0's representing the integer in binary. For an integer x, the algorithm is: As long as x is greater than 0 Output x % 2 (remainder is either 0 or 1) x = x // 2 Note: The above algorithm outputs the 0's and 1's in reverse order. You will need to write a second function to reverse the string. Ex: If the input is: 6 the output is: 110 Your program must define and call the following two functions. The function integer_to_reverse_binary() should return a string of 1's and 0's representing the integer in binary (in reverse). The function reverse_string() should return a string representing the input string in reverse.def integer_to_reverse_binary(integer_value)def reverse_string(input_string)arrow_forward
- Write a program that will:• Select a random number between 1 and 100.• Test if the number is prime• Call a function named isPrime(). Pass the number to be tested. isPrime() should test whetherthe number has any factors other than 1 and itself. isPrime() returns either True or False.• isPrime() should use a function isDivisible(x,y) to test if y divides evenly into x. That is,isDivisible() will identify whether y is a factor of x.• Print the resultarrow_forwardA natural number is prime if it is greater than 1 and has no divisors other than 1 and itself. Example: 8 isn't a prime number, as you can divide it by 2 and 4 (we can't use divisors equal to 1 and 8 as the definition prohibits this). On the other hand, 7 is a prime number as we can't find any legal divisors for it. Your task is to write a function checking whether a number is prime or not. in phython langauge Ques: The function: is called IsPrime() takes one argument (the value to check) returns True if the argument is a prime number, and False otherwise. Hint: try to divide the argument by all subsequent values (starting from 2) and check the remainder - if it's zero, your number cannot be a prime; think carefully about when you should stop the process. If you need to know the square root of any value you can utilize the ** operator. Remember: the square root of x is the same as x**0.5 Write a code that calculates all the prime numbers between 1 and 20. (Hint: Use a loop and…arrow_forwardA natural number is prime if it is greater than 1 and has no divisors other than 1 and itself.Example: 8 isn't a prime number, as you can divide it by 2 and 4 (we can't use divisors equal to 1 and 8 as the definition prohibits this). On the other hand, 7 is a prime number as we can't find any legal divisors for it.Your task is to write a function checking whether a number is prime or not.The function:•is called IsPrime()•takes one argument (the value to check)•returns True if the argument is a prime number, and False otherwise.Hint: try to divide the argument by all subsequent values (starting from 2) and check the remainder - if it's zero, your number cannot be a prime; think carefully about when you should stop the process.If you need to know the square root of any value you can utilize the ** operator. Remember: the square root of x is the same as x**0.5Python programming question Write a code that calculates all the prime numbers between 1 and 20. (Hint: Use a loop and call the…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
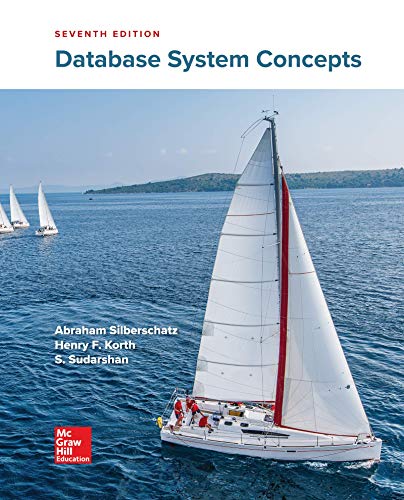
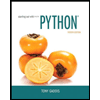
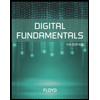
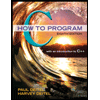
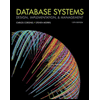
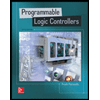